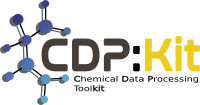 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_UTIL_FILEDATAWRITER_HPP
30 #define CDPL_UTIL_FILEDATAWRITER_HPP
49 template <
typename WriterImpl,
typename DataType =
typename WriterImpl::DataType>
55 std::ios_base::openmode mode = std::ios_base::in | std::ios_base::out | std::ios_base::trunc | std::ios_base::binary);
61 operator const void*()
const;
76 template <
typename WriterImpl,
typename DataType>
78 stream(file_name.c_str(), mode), fileName(file_name), writer(stream)
80 writer.setParent(
this);
84 template <
typename WriterImpl,
typename DataType>
91 }
catch (
const std::exception& e) {
92 throw Base::IOError(
"FileDataWriter: while writing file '" + fileName +
"': " + e.what());
98 template <
typename WriterImpl,
typename DataType>
105 template <
typename WriterImpl,
typename DataType>
108 return writer.operator
const void*();
111 template <
typename WriterImpl,
typename DataType>
114 return writer.operator!();
117 #endif // CDPL_UTIL_FILEDATAWRITER_HPP
void close()
Writes format dependent data (if required) to mark the end of output.
Definition: FileDataWriter.hpp:99
Definition of the class CDPL::Base::DataWriter.
Thrown to indicate that an I/O operation has failed because of physical (e.g. broken pipe) or logical...
Definition: Base/Exceptions.hpp:250
FileDataWriter.
Definition: FileDataWriter.hpp:51
void invokeIOCallbacks(double progress) const
Invokes all registered I/O callback functions with the argument *this.
FileDataWriter(const std::string &file_name, std::ios_base::openmode mode=std::ios_base::in|std::ios_base::out|std::ios_base::trunc|std::ios_base::binary)
Definition: FileDataWriter.hpp:77
bool operator!() const
Definition: FileDataWriter.hpp:112
typename WriterImpl::DataType DataType
The type of the written data objects.
Definition: DataWriter.hpp:74
Definition of exception classes.
The namespace of the Chemical Data Processing Library.
An interface for writing data objects of a given type to an arbitrary data sink.
Definition: DataWriter.hpp:63
FileDataWriter & write(const DataType &obj)
Definition: FileDataWriter.hpp:86