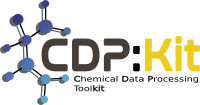 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_UTIL_FILEDATAREADER_HPP
30 #define CDPL_UTIL_FILEDATAREADER_HPP
49 template <
typename ReaderImpl,
typename DataType =
typename ReaderImpl::DataType>
55 std::ios_base::openmode mode = std::ios_base::in | std::ios_base::binary);
71 operator const void*()
const;
87 template <
typename ReaderImpl,
typename DataType>
89 stream(file_name.c_str(), mode), fileName(file_name), reader(stream)
91 reader.setParent(
this);
95 template <
typename ReaderImpl,
typename DataType>
100 reader.
read(obj, overwrite);
102 }
catch (
const std::exception& e) {
103 throw Base::IOError(
"FileDataReader: while reading file '" + fileName +
"': " + e.what());
109 template <
typename ReaderImpl,
typename DataType>
114 reader.
read(idx, obj, overwrite);
116 }
catch (
const std::exception& e) {
117 throw Base::IOError(
"FileDataReader: while reading file '" + fileName +
"': " + e.what());
123 template <
typename ReaderImpl,
typename DataType>
130 }
catch (
const std::exception& e) {
131 throw Base::IOError(
"FileDataReader: while reading file '" + fileName +
"': " + e.what());
137 template <
typename ReaderImpl,
typename DataType>
140 return reader.hasMoreData();
143 template <
typename ReaderImpl,
typename DataType>
146 return reader.getRecordIndex();
149 template <
typename ReaderImpl,
typename DataType>
152 reader.setRecordIndex(idx);
155 template <
typename ReaderImpl,
typename DataType>
158 return reader.getNumRecords();
161 template <
typename ReaderImpl,
typename DataType>
164 return reader.operator
const void*();
167 template <
typename ReaderImpl,
typename DataType>
170 return reader.operator!();
173 template <
typename ReaderImpl,
typename DataType>
180 #endif // CDPL_UTIL_FILEDATAREADER_HPP
FileDataReader(const std::string &file_name, std::ios_base::openmode mode=std::ios_base::in|std::ios_base::binary)
Definition: FileDataReader.hpp:88
bool operator!() const
Definition: FileDataReader.hpp:168
bool hasMoreData()
Tells if there are any data records left to read.
Definition: FileDataReader.hpp:138
typename ReaderImpl::DataType DataType
The type of the read data objects.
Definition: DataReader.hpp:79
Definition of the class CDPL::Base::DataReader.
Thrown to indicate that an I/O operation has failed because of physical (e.g. broken pipe) or logical...
Definition: Base/Exceptions.hpp:250
void invokeIOCallbacks(double progress) const
Invokes all registered I/O callback functions with the argument *this.
FileDataReader & skip()
Skips the data record at the current record index.
Definition: FileDataReader.hpp:125
An interface for reading data objects of a given type from an arbitrary data source.
Definition: DataReader.hpp:73
void setRecordIndex(std::size_t idx)
Sets the index of the current data record to idx.
Definition: FileDataReader.hpp:150
void close()
Performs a reader specific shutdown operation (if required).
Definition: FileDataReader.hpp:174
Definition of exception classes.
std::size_t getRecordIndex() const
Definition: FileDataReader.hpp:144
FileDataReader.
Definition: FileDataReader.hpp:51
The namespace of the Chemical Data Processing Library.
FileDataReader & read(DataType &obj, bool overwrite=true)
Definition: FileDataReader.hpp:97
std::size_t getNumRecords()
Returns the total number of available data records.
Definition: FileDataReader.hpp:156