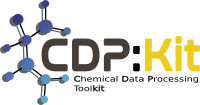 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
27 #ifndef CDPL_MATH_EXPRESSION_HPP
28 #define CDPL_MATH_EXPRESSION_HPP
118 template <
typename E>
140 template <
typename C>
162 template <
typename C>
184 template <
typename C>
206 template <
typename C>
230 #endif // CDPL_MATH_EXPRESSION_HPP
E ExpressionType
Definition: Expression.hpp:79
const unsigned int E
Specifies that the stereocenter has E configuration.
Definition: CIPDescriptor.hpp:96
ContainerType & operator()()
Definition: Expression.hpp:174
~GridExpression()
Definition: Expression.hpp:137
Definition: Quaternion.hpp:483
Definition: Matrix.hpp:212
C ContainerType
Definition: Expression.hpp:189
const ExpressionType & operator()() const
Definition: Expression.hpp:125
~MatrixContainer()
Definition: Expression.hpp:181
Definition: Expression.hpp:54
MatrixExpression()
Definition: Expression.hpp:92
Definition: Vector.hpp:507
QuaternionContainer()
Definition: Expression.hpp:202
const ContainerType & operator()() const
Definition: Expression.hpp:147
ContainerType & operator()()
Definition: Expression.hpp:196
~VectorContainer()
Definition: Expression.hpp:159
Definition: Expression.hpp:76
Definition: Expression.hpp:186
const ExpressionType & operator()() const
Definition: Expression.hpp:59
Definition: Expression.hpp:98
C ContainerType
Definition: Expression.hpp:211
ExpressionType & operator()()
Definition: Expression.hpp:86
Expression()
Definition: Expression.hpp:45
const ExpressionType & operator()() const
Definition: Expression.hpp:81
~Expression()
Definition: Expression.hpp:46
GridContainer()
Definition: Expression.hpp:224
Definition: Math/Grid.hpp:605
~MatrixExpression()
Definition: Expression.hpp:93
const ExpressionType & operator()() const
Definition: Expression.hpp:103
C ContainerType
Definition: Expression.hpp:167
ExpressionType & operator()()
Definition: Expression.hpp:64
E ExpressionType
Definition: Expression.hpp:57
ExpressionType & operator()()
Definition: Expression.hpp:130
Definition: Expression.hpp:142
ContainerType & operator()()
Definition: Expression.hpp:152
const ContainerType & operator()() const
Definition: Expression.hpp:169
~GridContainer()
Definition: Expression.hpp:225
const ContainerType & operator()() const
Definition: Expression.hpp:213
C ContainerType
Definition: Expression.hpp:145
Definition: Expression.hpp:120
E ExpressionType
Definition: Expression.hpp:101
The namespace of the Chemical Data Processing Library.
ExpressionType & operator()()
Definition: Expression.hpp:108
VectorContainer()
Definition: Expression.hpp:158
Definition: Expression.hpp:208
E ExpressionType
Definition: Expression.hpp:123
const ContainerType & operator()() const
Definition: Expression.hpp:191
MatrixContainer()
Definition: Expression.hpp:180
Definition: VectorExpression.hpp:48
~QuaternionExpression()
Definition: Expression.hpp:115
Definition: Expression.hpp:39
VectorExpression()
Definition: Expression.hpp:70
GridExpression()
Definition: Expression.hpp:136
E ExpressionType
Definition: Expression.hpp:42
~QuaternionContainer()
Definition: Expression.hpp:203
const unsigned int C
Specifies Carbon.
Definition: AtomType.hpp:92
ContainerType & operator()()
Definition: Expression.hpp:218
QuaternionExpression()
Definition: Expression.hpp:114
~VectorExpression()
Definition: Expression.hpp:71
Definition: Expression.hpp:164