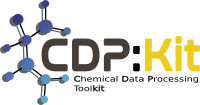 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_SHAPE_EXACTGAUSSIANSHAPEOVERLAPFUNCTION_HPP
30 #define CDPL_SHAPE_EXACTGAUSSIANSHAPEOVERLAPFUNCTION_HPP
44 class GaussianProductList;
50 typedef std::shared_ptr<ExactGaussianShapeOverlapFunction>
SharedPointer;
90 bool checkShapeFuncsNotNull()
const;
92 double calcOverlap(
const GaussianProductList* ref_prod_list,
const GaussianProductList* ovl_prod_list,
94 double calcOverlap(
const GaussianProductList* ref_prod_list,
const GaussianProductList* ovl_prod_list,
97 double calcOverlapGradient(
const GaussianProductList* ref_prod_list,
const GaussianProductList* ovl_prod_list,
108 #endif // CDPL_SHAPE_EXACTGAUSSIANSHAPEOVERLAPFUNCTION_HPP
double calcColorOverlap(const Math::Vector3DArray &coords) const
ExactGaussianShapeOverlapFunction(const GaussianShapeFunction &ref_shape_func, const GaussianShapeFunction &ovl_shape_func)
Definition of the class CDPL::Shape::GaussianShapeOverlapFunction.
void setShapeFunction(const GaussianShapeFunction &func, bool is_ref)
double calcOverlapGradient(const Math::Vector3DArray &coords, Math::Vector3DArray &grad) const
std::function< bool(std::size_t, std::size_t)> ColorMatchFunction
Definition: GaussianShapeOverlapFunction.hpp:55
double calcColorSelfOverlap(bool ref) const
double calcColorOverlap() const
void setColorMatchFunction(const ColorMatchFunction &func)
~ExactGaussianShapeOverlapFunction()
std::shared_ptr< ExactGaussianShapeOverlapFunction > SharedPointer
Definition: ExactGaussianShapeOverlapFunction.hpp:50
double calcSelfOverlap(bool ref) const
Definition: GaussianShapeOverlapFunction.hpp:49
ExactGaussianShapeOverlapFunction()
Definition: GaussianShapeFunction.hpp:53
#define CDPL_SHAPE_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
const ColorMatchFunction & getColorMatchFunction() const
The namespace of the Chemical Data Processing Library.
Definition of the preprocessor macro CDPL_SHAPE_API.
ExactGaussianShapeOverlapFunction(const ExactGaussianShapeOverlapFunction &func)
double calcOverlap(const Math::Vector3DArray &coords) const
double calcOverlap() const
const ColorFilterFunction & getColorFilterFunction() const
const GaussianShapeFunction * getShapeFunction(bool ref) const
std::function< bool(std::size_t)> ColorFilterFunction
Definition: GaussianShapeOverlapFunction.hpp:54
Definition: ExactGaussianShapeOverlapFunction.hpp:47
ExactGaussianShapeOverlapFunction & operator=(const ExactGaussianShapeOverlapFunction &func)
void setColorFilterFunction(const ColorFilterFunction &func)