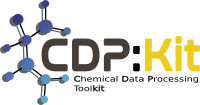 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_FORCEFIELD_ELASTICPOTENTIAL_HPP
30 #define CDPL_FORCEFIELD_ELASTICPOTENTIAL_HPP
48 ElasticPotential(std::size_t atom1_idx, std::size_t atom2_idx,
double force_const,
double ref_length):
49 atom1Idx(atom1_idx), atom2Idx(atom2_idx), forceConst(force_const), refLength(ref_length) {}
85 #endif // CDPL_FORCEFIELD_ELASTICPOTENTIAL_HPP
ElasticPotential(std::size_t atom1_idx, std::size_t atom2_idx, double force_const, double ref_length)
Definition: ElasticPotential.hpp:48
Definition: ElasticPotential.hpp:45
std::size_t getAtom1Index() const
Definition: ElasticPotential.hpp:51
void setReferenceLength(double length)
Definition: ElasticPotential.hpp:71
double getForceConstant() const
Definition: ElasticPotential.hpp:61
The namespace of the Chemical Data Processing Library.
double getReferenceLength() const
Definition: ElasticPotential.hpp:66
std::size_t getAtom2Index() const
Definition: ElasticPotential.hpp:56
VectorNorm2< E >::ResultType length(const VectorExpression< E > &e)
Definition: VectorExpression.hpp:553