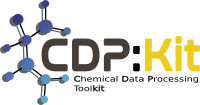 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
27 #ifndef CDPL_MATH_DIRECTASSIGNMENTPROXY_HPP
28 #define CDPL_MATH_DIRECTASSIGNMENTPROXY_HPP
42 typedef typename C::ClosureType ClosureType;
49 lvalue(proxy.lvalue) {}
68 lvalue.minusAssign(e);
75 const DirectAssignmentProxy&
operator=(
const DirectAssignmentProxy&);
94 #endif // CDPL_MATH_DIRECTASSIGNMENTPROXY_HPP
const unsigned int E
Specifies that the stereocenter has E configuration.
Definition: CIPDescriptor.hpp:96
ClosureType & operator+=(const E &e)
Definition: DirectAssignmentProxy.hpp:59
Definition: DirectAssignmentProxy.hpp:39
DirectAssignmentProxy(LValueType &lval)
Definition: DirectAssignmentProxy.hpp:45
The namespace of the Chemical Data Processing Library.
DirectAssignmentProxy(const DirectAssignmentProxy &proxy)
Definition: DirectAssignmentProxy.hpp:48
ClosureType & operator-=(const E &e)
Definition: DirectAssignmentProxy.hpp:66
ClosureType & operator=(const E &e)
Definition: DirectAssignmentProxy.hpp:52
const unsigned int C
Specifies Carbon.
Definition: AtomType.hpp:92
DirectAssignmentProxy< const C > direct(const C &lvalue)
Definition: DirectAssignmentProxy.hpp:81