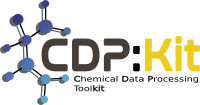 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_BASE_DATAREADER_HPP
30 #define CDPL_BASE_DATAREADER_HPP
167 virtual operator const void*()
const = 0;
206 template <
typename T>
215 template <
typename T>
221 DataIOBase::operator=(reader);
228 template <
typename T>
231 return reader.
read(obj);
236 #endif // CDPL_BASE_DATAREADER_HPP
virtual DataReader & read(DataType &obj, bool overwrite=true)=0
Reads the data record at the current record index and stores the read data in obj.
DataReader< T > & operator>>(DataReader< T > &reader, T &obj)
An input operator that reads the next data record by means of the Base::DataReader instance reader in...
T DataType
The type of the read data objects.
Definition: DataReader.hpp:79
DataReader & operator=(const DataReader &reader)
Assignment operator.
Definition: DataReader.hpp:216
virtual bool operator!() const =0
Tells whether the reader is in a bad state.
virtual std::size_t getRecordIndex() const =0
Returns the index of the current data record.
virtual void setRecordIndex(std::size_t idx)=0
Sets the index of the current data record to idx.
Provides infrastructure for the registration of I/O callback functions.
Definition: DataIOBase.hpp:59
virtual bool hasMoreData()=0
Tells if there are any data records left to read.
An interface for reading data objects of a given type from an arbitrary data source.
Definition: DataReader.hpp:73
virtual DataReader & skip()=0
Skips the data record at the current record index.
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
std::shared_ptr< DataReader > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated DataReader instances.
Definition: DataReader.hpp:84
Definition of the class CDPL::Base::DataIOBase.
The namespace of the Chemical Data Processing Library.
virtual DataReader & read(std::size_t idx, DataType &obj, bool overwrite=true)=0
Reads the data record at index idx and stores the read data in obj.
virtual std::size_t getNumRecords()=0
Returns the total number of available data records.
virtual void close()
Performs a reader specific shutdown operation (if required).
Definition: DataReader.hpp:187