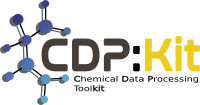 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_BASE_DATAINPUTHANDLER_HPP
30 #define CDPL_BASE_DATAINPUTHANDLER_HPP
86 std::ios_base::openmode mode = std::ios_base::in | std::ios_base::binary)
const = 0;
91 #endif // CDPL_BASE_DATAINPUTHANDLER_HPP
Definition of the class CDPL::Base::DataReader.
An interface for reading data objects of a given type from an arbitrary data source.
Definition: DataReader.hpp:73
std::shared_ptr< DataReader > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated DataReader instances.
Definition: DataReader.hpp:84
The namespace of the Chemical Data Processing Library.