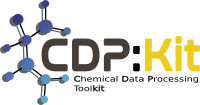 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_CONNECTEDSUBSTRUCTURESET_HPP
30 #define CDPL_CHEM_CONNECTEDSUBSTRUCTURESET_HPP
103 class SubstructDescriptor;
106 typedef SubstructDescriptorCache::SharedObjectPointer SubstructDescriptorPtr;
114 void growSubstructDescriptors(std::size_t);
115 void createSubstructFragments();
117 SubstructDescriptorPtr allocSubstructDescriptor(
const Bond&);
119 const char* getClassName()
const
121 return "ConnectedSubstructureSet";
124 class SubstructDescriptor
135 void copy(
const SubstructDescriptor&);
137 bool operator<(
const SubstructDescriptor&)
const;
140 typedef std::vector<const Atom*> AtomList;
141 typedef std::vector<std::size_t> BondCountList;
146 BondCountList atomBondCounts;
147 std::size_t unsatAListIdx;
148 std::size_t nbrBListIdx;
149 std::size_t lastBondIdx;
151 const Atom* startAtom;
154 struct SubstructDescriptorLessCmpFunc
157 bool operator()(
const SubstructDescriptorPtr&,
const SubstructDescriptorPtr&)
const;
160 typedef std::vector<SubstructDescriptorPtr> SubstructDescriptorList;
162 SubstructDescriptorCache substructDescrCache;
163 SubstructDescriptorList foundSubstructDescriptors;
165 std::size_t currSubstructSize;
166 const MolecularGraph* molGraph;
171 #endif // CDPL_CHEM_CONNECTEDSUBSTRUCTURESET_HPP
Definition of the class CDPL::Util::ObjectPool.
Definition of the preprocessor macro CDPL_CHEM_API.
std::size_t getSubstructureSize() const
Returns the current substructure size in terms of number of bonds.
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Bond.
Definition: Bond.hpp:50
ConnectedSubstructureSet()
Constructs an empty ConnectedSubstructureSet instance.
void findSubstructures(std::size_t size)
Searches the specified molecular graph for connected substructures of the given size.
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
Atom.
Definition: Atom.hpp:52
MolecularGraph.
Definition: MolecularGraph.hpp:52
Definition of the type CDPL::Util::BitSet.
bool operator<(const Array< ValueType > &array1, const Array< ValueType > &array2)
Less than comparison operator.
ConnectedSubstructureSet(const MolecularGraph &molgraph)
Constructs and initialzes a ConnectedSubstructureSet instance for the molecular graph molgraph.
std::shared_ptr< Fragment > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated Fragment instances.
Definition: Fragment.hpp:61
ConnectedSubstructureSet.
Definition: ConnectedSubstructureSet.hpp:52
A data type for the storage of Chem::Fragment objects.
Definition: FragmentList.hpp:49
The namespace of the Chemical Data Processing Library.
Definition of the class CDPL::Chem::FragmentList.
std::shared_ptr< ConnectedSubstructureSet > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated ConnectedSubstructureSet instance...
Definition: ConnectedSubstructureSet.hpp:58
void reset(const MolecularGraph &molgraph)
Specifies the molecular graph that is searched for connected substructures.