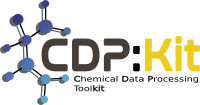 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CONFGEN_MOLECULARGRAPHFUNCTIONS_HPP
30 #define CDPL_CONFGEN_MOLECULARGRAPHFUNCTIONS_HPP
47 class SubstructureSearch;
48 class CommonConnectedSubstructureSearch;
54 class MMFF94InteractionData;
55 class MMFF94InteractionParameterizer;
64 bool het_h_rotors,
bool reset =
true);
67 Util::BitSet& bond_mask,
bool het_h_rotors,
bool reset =
true);
76 bool strict,
double estat_de_const,
double estat_dist_expo);
105 #endif // CDPL_CONFGEN_MOLECULARGRAPHFUNCTIONS_HPP
CDPL_CONFGEN_API std::size_t createRotatableBondMask(const Chem::MolecularGraph &molgraph, Util::BitSet &bond_mask, bool het_h_rotors, bool reset=true)
CDPL_CONFGEN_API std::size_t setupFixedSubstructureData(const Chem::SubstructureSearch &sub_search, std::size_t max_num_matches, Chem::MolecularGraph &molgraph, Chem::Fragment &fixed_substr, Math::Vector3DArray *fixed_substr_coords)
std::vector< ConformerData::SharedPointer > ConformerDataArray
A data type for the storage of dyn. allocated ConfGen::ConformerData objects.
Definition: ConformerDataArray.hpp:46
Definition: MMFF94InteractionData.hpp:51
CDPL_CONFGEN_API void initFixedSubstructureTemplate(Chem::MolecularGraph &molgraph, bool init_match_expr)
SubstructureSearch.
Definition: SubstructureSearch.hpp:64
CDPL_CONFGEN_API void setConformers(Chem::MolecularGraph &molgraph, const ConformerDataArray &conf_array)
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
Fragment.
Definition: Fragment.hpp:52
CommonConnectedSubstructureSearch.
Definition: CommonConnectedSubstructureSearch.hpp:62
MolecularGraph.
Definition: MolecularGraph.hpp:52
Definition of the type CDPL::Util::BitSet.
CDPL_CONFGEN_API bool initFixedSubstructurePattern(Chem::MolecularGraph &molgraph, const Chem::MolecularGraph *tmplt)
Definition of the preprocessor macro CDPL_CONFGEN_API.
#define CDPL_CONFGEN_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
CDPL_CONFGEN_API unsigned int perceiveFragmentType(const Chem::MolecularGraph &molgraph)
CDPL_CONFGEN_API std::size_t getRotatableBondCount(const Chem::MolecularGraph &molgraph, bool het_h_rotors)
CDPL_CONFGEN_API unsigned int parameterizeMMFF94Interactions(const Chem::MolecularGraph &molgraph, ForceField::MMFF94InteractionParameterizer ¶meterizer, ForceField::MMFF94InteractionData ¶m_data, unsigned int ff_type, bool strict, double estat_de_const, double estat_dist_expo)
The namespace of the Chemical Data Processing Library.
CDPL_CONFGEN_API std::size_t createFragmentLinkBondMask(const Chem::MolecularGraph &molgraph, Util::BitSet &bond_mask, bool reset=true)
Definition: MMFF94InteractionParameterizer.hpp:88