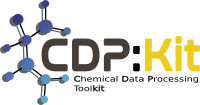 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_UTIL_COMPRESSIONSTREAMS_HPP
30 #define CDPL_UTIL_COMPRESSIONSTREAMS_HPP
34 #include <boost/iostreams/copy.hpp>
35 #include <boost/iostreams/filtering_stream.hpp>
36 #include <boost/iostreams/filter/gzip.hpp>
37 #include <boost/iostreams/filter/bzip2.hpp>
56 template <CompressionAlgo CompAlgo>
75 template <CompressionAlgo CompAlgo,
typename StreamType>
82 typedef typename StreamType::int_type
int_type;
83 typedef typename StreamType::pos_type
pos_type;
84 typedef typename StreamType::off_type
off_type;
85 typedef std::basic_istream<char_type, traits_type>
IStreamType;
86 typedef std::basic_ostream<char_type, traits_type>
OStreamType;
100 typedef std::basic_filebuf<char_type, traits_type> FileBufType;
102 FileBufType tmpFileBuf;
105 template <CompressionAlgo CompAlgo,
typename CharT =
char,
typename TraitsT = std::
char_traits<CharT> >
110 typedef typename std::basic_istream<CharT, TraitsT> StreamType;
120 void open(StreamType& stream);
124 template <CompressionAlgo CompAlgo,
typename CharT =
char,
typename TraitsT = std::
char_traits<CharT> >
129 typedef typename std::basic_ostream<CharT, TraitsT> StreamType;
141 void open(StreamType& stream);
149 template <CompressionAlgo CompAlgo,
typename CharT =
char,
typename TraitsT = std::
char_traits<CharT> >
154 typedef typename std::basic_iostream<CharT, TraitsT> StreamType;
166 void open(StreamType& stream);
186 template <CDPL::Util::CompressionAlgo CompAlgo,
typename StreamType>
188 StreamType(&tmpFileBuf)
191 template <CDPL::Util::CompressionAlgo CompAlgo,
typename StreamType>
194 if (!tmpFileBuf.close())
195 this->setstate(std::ios_base::failbit);
200 template <CDPL::Util::CompressionAlgo CompAlgo,
typename StreamType>
205 if (!tmpFileBuf.open(tmp_file_rem.
getPath().c_str(),
206 std::ios_base::in | std::ios_base::out |
207 std::ios_base::trunc | std::ios_base::binary))
208 this->setstate(std::ios_base::failbit);
210 this->clear(std::ios_base::goodbit);
213 template <CDPL::Util::CompressionAlgo CompAlgo,
typename StreamType>
218 is.seekg(0, std::ios_base::end);
223 this->setstate(std::ios_base::failbit);
232 boost::iostreams::filtering_stream<boost::iostreams::input, char_type, traits_type> fs;
237 boost::iostreams::copy(fs, *this->rdbuf());
239 if (this->tmpFileBuf.pubseekpos(0, std::ios_base::in | std::ios_base::out) != 0)
240 this->setstate(std::ios_base::failbit);
242 this->setstate(is.rdstate() | fs.rdstate());
245 template <CDPL::Util::CompressionAlgo CompAlgo,
typename StreamType>
248 if (tmpFileBuf.pubseekpos(0, std::ios_base::in) != 0) {
249 this->setstate(std::ios_base::failbit);
253 boost::iostreams::filtering_stream<boost::iostreams::output, char_type, traits_type> fs;
258 boost::iostreams::copy(*this->rdbuf(), fs);
260 this->setstate(os.rdstate() | fs.rdstate());
265 template <CDPL::Util::CompressionAlgo CompAlgo,
typename CharT,
typename TraitsT>
269 template <CDPL::Util::CompressionAlgo CompAlgo,
typename CharT,
typename TraitsT>
275 template <CDPL::Util::CompressionAlgo CompAlgo,
typename CharT,
typename TraitsT>
281 this->decompInput(stream);
284 template <CDPL::Util::CompressionAlgo CompAlgo,
typename CharT,
typename TraitsT>
287 this->closeTmpFile();
292 template <CDPL::Util::CompressionAlgo CompAlgo,
typename CharT,
typename TraitsT>
297 template <CDPL::Util::CompressionAlgo CompAlgo,
typename CharT,
typename TraitsT>
304 template <CDPL::Util::CompressionAlgo CompAlgo,
typename CharT,
typename TraitsT>
309 stream->seekp(outPos);
310 this->compOutput(*stream);
315 template <CDPL::Util::CompressionAlgo CompAlgo,
typename CharT,
typename TraitsT>
323 outPos = stream.tellp();
324 this->setstate(stream.rdstate());
327 this->stream = &stream;
330 template <CDPL::Util::CompressionAlgo CompAlgo,
typename CharT,
typename TraitsT>
334 stream->seekp(outPos);
335 this->compOutput(*stream);
343 this->closeTmpFile();
348 template <CDPL::Util::CompressionAlgo CompAlgo,
typename CharT,
typename TraitsT>
353 template <CDPL::Util::CompressionAlgo CompAlgo,
typename CharT,
typename TraitsT>
360 template <CDPL::Util::CompressionAlgo CompAlgo,
typename CharT,
typename TraitsT>
365 stream->seekp(outPos);
366 this->compOutput(*stream);
371 template <CDPL::Util::CompressionAlgo CompAlgo,
typename CharT,
typename TraitsT>
379 outPos = stream.tellp();
380 this->setstate(stream.rdstate());
385 this->decompInput(stream);
388 this->stream = &stream;
391 template <CDPL::Util::CompressionAlgo CompAlgo,
typename CharT,
typename TraitsT>
395 stream->seekp(outPos);
396 this->compOutput(*stream);
404 this->closeTmpFile();
407 #endif // CDPL_UTIL_COMPRESSIONSTREAMS_HPP
Definition: CompressionStreams.hpp:107
StreamType::pos_type pos_type
Definition: CompressionStreams.hpp:133
StreamType::char_type char_type
Definition: CompressionStreams.hpp:155
boost::iostreams::bzip2_decompressor DecompFilter
Definition: CompressionStreams.hpp:71
boost::iostreams::gzip_decompressor DecompFilter
Definition: CompressionStreams.hpp:63
void close()
Definition: CompressionStreams.hpp:392
StreamType::char_type char_type
Definition: CompressionStreams.hpp:80
DecompressionIStream()
Definition: CompressionStreams.hpp:266
StreamType::traits_type traits_type
Definition: CompressionStreams.hpp:112
CompressedIOStream< BZIP2 > BZip2IOStream
Definition: CompressionStreams.hpp:179
std::basic_istream< char_type, traits_type > IStreamType
Definition: CompressionStreams.hpp:85
Definition: FileRemover.hpp:44
StreamType::traits_type traits_type
Definition: CompressionStreams.hpp:81
CompressedIOStream()
Definition: CompressionStreams.hpp:349
@ BZIP2
Definition: CompressionStreams.hpp:53
void open(StreamType &stream)
Definition: CompressionStreams.hpp:316
std::basic_ostream< char_type, traits_type > OStreamType
Definition: CompressionStreams.hpp:86
void closeTmpFile()
Definition: CompressionStreams.hpp:192
StreamType::int_type int_type
Definition: CompressionStreams.hpp:82
CompressionOStream< BZIP2 > BZip2OStream
Definition: CompressionStreams.hpp:177
StreamType::char_type char_type
Definition: CompressionStreams.hpp:130
const std::string & getPath() const
Definition of the class CDPL::Util::FileRemover.
StreamType::pos_type pos_type
Definition: CompressionStreams.hpp:114
CompressionAlgo
Definition: CompressionStreams.hpp:50
Definition: CompressionStreams.hpp:77
void open(StreamType &stream)
Definition: CompressionStreams.hpp:276
Definition: CompressionStreams.hpp:151
void decompInput(IStreamType &is)
Definition: CompressionStreams.hpp:214
CompressionOStream()
Definition: CompressionStreams.hpp:293
virtual ~CompressionStreamBase()
Definition: CompressionStreams.hpp:91
StreamType::pos_type pos_type
Definition: CompressionStreams.hpp:83
void close()
Definition: CompressionStreams.hpp:285
StreamType::traits_type traits_type
Definition: CompressionStreams.hpp:156
CompressionOStream< GZIP > GZipOStream
Definition: CompressionStreams.hpp:176
StreamType::off_type off_type
Definition: CompressionStreams.hpp:159
Definition: CompressionStreams.hpp:57
StreamType::int_type int_type
Definition: CompressionStreams.hpp:132
@ GZIP
Definition: CompressionStreams.hpp:52
StreamType::char_type char_type
Definition: CompressionStreams.hpp:111
The namespace of the Chemical Data Processing Library.
void open(StreamType &stream)
Definition: CompressionStreams.hpp:372
StreamType::pos_type pos_type
Definition: CompressionStreams.hpp:158
CompressedIOStream< GZIP > GZipIOStream
Definition: CompressionStreams.hpp:178
boost::iostreams::gzip_compressor CompFilter
Definition: CompressionStreams.hpp:64
CDPL_UTIL_API std::string genCheckedTempFilePath(const std::string &dir="", const std::string &ptn="%%%%-%%%%-%%%%-%%%%")
Definition: CompressionStreams.hpp:126
StreamType::off_type off_type
Definition: CompressionStreams.hpp:115
StreamType::off_type off_type
Definition: CompressionStreams.hpp:134
~CompressionOStream()
Definition: CompressionStreams.hpp:305
StreamType::int_type int_type
Definition: CompressionStreams.hpp:113
void close()
Definition: CompressionStreams.hpp:331
~CompressedIOStream()
Definition: CompressionStreams.hpp:361
CompressionStreamBase()
Definition: CompressionStreams.hpp:187
DecompressionIStream< BZIP2 > BZip2IStream
Definition: CompressionStreams.hpp:175
StreamType::off_type off_type
Definition: CompressionStreams.hpp:84
StreamType::int_type int_type
Definition: CompressionStreams.hpp:157
void compOutput(OStreamType &os)
Definition: CompressionStreams.hpp:246
StreamType::traits_type traits_type
Definition: CompressionStreams.hpp:131
boost::iostreams::bzip2_compressor CompFilter
Definition: CompressionStreams.hpp:72
DecompressionIStream< GZIP > GZipIStream
Definition: CompressionStreams.hpp:174
Declaration of filesystem-related functions.
void openTmpFile()
Definition: CompressionStreams.hpp:201