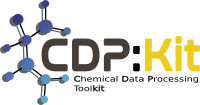 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_UTIL_COMPRESSEDDATAWRITER_HPP
30 #define CDPL_UTIL_COMPRESSEDDATAWRITER_HPP
48 template <
typename WriterImpl,
typename CompStream,
typename DataType =
typename WriterImpl::DataType>
59 operator const void*()
const;
73 template <
typename WriterImpl,
typename DecompStream,
typename DataType>
75 stream(ios), writer(stream)
77 writer.setParent(
this);
81 template <
typename WriterImpl,
typename DecompStream,
typename DataType>
90 template <
typename WriterImpl,
typename DecompStream,
typename DataType>
97 template <
typename WriterImpl,
typename DecompStream,
typename DataType>
100 return writer.operator
const void*();
103 template <
typename WriterImpl,
typename DecompStream,
typename DataType>
106 return writer.operator!();
109 #endif // CDPL_UTIL_COMPRESSEDDATAWRITER_HPP
CompressedDataWriter.
Definition: CompressedDataWriter.hpp:50
CompressedDataWriter & write(const DataType &obj)
Definition: CompressedDataWriter.hpp:83
Definition of the class CDPL::Base::DataWriter.
void invokeIOCallbacks(double progress) const
Invokes all registered I/O callback functions with the argument *this.
void close()
Writes format dependent data (if required) to mark the end of output.
Definition: CompressedDataWriter.hpp:91
bool operator!() const
Definition: CompressedDataWriter.hpp:104
CompressedDataWriter(std::iostream &ios)
Definition: CompressedDataWriter.hpp:74
typename WriterImpl::DataType DataType
The type of the written data objects.
Definition: DataWriter.hpp:74
The namespace of the Chemical Data Processing Library.
Provides I/O-streams capable of performing compression and decompression.
An interface for writing data objects of a given type to an arbitrary data sink.
Definition: DataWriter.hpp:63