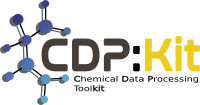 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_UTIL_COMPRESSEDDATAREADER_HPP
30 #define CDPL_UTIL_COMPRESSEDDATAREADER_HPP
48 template <
typename ReaderImpl,
typename DecompStream,
typename DataType =
typename ReaderImpl::DataType>
67 operator const void*()
const;
82 template <
typename ReaderImpl,
typename DecompStream,
typename DataType>
84 stream(is), reader(stream)
86 reader.setParent(
this);
90 template <
typename ReaderImpl,
typename DecompStream,
typename DataType>
94 reader.
read(obj, overwrite);
99 template <
typename ReaderImpl,
typename DecompStream,
typename DataType>
103 reader.
read(idx, obj, overwrite);
108 template <
typename ReaderImpl,
typename DecompStream,
typename DataType>
117 template <
typename ReaderImpl,
typename DecompStream,
typename DataType>
120 return reader.hasMoreData();
123 template <
typename ReaderImpl,
typename DecompStream,
typename DataType>
126 return reader.getRecordIndex();
129 template <
typename ReaderImpl,
typename DecompStream,
typename DataType>
132 reader.setRecordIndex(idx);
135 template <
typename ReaderImpl,
typename DecompStream,
typename DataType>
138 return reader.getNumRecords();
141 template <
typename ReaderImpl,
typename DecompStream,
typename DataType>
144 return reader.operator
const void*();
147 template <
typename ReaderImpl,
typename DecompStream,
typename DataType>
150 return reader.operator!();
153 template <
typename ReaderImpl,
typename DecompStream,
typename DataType>
160 #endif // CDPL_UTIL_COMPRESSEDDATAREADER_HPP
void close()
Performs a reader specific shutdown operation (if required).
Definition: CompressedDataReader.hpp:154
void setRecordIndex(std::size_t idx)
Sets the index of the current data record to idx.
Definition: CompressedDataReader.hpp:130
typename ReaderImpl::DataType DataType
The type of the read data objects.
Definition: DataReader.hpp:79
Definition of the class CDPL::Base::DataReader.
void invokeIOCallbacks(double progress) const
Invokes all registered I/O callback functions with the argument *this.
std::size_t getNumRecords()
Returns the total number of available data records.
Definition: CompressedDataReader.hpp:136
An interface for reading data objects of a given type from an arbitrary data source.
Definition: DataReader.hpp:73
CompressedDataReader & read(DataType &obj, bool overwrite=true)
Definition: CompressedDataReader.hpp:92
bool hasMoreData()
Tells if there are any data records left to read.
Definition: CompressedDataReader.hpp:118
CompressedDataReader & skip()
Skips the data record at the current record index.
Definition: CompressedDataReader.hpp:110
The namespace of the Chemical Data Processing Library.
CompressedDataReader.
Definition: CompressedDataReader.hpp:50
std::size_t getRecordIndex() const
Definition: CompressedDataReader.hpp:124
Provides I/O-streams capable of performing compression and decompression.
bool operator!() const
Definition: CompressedDataReader.hpp:148
CompressedDataReader(std::istream &is)
Definition: CompressedDataReader.hpp:83