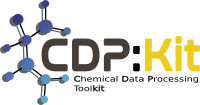 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
32 #ifndef CDPL_CHEM_COMPLETERINGSET_HPP
33 #define CDPL_CHEM_COMPLETERINGSET_HPP
102 EdgePtr allocEdge(
const EdgePtr&,
const EdgePtr&, Node*, Node*,
bool);
103 EdgePtr allocEdge(
const Bond&, Node*, Node*);
105 const char* getClassName()
const
107 return "CompleteRingSet";
113 typedef std::list<EdgePtr> EdgeList;
116 typedef EdgeList::iterator EdgeIterator;
124 Node(std::size_t idx):
127 EdgeIterator addEdge(
const EdgePtr&);
128 void removeEdge(
const EdgeIterator&);
130 EdgeIterator getEdgesBegin();
131 EdgeIterator getEdgesEnd();
133 std::size_t getIndex()
const;
144 void init(
const EdgePtr& this_edge,
const MolecularGraph*,
const Bond&, Node*, Node*);
145 void init(
const EdgePtr& this_edge,
const EdgePtr&,
const EdgePtr&, Node*, Node*,
bool);
147 bool intersects(
const EdgePtr&)
const;
151 Node* getNeighbor(
const Node*)
const;
152 const Node::EdgeIterator& getEdgeListIterator(
const Node*)
const;
156 Node::EdgeIterator edgeListIters[2];
162 typedef std::vector<Node> NodeArray;
163 typedef std::priority_queue<Node*, std::vector<Node*>, Node::GreaterCmpFunc> NodeQueue;
166 const MolecularGraph* molGraph;
173 #endif // CDPL_CHEM_COMPLETERINGSET_HPP
void perceive(const MolecularGraph &molgraph)
Replaces the current set of rings by the rings in the molecular graph molgraph.
Definition of the class CDPL::Util::ObjectPool.
std::shared_ptr< CompleteRingSet > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated CompleteRingSet instances.
Definition: CompleteRingSet.hpp:64
Definition of the preprocessor macro CDPL_CHEM_API.
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Bond.
Definition: Bond.hpp:50
Implements the exhaustive perception of rings in a molecular graph.
Definition: CompleteRingSet.hpp:58
CompleteRingSet(const MolecularGraph &molgraph)
Constructs a CompleteRingSet instance that contains the rings in the molecular graph molgraph.
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
MolecularGraph.
Definition: MolecularGraph.hpp:52
Definition of the type CDPL::Util::BitSet.
std::shared_ptr< ObjectType > SharedObjectPointer
Definition: ObjectPool.hpp:65
std::shared_ptr< Fragment > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated Fragment instances.
Definition: Fragment.hpp:61
A data type for the storage of Chem::Fragment objects.
Definition: FragmentList.hpp:49
Definition: CompleteRingSet.hpp:119
CompleteRingSet()
Constructs an empty CompleteRingSet instance.
The namespace of the Chemical Data Processing Library.
bool operator()(const Node *, const Node *) const
~CompleteRingSet()
Destructor.
Definition of the class CDPL::Chem::FragmentList.