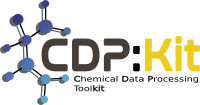 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_VIS_COLOR_HPP
30 #define CDPL_VIS_COLOR_HPP
162 Color(
double red,
double green,
double blue,
double alpha = 1.0);
239 void setRGBA(
double red,
double green,
double blue,
double alpha = 1.0);
267 #endif // CDPL_VIS_COLOR_HPP
static const Color GREEN
A preinitialized Color instance for the color green.
Definition: Color.hpp:78
void setRGBA(double red, double green, double blue, double alpha=1.0)
Sets the values of the RGBA components to specified values.
double getRed() const
Returns the value of the red color component.
bool operator==(const Color &color) const
Equality comparison operator.
static const Color BLUE
A preinitialized Color instance for the color blue.
Definition: Color.hpp:88
static const Color DARK_YELLOW
A preinitialized Color instance for the color dark yellow.
Definition: Color.hpp:123
static const Color DARK_GRAY
A preinitialized Color instance for the color dark gray.
Definition: Color.hpp:138
static const Color TRANSPARENT
A preinitialized Color instance for a totally transparent (alpha = 0) black.
Definition: Color.hpp:143
static const Color LIGHT_GRAY
A preinitialized Color instance for the color light gray.
Definition: Color.hpp:133
void setRed(double red)
Sets the value of the red color component to red.
static const Color BLACK
A preinitialized Color instance for the color black.
Definition: Color.hpp:58
static const Color CYAN
A preinitialized Color instance for the color cyan.
Definition: Color.hpp:98
void setGreen(double green)
Sets the the value of green color component to green.
double getGreen() const
Returns the value of the green color component.
static const Color WHITE
A preinitialized Color instance for the color white.
Definition: Color.hpp:63
Color()
Constructs and initializes a Color object with all RGBA components set to zero.
static const Color DARK_MAGENTA
A preinitialized Color instance for the color dark magenta.
Definition: Color.hpp:113
double getAlpha() const
Returns the value of the alpha component.
static const Color DARK_CYAN
A preinitialized Color instance for the color dark cyan.
Definition: Color.hpp:103
#define CDPL_VIS_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
static const Color DARK_GREEN
A preinitialized Color instance for the color dark green.
Definition: Color.hpp:83
double getBlue() const
Returns the value of the blue color component.
static const Color YELLOW
A preinitialized Color instance for the color yellow.
Definition: Color.hpp:118
static const Color MAGENTA
A preinitialized Color instance for the color magenta.
Definition: Color.hpp:108
static const Color GRAY
A preinitialized Color instance for the color gray.
Definition: Color.hpp:128
The namespace of the Chemical Data Processing Library.
Color(double red, double green, double blue, double alpha=1.0)
Constructs and initializes a Color object with the RGBA components set to the specified values.
static const Color RED
A preinitialized Color instance for the color red.
Definition: Color.hpp:68
static const Color DARK_RED
A preinitialized Color instance for the color dark red.
Definition: Color.hpp:73
static const Color DARK_BLUE
A preinitialized Color instance for the color dark blue.
Definition: Color.hpp:93
void setBlue(double blue)
Sets the value of the blue color component to blue.
Definition of the preprocessor macro CDPL_VIS_API.
void setAlpha(double alpha)
Sets the value of the alpha component to alpha.
bool operator!=(const Color &color) const
Inequality comparison operator.
Specifies a color in terms of its red, green and blue components and an alpha-channel for transparenc...
Definition: Color.hpp:52