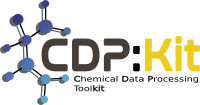 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_REACTIONFUNCTIONS_HPP
30 #define CDPL_CHEM_REACTIONFUNCTIONS_HPP
139 bool ord_h_deplete =
true);
239 #endif // CDPL_CHEM_REACTIONFUNCTIONS_HPP
CDPL_CHEM_API void setComponentMatchConstraints(Reaction &rxn, const MatchConstraintList::SharedPointer &constr, bool overwrite)
CDPL_CHEM_API const std::string & getMDLExternalRegistryNumber(const Reaction &rxn)
Definition of the class CDPL::Chem::Molecule.
CDPL_CHEM_API void setMDLExternalRegistryNumber(Reaction &rxn, const std::string ®_no)
CDPL_CHEM_API void setAtomMapping(Reaction &rxn, const AtomMapping::SharedPointer &mapping)
Definition of constants in namespace CDPL::Chem::ReactionRole.
CDPL_CHEM_API void clearMDLInternalRegistryNumber(Reaction &rxn)
CDPL_CHEM_API const std::string & getMDLInternalRegistryNumber(const Reaction &rxn)
CDPL_CHEM_API unsigned int getMDLRXNFileVersion(const Reaction &rxn)
Definition of the preprocessor macro CDPL_CHEM_API.
CDPL_CHEM_API void clearMDLMoleculeRecord(Reaction &rxn)
CDPL_CHEM_API const std::string & getMDLUserInitials(const MolecularGraph &molgraph)
CDPL_CHEM_API bool hasTimestamp(const MolecularGraph &molgraph)
CDPL_CHEM_API const AtomMapping::SharedPointer & getAtomMapping(const Reaction &rxn)
CDPL_CHEM_API bool hasMDLRegistryNumber(const MolecularGraph &molgraph)
CDPL_CHEM_API void setMDLMoleculeRecord(Reaction &rxn, const Molecule::SharedPointer &mol_rec)
CDPL_CHEM_API void setMDLRegistryNumber(MolecularGraph &molgraph, std::size_t reg_no)
CDPL_CHEM_API bool hasReactionData(const Reaction &rxn)
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
CDPL_CHEM_API void generateMatchExpressions(MolecularGraph &molgraph, bool overwrite)
CDPL_CHEM_API void clearTimestamp(MolecularGraph &molgraph)
CDPL_CHEM_API void initSubstructureSearchQuery(MolecularGraph &molgraph, bool overwrite)
CDPL_CHEM_API const std::string & getMDLProgramName(const MolecularGraph &molgraph)
CDPL_CHEM_API const StringDataBlock::SharedPointer & getReactionData(const Reaction &rxn)
CDPL_CHEM_API void setMatchExpression(Atom &atom, const MatchExpression< Atom, MolecularGraph >::SharedPointer &expr)
CDPL_CHEM_API std::uint64_t calcHashCode(const MolecularGraph &molgraph, unsigned int atom_flags=AtomPropertyFlag::DEFAULT, unsigned int bond_flags=BondPropertyFlag::DEFAULT, bool ord_h_deplete=true)
CDPL_CHEM_API std::size_t getMaxComponentGroupID(const AtomContainer &cntnr)
CDPL_CHEM_API void setReactionData(Reaction &rxn, const StringDataBlock::SharedPointer &data)
const unsigned int ALL
Specifies reactants, agents and products of a reaction.
Definition: ReactionRole.hpp:74
CDPL_CHEM_API void setAtomMatchConstraints(MolecularGraph &molgraph, const MatchConstraintList::SharedPointer &constr, bool overwrite)
std::shared_ptr< AtomMapping > SharedPointer
Definition: AtomMapping.hpp:57
std::shared_ptr< MatchConstraintList > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated MatchConstraintList instances.
Definition: MatchConstraintList.hpp:197
std::shared_ptr< StringDataBlock > SharedPointer
Definition: StringDataBlock.hpp:128
CDPL_CHEM_API void clearMatchExpression(Atom &atom)
CDPL_CHEM_API bool hasMatchExpression(const Atom &atom)
CDPL_CHEM_API bool hasAtomMapping(const Reaction &rxn)
CDPL_CHEM_API void setBondMatchConstraints(MolecularGraph &molgraph, const MatchConstraintList::SharedPointer &constr, bool overwrite)
std::shared_ptr< Molecule > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated Molecule instances.
Definition: Molecule.hpp:55
CDPL_CHEM_API void clearComponentGroups(MolecularGraph &molgraph)
CDPL_CHEM_API MatchExpression< Atom, MolecularGraph >::SharedPointer generateMatchExpression(const Atom &atom, const MolecularGraph &molgraph)
CDPL_CHEM_API bool hasComponentGroups(const MolecularGraph &molgraph)
CDPL_CHEM_API void clearName(Atom &atom)
std::shared_ptr< FragmentList > SharedPointer
Definition: FragmentList.hpp:52
CDPL_CHEM_API void setComponentGroups(MolecularGraph &molgraph, const FragmentList::SharedPointer &comp_groups)
CDPL_CHEM_API bool hasMDLMoleculeRecord(const Reaction &rxn)
CDPL_CHEM_API void setComment(MolecularGraph &molgraph, const std::string &comment)
Definition of constants in namespace CDPL::Chem::AtomPropertyFlag.
CDPL_CHEM_API FragmentList::SharedPointer perceiveComponentGroups(const MolecularGraph &molgraph)
CDPL_CHEM_API const std::string & getName(const Atom &atom)
CDPL_CHEM_API bool hasComment(const MolecularGraph &molgraph)
CDPL_CHEM_API void clearMDLRegistryNumber(MolecularGraph &molgraph)
CDPL_CHEM_API void calcBasicProperties(MolecularGraph &molgraph, bool overwrite)
CDPL_CHEM_API void setMDLUserInitials(MolecularGraph &molgraph, const std::string &initials)
CDPL_CHEM_API void clearComment(MolecularGraph &molgraph)
CDPL_CHEM_API void clearReactionData(Reaction &rxn)
CDPL_CHEM_API AtomMapping::SharedPointer perceiveAtomMapping(const Reaction &rxn)
CDPL_CHEM_API void initSubstructureSearchTarget(MolecularGraph &molgraph, bool overwrite)
CDPL_CHEM_API void clearAtomMapping(Reaction &rxn)
CDPL_CHEM_API bool hasMDLUserInitials(const MolecularGraph &molgraph)
CDPL_CHEM_API void clearMatchConstraints(Atom &atom)
const unsigned int DEFAULT
Represents the default set of atom properties.
Definition: Chem/AtomPropertyFlag.hpp:53
CDPL_CHEM_API bool hasMDLRXNFileVersion(const Reaction &rxn)
CDPL_CHEM_API std::size_t getMaxAtomMappingID(const AtomContainer &cntnr)
CDPL_CHEM_API const Molecule::SharedPointer & getMDLMoleculeRecord(const Reaction &rxn)
CDPL_CHEM_API bool hasMDLProgramName(const MolecularGraph &molgraph)
A generic boolean expression interface for the implementation of query/target object equivalence test...
Definition: MatchExpression.hpp:75
CDPL_CHEM_API const std::string & getComment(const MolecularGraph &molgraph)
CDPL_CHEM_API bool generateSMILES(const MolecularGraph &molgraph, std::string &smiles, bool canonical=false, bool ord_h_deplete=true, unsigned int atom_flags=AtomPropertyFlag::DEFAULT, unsigned int bond_flags=BondPropertyFlag::DEFAULT)
CDPL_CHEM_API std::time_t getTimestamp(const MolecularGraph &molgraph)
Definition of the class CDPL::Chem::MatchExpression.
The namespace of the Chemical Data Processing Library.
CDPL_CHEM_API bool hasMDLInternalRegistryNumber(const Reaction &rxn)
CDPL_CHEM_API void setMDLProgramName(MolecularGraph &molgraph, const std::string &name)
CDPL_CHEM_API std::size_t getMDLRegistryNumber(const MolecularGraph &molgraph)
Reaction.
Definition: Reaction.hpp:52
CDPL_CHEM_API void setTimestamp(MolecularGraph &molgraph, std::time_t time)
CDPL_CHEM_API bool hasName(const Atom &atom)
CDPL_CHEM_API void clearMDLExternalRegistryNumber(Reaction &rxn)
CDPL_CHEM_API const MatchExpression< Atom, MolecularGraph >::SharedPointer & getMatchExpression(const Atom &atom)
CDPL_CHEM_API void clearMDLUserInitials(MolecularGraph &molgraph)
CDPL_CHEM_API void setName(Atom &atom, const std::string &name)
CDPL_CHEM_API void clearMDLProgramName(MolecularGraph &molgraph)
Definition of the type CDPL::Chem::AtomMapping.
CDPL_CHEM_API void setMDLInternalRegistryNumber(Reaction &rxn, const std::string ®_no)
CDPL_CHEM_API void setMatchConstraints(Atom &atom, const MatchConstraintList::SharedPointer &constr)
Definition of constants in namespace CDPL::Chem::BondPropertyFlag.
CDPL_CHEM_API bool hasMatchConstraints(const Atom &atom)
Definition of the class CDPL::Chem::MatchConstraintList.
Definition of the class CDPL::Chem::FragmentList.
CDPL_CHEM_API void setMDLRXNFileVersion(Reaction &rxn, unsigned int version)
CDPL_CHEM_API bool hasMDLExternalRegistryNumber(const Reaction &rxn)
Definition of the class CDPL::Chem::StringDataBlockItem and the type CDPL::Chem::StringDataBlock.
CDPL_CHEM_API const MatchConstraintList::SharedPointer & getMatchConstraints(const Atom &atom)
CDPL_CHEM_API const FragmentList::SharedPointer & getComponentGroups(const MolecularGraph &molgraph)
const unsigned int DEFAULT
Represents the default set of bond properties.
Definition: BondPropertyFlag.hpp:53
CDPL_CHEM_API void clearMDLRXNFileVersion(Reaction &rxn)