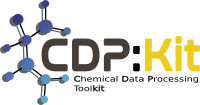 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_ATOMCONTAINERFUNCTIONS_HPP
30 #define CDPL_CHEM_ATOMCONTAINERFUNCTIONS_HPP
132 #endif // CDPL_CHEM_ATOMCONTAINERFUNCTIONS_HPP
Definition of the preprocessor macro CDPL_CHEM_API.
CDPL_CHEM_API bool calcCenterOfMass(const AtomContainer &cntnr, const Atom3DCoordinatesFunction &coords_func, Math::Vector3D &ctr)
Definition of the class CDPL::Math::VectorArray.
CDPL_CHEM_API void getConformation(const AtomContainer &cntnr, std::size_t conf_idx, Math::Vector3DArray &coords, bool append=false)
CDPL_CHEM_API std::size_t getNumConformations(const AtomContainer &cntnr)
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
CVector< double, 3 > Vector3D
A bounded 3 element vector holding floating point values of type double.
Definition: Vector.hpp:1637
CDPL_CHEM_API void get2DCoordinates(const AtomContainer &cntnr, Math::Vector2DArray &coords, bool append=false)
CDPL_CHEM_API std::size_t getMaxComponentGroupID(const AtomContainer &cntnr)
CDPL_CHEM_API void copyAtomsIfNot(const AtomContainer &cntnr, Molecule &mol, const AtomPredicate &pred, bool append=false)
CDPL_CHEM_API bool hasCoordinates(const AtomContainer &cntnr, std::size_t dim)
CDPL_CHEM_API bool calcCentroid(const AtomContainer &cntnr, const Atom3DCoordinatesFunction &coords_func, Math::Vector3D &ctr)
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
CDPL_CHEM_API void transform2DCoordinates(AtomContainer &cntnr, const Math::Matrix3D &mtx)
Fragment.
Definition: Fragment.hpp:52
CDPL_CHEM_API void addConformation(AtomContainer &cntnr, const Math::Vector3DArray &coords)
Definition of the type CDPL::Util::BitSet.
Type definition of a generic wrapper class for storing user-defined Chem::Atom 3D-coordinates functio...
Molecule.
Definition: Molecule.hpp:49
std::function< bool(const Chem::Atom &)> AtomPredicate
A generic wrapper class used to store a user-defined atom predicate.
Definition: AtomPredicate.hpp:41
A common interface for data-structures that support a random access to stored Chem::Atom instances.
Definition: AtomContainer.hpp:55
CDPL_CHEM_API void applyConformation(AtomContainer &cntnr, std::size_t conf_idx)
CDPL_CHEM_API void setConformation(AtomContainer &cntnr, std::size_t conf_idx, const Math::Vector3DArray &coords)
Type definition of a generic wrapper class for storing user-defined Chem::Atom predicates.
CDPL_CHEM_API void copyAtomsIf(const AtomContainer &cntnr, Molecule &mol, const AtomPredicate &pred, bool append=false)
CDPL_CHEM_API void clearConformations(AtomContainer &cntnr)
CDPL_CHEM_API std::size_t getMaxAtomMappingID(const AtomContainer &cntnr)
std::function< const Math::Vector3D &(const Chem::Atom &)> Atom3DCoordinatesFunction
A generic wrapper class used to store a user-defined Chem::Atom 3D-coordinates function.
Definition: Atom3DCoordinatesFunction.hpp:43
CMatrix< double, 3, 3 > Matrix3D
A bounded 3x3 matrix holding floating point values of type double.
Definition: Matrix.hpp:1849
CDPL_CHEM_API bool insideBoundingBox(const AtomContainer &cntnr, const Math::Vector3D &min, const Math::Vector3D &max, const Atom3DCoordinatesFunction &coords_func)
The namespace of the Chemical Data Processing Library.
CDPL_CHEM_API bool alignConformations(AtomContainer &cntnr, const Util::BitSet &ref_atoms, const Math::Vector3DArray &ref_coords)
CDPL_CHEM_API bool intersectsBoundingBox(const AtomContainer &cntnr, const Math::Vector3D &min, const Math::Vector3D &max, const Atom3DCoordinatesFunction &coords_func)
CDPL_CHEM_API std::size_t createAtomTypeMask(const AtomContainer &cntnr, Util::BitSet &mask, unsigned int type, bool reset=true, bool strict=true)
Definition of matrix data types.
VectorArray< Vector2D > Vector2DArray
An array of Math::Vector2D objects.
Definition: VectorArray.hpp:79
CDPL_CHEM_API void calcBoundingBox(const AtomContainer &cntnr, Math::Vector3D &min, Math::Vector3D &max, const Atom3DCoordinatesFunction &coords_func, bool reset=true)
CDPL_CHEM_API void transformConformations(AtomContainer &cntnr, const Math::Matrix4D &mtx)
CDPL_CHEM_API void transformConformation(AtomContainer &cntnr, std::size_t conf_idx, const Math::Matrix4D &mtx)
CDPL_CHEM_API void get3DCoordinates(const AtomContainer &cntnr, Math::Vector3DArray &coords, const Atom3DCoordinatesFunction &coords_func, bool append=false)
CDPL_CHEM_API void set2DCoordinates(AtomContainer &cntnr, const Math::Vector2DArray &coords)