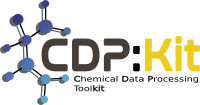 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
32 #ifndef CDPL_CHEM_CHEMBLSTANDARDIZER_HPP
33 #define CDPL_CHEM_CHEMBLSTANDARDIZER_HPP
38 #include <unordered_set>
41 #include <boost/functional/hash.hpp>
75 EXPLICIT_HYDROGENS_REMOVED = 0x2,
76 UNKNOWN_STEREO_STANDARDIZED = 0x4,
77 BONDS_KEKULIZED = 0x8,
78 STRUCTURE_NORMALIZED = 0x10,
79 CHARGES_REMOVED = 0x20,
80 TARTRATE_STEREO_CLEARED = 0x40,
81 STRUCTURE_2D_CORRECTED = 0x80,
82 ISOTOPE_INFO_CLEARED = 0x100,
83 SALT_COMPONENTS_REMOVED = 0x200,
84 SOLVENT_COMPONENTS_REMOVED = 0x400,
85 DUPLICATE_COMPONENTS_REMOVED = 0x800
103 typedef std::vector<Atom*> AtomList;
107 bool checkExclusionCriterions(
const Molecule& mol)
const;
108 bool checkExclusionCriterions(
const MolecularGraph& molgraph, std::size_t& boron_cnt)
const;
110 bool standardizeUnknownStereochemistry(
Molecule& mol)
const;
114 bool removeExplicitHydrogens(
Molecule& mol)
const;
115 bool isRemovableHydrogen(
const Atom& atom)
const;
117 bool normalizeStructure(
Molecule& mol);
122 bool removeTartrateStereochemistry(
Molecule& mol);
124 bool cleanup2DStructure(
Molecule& mol);
125 double calc2DBondAngle(
const Molecule& mol,
const Atom& ctr_atom,
const Atom& nbr_atom1,
const Atom& nbr_atom2);
126 void rotateSubstituent(
const Molecule& mol,
const Atom& ctr_atom,
const Atom& subst_atom,
double rot_ang);
130 typedef std::pair<std::uint64_t, std::uint64_t> StructureID;
131 typedef std::pair<const Fragment*, StructureID> MoleculeComponent;
132 typedef std::vector<MoleculeComponent> MoleculeComponentList;
133 typedef std::unordered_set<StructureID, boost::hash<StructureID> > StructureIDSet;
144 MoleculeComponentList molCompList1;
145 MoleculeComponentList molCompList2;
146 StructureIDSet uniqueMolComps;
151 #endif // CDPL_CHEM_CHEMBLSTANDARDIZER_HPP
Definition of the preprocessor macro CDPL_CHEM_API.
Definition of the class CDPL::Math::VectorArray.
ChangeFlags getParent(Molecule &mol, bool neutralize=true, bool check_exclusion=true)
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Implementation of the ChEMBL structure preprocessing pipeline.
Definition: ChEMBLStandardizer.hpp:65
SubstructureSearch.
Definition: SubstructureSearch.hpp:64
ChangeFlags
Definition: ChEMBLStandardizer.hpp:71
Definition of the class CDPL::Chem::HashCodeCalculator.
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
BasicMolecule.
Definition: BasicMolecule.hpp:54
ChangeFlags getParent(const Molecule &mol, Molecule &parent_mol, bool neutralize=true, bool check_exclusion=true)
Atom.
Definition: Atom.hpp:52
Fragment.
Definition: Fragment.hpp:52
KekuleStructureCalculator.
Definition: KekuleStructureCalculator.hpp:54
CDPL_CHEM_API void kekulizeBonds(MolecularGraph &molgraph)
MolecularGraph.
Definition: MolecularGraph.hpp:52
Definition of the type CDPL::Util::BitSet.
Sets the protation state of molecules according to desired objectives.
Definition: ProtonationStateStandardizer.hpp:57
Molecule.
Definition: Molecule.hpp:49
ChEMBLStandardizer & operator=(const ChEMBLStandardizer &standardizer)
CDPL_CHEM_API void clearMatchConstraints(Atom &atom)
ChEMBLStandardizer(const ChEMBLStandardizer &standardizer)
Definition of the class CDPL::Chem::Fragment.
std::shared_ptr< ChEMBLStandardizer > SharedPointer
Definition: ChEMBLStandardizer.hpp:68
const unsigned int NONE
Represents an empty set of atom properties.
Definition: Biomol/AtomPropertyFlag.hpp:48
The namespace of the Chemical Data Processing Library.
Array< std::size_t > STArray
An array of unsigned integers of type std::size_t.
Definition: Array.hpp:567
ChangeFlags standardize(const Molecule &mol, Molecule &std_mol, bool proc_excluded=false)
ChangeFlags standardize(Molecule &mol, bool proc_excld=false)
Definition of the class CDPL::Chem::SubstructureSearch.
HashCodeCalculator.
Definition: HashCodeCalculator.hpp:57
VectorArray< Vector2D > Vector2DArray
An array of Math::Vector2D objects.
Definition: VectorArray.hpp:79
Definition of the class CDPL::Chem::ProtonationStateStandardizer.
Definition of the class CDPL::Chem::KekuleStructureCalculator.
Definition of the class CDPL::Chem::BasicMolecule.