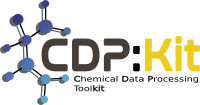 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CONFGEN_CANONICALFRAGMENT_HPP
30 #define CDPL_CONFGEN_CANONICALFRAGMENT_HPP
138 bool modify =
true,
bool strip_aro_subst =
true);
146 bool modify,
bool strip_aro_subst);
156 typedef std::vector<std::uint32_t> HashInputData;
160 std::uint64_t hashCode;
162 SmallestSetOfSmallestRingsPtr sssr;
164 HashInputData hashInputData;
170 #endif // CDPL_CONFGEN_CANONICALFRAGMENT_HPP
std::shared_ptr< MolecularGraph > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated MolecularGraph instances.
Definition: MolecularGraph.hpp:58
const Chem::Atom & getAtom(std::size_t idx) const
Returns a const reference to the Chem::Atom instance at index idx.
BondIterator getBondsBegin()
std::function< bool(const Chem::Atom &, const Chem::Atom &)> AtomCompareFunction
A generic wrapper class used to store a user-defined atom compare function.
Definition: AtomCompareFunction.hpp:41
void orderAtoms(const Chem::AtomCompareFunction &func)
Orders the stored atoms according to criteria implemented by the provided atom comparison function.
std::vector< const Chem::Atom * > AtomMapping
Definition: CanonicalFragment.hpp:67
Bond.
Definition: Bond.hpp:50
Chem::BasicMolecule::ConstAtomIterator ConstAtomIterator
Definition: CanonicalFragment.hpp:63
CDPL_CHEM_API std::uint64_t calcHashCode(const MolecularGraph &molgraph, unsigned int atom_flags=AtomPropertyFlag::DEFAULT, unsigned int bond_flags=BondPropertyFlag::DEFAULT, bool ord_h_deplete=true)
std::size_t getNumBonds() const
Returns the number of stored Chem::Bond objects.
BasicMolecule.
Definition: BasicMolecule.hpp:54
ConstAtomIterator getAtomsBegin() const
Chem::BasicMolecule::BondIterator BondIterator
Definition: CanonicalFragment.hpp:64
A STL compatible random access iterator for container elements accessible by index.
Definition: IndexedElementIterator.hpp:125
Atom.
Definition: Atom.hpp:52
bool containsAtom(const Chem::Atom &atom) const
Tells whether the specified Chem::Atom instance is stored in this container.
CanonicalFragment(const CanonicalFragment &frag)
const AtomMapping & getAtomMapping() const
Chem::Entity3D & getEntity(std::size_t idx)
Returns a non-const reference to the entity at index idx.
ConstBondIterator getBondsEnd() const
Definition of the class CDPL::Chem::CanonicalNumberingCalculator.
BondIterator getBondsEnd()
std::size_t getAtomIndex(const Chem::Atom &atom) const
Returns the index of the specified Chem::Atom instance in this container.
MolecularGraph.
Definition: MolecularGraph.hpp:52
void create(const Chem::MolecularGraph &molgraph, const Chem::MolecularGraph &parent, bool modify=true, bool strip_aro_subst=true)
Definition of the class CDPL::Util::Array.
std::uint64_t getHashCode() const
Chem::MolecularGraph::SharedPointer clone() const
Creates a copy of the molecular graph.
CanonicalFragment.
Definition: CanonicalFragment.hpp:54
Chem::Atom & getAtom(std::size_t idx)
Returns a non-const reference to the atom at index idx.
Definition of the preprocessor macro CDPL_CONFGEN_API.
std::function< bool(const Chem::Bond &, const Chem::Bond &)> BondCompareFunction
A generic wrapper class used to store a user-defined bond compare function.
Definition: BondCompareFunction.hpp:41
std::shared_ptr< CanonicalFragment > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated CanonicalFragment instances.
Definition: CanonicalFragment.hpp:60
boost::indirect_iterator< BondList::const_iterator, const BasicBond > ConstBondIterator
Definition: BasicMolecule.hpp:72
AtomIterator getAtomsEnd()
boost::indirect_iterator< BondList::iterator, BasicBond > BondIterator
Definition: BasicMolecule.hpp:71
#define CDPL_CONFGEN_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
boost::indirect_iterator< AtomList::iterator, BasicAtom > AtomIterator
Definition: BasicMolecule.hpp:69
Definition of the class CDPL::Chem::SmallestSetOfSmallestRings.
Chem::BasicMolecule::AtomIterator AtomIterator
Definition: CanonicalFragment.hpp:62
Chem::Bond & getBond(std::size_t idx)
Returns a non-const reference to the bond at index idx.
const Chem::Entity3D & getEntity(std::size_t idx) const
Returns a const reference to the Chem::Entity3D instance at index idx.
std::shared_ptr< SmallestSetOfSmallestRings > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated SmallestSetOfSmallestRings instan...
Definition: SmallestSetOfSmallestRings.hpp:64
AtomIterator getAtomsBegin()
CanonicalNumberingCalculator.
Definition: CanonicalNumberingCalculator.hpp:67
CanonicalFragment()
Constructs an empty CanonicalFragment instance.
std::size_t getBondIndex(const Chem::Bond &bond) const
Returns the index of the specified Chem::Bond instance in this container.
The namespace of the Chemical Data Processing Library.
Array< std::size_t > STArray
An array of unsigned integers of type std::size_t.
Definition: Array.hpp:567
CanonicalFragment(const Chem::MolecularGraph &molgraph, const Chem::MolecularGraph &parent)
Constructs a CanonicalFragment instance that contains the relevant atoms and bonds of the molecular g...
std::size_t getNumEntities() const
Returns the number of stored Chem::Entity3D objects.
Chem::BasicMolecule::ConstBondIterator ConstBondIterator
Definition: CanonicalFragment.hpp:65
ConstAtomIterator getAtomsEnd() const
CanonicalFragment & operator=(const CanonicalFragment &frag)
std::size_t getNumAtoms() const
Returns the number of stored Chem::Atom objects.
boost::indirect_iterator< AtomList::const_iterator, const BasicAtom > ConstAtomIterator
Definition: BasicMolecule.hpp:70
void orderBonds(const Chem::BondCompareFunction &func)
Orders the stored bonds according to criteria implemented by the provided bond comparison function.
Entity3D.
Definition: Entity3D.hpp:46
bool containsBond(const Chem::Bond &bond) const
Tells whether the specified Chem::Bond instance is stored in this container.
CDPL_CHEM_API void canonicalize(MolecularGraph &molgraph, const AtomCompareFunction &func, bool atoms=true, bool atom_nbrs=true, bool bonds=true, bool bond_atoms=false)
Definition of the class CDPL::Chem::BasicMolecule.
const Chem::Bond & getBond(std::size_t idx) const
Returns a const reference to the Chem::Bond instance at index idx.
ConstBondIterator getBondsBegin() const