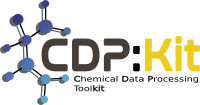 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_VIS_CAIROPOINTER_HPP
30 #define CDPL_VIS_CAIROPOINTER_HPP
134 template <
typename T>
179 bool operator!() const throw();
186 T& operator*() const throw();
196 T* operator->() const throw();
232 template <typename
T>
237 template <
typename T>
242 template <
typename T>
248 template <
typename T>
251 if (
this != &other) {
259 template <
typename T>
265 template <
typename T>
271 template <
typename T>
277 template <
typename T>
283 template <
typename T>
291 template <
typename T>
300 #endif // CDPL_VIS_CAIROPOINTER_HPP
bool operator!() const
Tells whether this CairoPointer references an object or holds a null pointer.
Definition: CairoPointer.hpp:260
const unsigned int p
Specifies that the stereocenter has p configuration.
Definition: CIPDescriptor.hpp:121
struct _cairo_pattern cairo_pattern_t
Definition: CairoPointer.hpp:37
CairoPointer & operator=(const CairoPointer &ptr)
Assignment operator.
Definition: CairoPointer.hpp:249
static cairo_pattern_t * reference(cairo_pattern_t *p)
Increments the reference count of the object pointed to by p by 1.
T * operator->() const
Returns a pointer to the referenced object.
Definition: CairoPointer.hpp:272
static void destroy(cairo_surface_t *p)
Decrements the reference count of the object pointed to by p by 1.
struct _cairo_surface cairo_surface_t
Definition: CairoPointer.hpp:36
static void destroy(cairo_t *p)
Decrements the reference count of the object pointed to by p by 1.
struct _cairo cairo_t
Definition: CairoPointer.hpp:35
~CairoPointer()
Destroys the CairoPointer.
Definition: CairoPointer.hpp:243
#define CDPL_VIS_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
static cairo_surface_t * reference(cairo_surface_t *p)
Increments the reference count of the object pointed to by p by 1.
T & operator*() const
Returns a non-const reference to the pointed-to object.
Definition: CairoPointer.hpp:266
static cairo_t * reference(cairo_t *p)
Increments the reference count of the object pointed to by p by 1.
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
The namespace of the Chemical Data Processing Library.
T * get() const
Returns a pointer to the referenced object.
Definition: CairoPointer.hpp:278
static void destroy(cairo_pattern_t *p)
Decrements the reference count of the object pointed to by p by 1.
T * release()
Releases the currently referenced object.
Definition: CairoPointer.hpp:284
Definition: CairoPointer.hpp:47
Definition of the preprocessor macro CDPL_VIS_API.
void reset(T *ptr=0)
Replaces the current object reference with a reference to the object pointed to by ptr.
Definition: CairoPointer.hpp:292
A smart pointer managing the lifetime of allocated Cairo data structures.
Definition: CairoPointer.hpp:136
CairoPointer(T *ptr=0)
Constructs a CairoPointer that manages the reference count of the object pointed to by ptr.
Definition: CairoPointer.hpp:233