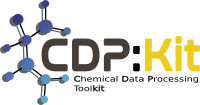 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_BONDORDERCALCULATOR_HPP
30 #define CDPL_CHEM_BONDORDERCALCULATOR_HPP
49 class SubstructureSearch;
54 class AtomBondMapping;
113 typedef std::shared_ptr<MolecularGraph> MolecularGraphPtr;
114 typedef std::shared_ptr<SubstructureSearch> SubstructureSearchPtr;
115 typedef std::vector<const Bond*> BondList;
116 typedef std::vector<const Atom*> AtomList;
117 typedef std::vector<std::size_t> UIntTable;
118 typedef std::vector<Geometry> GeometryTable;
119 typedef std::vector<MolecularGraphPtr> MolecularGraphPtrList;
120 typedef std::vector<std::pair<double, const AtomBondMapping*> > ABMappingList;
122 class AtomMatchExpression;
123 class BondMatchExpression;
125 friend class BondMatchExpression;
139 void assignFragBondOrders(std::size_t depth,
Util::STArray& orders);
141 double calcHybridizationMatchScore();
146 Geometry perceiveInitialGeometry(
const Atom& atom);
147 void fixRingAtomGeometries(
const Fragment& ring);
152 void getNeighborAtoms(
const Atom& atom, AtomList& atom_list,
const Atom* exclude_atom)
const;
153 std::size_t countBondsWithOrder(
const Atom& atom, std::size_t order,
const UIntTable& order_table)
const;
155 template <
typename Pred>
156 void getUndefBondFragment(
const Atom& atom,
bool add_atoms,
const Pred& bond_pred);
158 double calcAvgBondAngle(
const Atom& atom,
const AtomList& nbr_atoms)
const;
159 double calcDihedralAngle(
const Atom& atom1,
const Atom& atom2,
const Atom& atom3,
const Atom& atom4)
const;
162 double calcAvgTorsionAngle(
const Fragment& ring)
const;
164 bool isPlanarPiBond(
const Bond& bond)
const;
170 UIntTable freeAtomValences;
171 GeometryTable atomGeometries;
173 BondList fragBondList;
174 AtomList fragAtomList;
175 AtomList nbrAtomList1;
176 AtomList nbrAtomList2;
177 UIntTable fragBondOrders;
178 UIntTable workingBondOrders;
179 double currOrderAssmentScore;
180 double bestOrderAssmentScore;
181 SubstructureSearchPtr substructSearch;
182 MolecularGraphPtrList funcGroupPatterns;
183 ABMappingList funcGroupMappings;
192 #endif // CDPL_CHEM_BONDORDERCALCULATOR_HPP
Definition of the preprocessor macro CDPL_CHEM_API.
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Bond.
Definition: Bond.hpp:50
void calculate(const MolecularGraph &molgraph, Util::STArray &orders)
Perceives the order of the bonds in the molecular graph molgraph from its 3D structure and atom conne...
CVector< double, 3 > Vector3D
A bounded 3 element vector holding floating point values of type double.
Definition: Vector.hpp:1637
BondOrderCalculator()
Constructs the BondOrderCalculator instance.
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
Atom.
Definition: Atom.hpp:52
Fragment.
Definition: Fragment.hpp:52
MolecularGraph.
Definition: MolecularGraph.hpp:52
Definition of the type CDPL::Util::BitSet.
Definition of the class CDPL::Util::Array.
BondOrderCalculator.
Definition: BondOrderCalculator.hpp:60
bool undefinedOnly() const
Tells whether or not only undefined bond orders have to be perceived.
The namespace of the Chemical Data Processing Library.
Array< std::size_t > STArray
An array of unsigned integers of type std::size_t.
Definition: Array.hpp:567
const unsigned int LINEAR
Specifies linear geometry.
Definition: CoordinationGeometry.hpp:58
A data structure for the common storage of related atom to atom and bond to bond mappings.
Definition: AtomBondMapping.hpp:55
void undefinedOnly(bool undef_only)
Allows to specify whether already defined bond orders should be left unchanged.
const unsigned int TETRAHEDRAL
Specifies tetrahedral geometry.
Definition: CoordinationGeometry.hpp:68
Definition of vector data types.
BondOrderCalculator(const MolecularGraph &molgraph, Util::STArray &orders, bool undef_only=true)
Constructs the BondOrderCalculator instance and perceives the order of the bonds in the molecular gra...