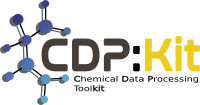 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_BIOMOL_UTILITYFUNCTIONS_HPP
30 #define CDPL_BIOMOL_UTILITYFUNCTIONS_HPP
48 template <
typename Iter>
50 char ins_code = 0, std::size_t model_no = 0,
const char* atom_name = 0,
long serial_no =
IGNORE_SERIAL_NO)
52 return std::find_if(it, end,
53 std::bind(
static_cast<bool (*)(
const Chem::Atom&,
const char*,
const char*,
long,
char, std::size_t,
const char*,
long)
>(&
matchesResidueInfo), std::placeholders::_1, res_code, chain_id, res_seq_no,
54 ins_code, model_no, atom_name, serial_no));
57 template <
typename Iter>
59 char ins_code = 0, std::size_t model_no = 0,
const char* atom_name = 0,
long serial_no =
IGNORE_SERIAL_NO)
61 for (; it != end; ++it) {
71 std::bind(
static_cast<bool (*)(
const Chem::Atom&,
const char*,
const char*,
long,
char, std::size_t,
const char*,
long)
>(&
matchesResidueInfo), std::placeholders::_1, res_code, chain_id, res_seq_no,
72 ins_code, model_no, atom_name, serial_no)) != res.
getAtomsEnd())
81 #endif // CDPL_BIOMOL_UTILITYFUNCTIONS_HPP
CDPL_BIOMOL_API bool matchesResidueInfo(const Chem::Atom &atom, const char *res_code=0, const char *chain_id=0, long res_seq_no=IGNORE_SEQUENCE_NO, char ins_code=0, std::size_t model_no=0, const char *atom_name=0, long serial_no=IGNORE_SERIAL_NO)
Atom.
Definition: Atom.hpp:52
Declaration of functions that operate on Chem::Atom instances.
Definition of flags for serial and residue sequence number processing.
ConstAtomIterator getAtomsBegin() const
Returns a constant iterator pointing to the beginning of the stored const Chem::Atom objects.
MolecularGraph.
Definition: MolecularGraph.hpp:52
Iter findResidue(Iter it, Iter end, const char *res_code=0, const char *chain_id=0, long res_seq_no=IGNORE_SEQUENCE_NO, char ins_code=0, std::size_t model_no=0, const char *atom_name=0, long serial_no=IGNORE_SERIAL_NO)
Definition: Biomol/UtilityFunctions.hpp:58
const long IGNORE_SEQUENCE_NO
Definition: ProcessingFlags.hpp:41
Definition of the class CDPL::Chem::MolecularGraph.
Definition of the class CDPL::Chem::Atom.
Declaration of functions that operate on Chem::MolecularGraph instances.
const long IGNORE_SERIAL_NO
Definition: ProcessingFlags.hpp:42
The namespace of the Chemical Data Processing Library.
Iter findResidueAtom(Iter it, Iter end, const char *res_code=0, const char *chain_id=0, long res_seq_no=IGNORE_SEQUENCE_NO, char ins_code=0, std::size_t model_no=0, const char *atom_name=0, long serial_no=IGNORE_SERIAL_NO)
Definition: Biomol/UtilityFunctions.hpp:49
ConstAtomIterator getAtomsEnd() const
Returns a constant iterator pointing to the end of the stored const Chem::Atom objects.