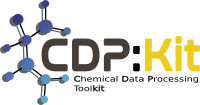 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_BIOMOL_MOLECULARGRAPHFUNCTIONS_HPP
30 #define CDPL_BIOMOL_MOLECULARGRAPHFUNCTIONS_HPP
115 double max_dist,
bool inc_core_atoms =
false,
bool append =
false);
121 double max_dist,
bool append =
false);
129 char ins_code = 0, std::size_t model_no = 0);
133 #endif // CDPL_BIOMOL_MOLECULARGRAPHFUNCTIONS_HPP
CDPL_BIOMOL_API void setResidueCode(Chem::Atom &atom, const std::string &code)
CDPL_BIOMOL_API void clearResidueSequenceNumber(Chem::Atom &atom)
const unsigned int DEFAULT
Represents the default set of atom properties.
Definition: Biomol/AtomPropertyFlag.hpp:53
CDPL_BIOMOL_API bool matchesResidueInfo(const Chem::Atom &atom, const char *res_code=0, const char *chain_id=0, long res_seq_no=IGNORE_SEQUENCE_NO, char ins_code=0, std::size_t model_no=0, const char *atom_name=0, long serial_no=IGNORE_SERIAL_NO)
CDPL_BIOMOL_API void clearChainID(Chem::Atom &atom)
CDPL_BIOMOL_API long getResidueSequenceNumber(const Chem::Atom &atom)
CDPL_BIOMOL_API void extractProximalAtoms(const Chem::MolecularGraph &core, const Chem::MolecularGraph ¯omol, Chem::Fragment &env_atoms, double max_dist, bool inc_core_atoms=false, bool append=false)
CDPL_BIOMOL_API void clearModelNumber(Chem::Atom &atom)
CDPL_BIOMOL_API void setHydrogenResidueSequenceInfo(Chem::MolecularGraph &molgraph, bool overwrite, unsigned int flags=AtomPropertyFlag::DEFAULT)
CDPL_BIOMOL_API void clearResidueInsertionCode(Chem::Atom &atom)
Definition of the class CDPL::Biomol::PDBData.
CDPL_BIOMOL_API void setResidueSequenceNumber(Chem::Atom &atom, long seq_no)
CDPL_BIOMOL_API bool hasChainID(const Chem::Atom &atom)
Fragment.
Definition: Fragment.hpp:52
CDPL_BIOMOL_API bool hasResidueCode(const Chem::Atom &atom)
CDPL_BIOMOL_API bool hasModelNumber(const Chem::Atom &atom)
CDPL_BIOMOL_API void convertMOL2ToPDBResidueInfo(Chem::MolecularGraph &molgraph, bool overwrite)
Definition of flags for serial and residue sequence number processing.
Definition of constants in namespace CDPL::Biomol::AtomPropertyFlag.
CDPL_BIOMOL_API void setModelNumber(Chem::Atom &atom, std::size_t model_no)
CDPL_BIOMOL_API bool hasResidueInsertionCode(const Chem::Atom &atom)
CDPL_BIOMOL_API bool hasPDBData(const Chem::MolecularGraph &molgraph)
CDPL_BIOMOL_API bool hasResidueSequenceNumber(const Chem::Atom &atom)
MolecularGraph.
Definition: MolecularGraph.hpp:52
Type definition of a generic wrapper class for storing user-defined Chem::Atom 3D-coordinates functio...
CDPL_BIOMOL_API void extractResidueSubstructures(const Chem::MolecularGraph &molgraph, const Chem::MolecularGraph &parent_molgraph, Chem::Fragment &res_substructs, bool cnctd_only=false, unsigned int flags=AtomPropertyFlag::DEFAULT, bool append=false)
const long IGNORE_SEQUENCE_NO
Definition: ProcessingFlags.hpp:41
CDPL_BIOMOL_API const std::string & getResidueCode(const Chem::Atom &atom)
CDPL_BIOMOL_API char getResidueInsertionCode(const Chem::Atom &atom)
CDPL_BIOMOL_API const std::string & getChainID(const Chem::Atom &atom)
CDPL_BIOMOL_API std::size_t getModelNumber(const Chem::Atom &atom)
CDPL_BIOMOL_API void setPDBData(Chem::MolecularGraph &molgraph, const PDBData::SharedPointer &data)
CDPL_BIOMOL_API void clearPDBData(Chem::MolecularGraph &molgraph)
std::function< const Math::Vector3D &(const Chem::Atom &)> Atom3DCoordinatesFunction
A generic wrapper class used to store a user-defined Chem::Atom 3D-coordinates function.
Definition: Atom3DCoordinatesFunction.hpp:43
Definition of the preprocessor macro CDPL_BIOMOL_API.
The namespace of the Chemical Data Processing Library.
CDPL_BIOMOL_API const PDBData::SharedPointer & getPDBData(const Chem::MolecularGraph &molgraph)
CDPL_BIOMOL_API void extractEnvironmentResidues(const Chem::MolecularGraph &core, const Chem::MolecularGraph ¯omol, Chem::Fragment &env_residues, double max_dist, bool append=false)
CDPL_BIOMOL_API void setChainID(Chem::Atom &atom, const std::string &id)
CDPL_BIOMOL_API void setResidueInsertionCode(Chem::Atom &atom, char code)
#define CDPL_BIOMOL_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
std::shared_ptr< PDBData > SharedPointer
Definition: PDBData.hpp:53
CDPL_BIOMOL_API void clearResidueCode(Chem::Atom &atom)