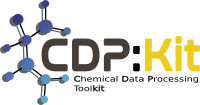 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_BASICMOLECULE_HPP
30 #define CDPL_CHEM_BASICMOLECULE_HPP
35 #include <boost/iterator/indirect_iterator.hpp>
58 typedef AtomCache::SharedObjectPointer AtomPtr;
59 typedef BondCache::SharedObjectPointer BondPtr;
60 typedef std::vector<AtomPtr> AtomList;
61 typedef std::vector<BondPtr> BondList;
69 typedef boost::indirect_iterator<AtomList::iterator, BasicAtom>
AtomIterator;
70 typedef boost::indirect_iterator<AtomList::const_iterator, const BasicAtom>
ConstAtomIterator;
71 typedef boost::indirect_iterator<BondList::iterator, BasicBond>
BondIterator;
72 typedef boost::indirect_iterator<BondList::const_iterator, const BasicBond>
ConstBondIterator;
220 using Molecule::operator=;
233 using Molecule::operator+=;
267 template <
typename T>
268 void doCopy(
const T& mol);
270 template <
typename T>
271 void doAppend(
const T& mol);
273 void clearAtomsAndBonds();
275 void renumberAtoms(std::size_t idx);
276 void renumberBonds(std::size_t idx);
281 static void destroyAtom(
BasicAtom* atom);
282 static void destroyBond(
BasicBond* bond);
295 #endif // CDPL_CHEM_BASICMOLECULE_HPP
std::shared_ptr< MolecularGraph > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated MolecularGraph instances.
Definition: MolecularGraph.hpp:58
Definition of the class CDPL::Chem::Molecule.
BasicAtom & addAtom()
Creates a new atom and adds it to the molecule.
BasicAtom.
Definition: BasicAtom.hpp:54
Definition of the class CDPL::Util::ObjectPool.
std::size_t getAtomIndex(const Atom &atom) const
Returns the index of the specified atom.
std::function< bool(const Chem::Atom &, const Chem::Atom &)> AtomCompareFunction
A generic wrapper class used to store a user-defined atom compare function.
Definition: AtomCompareFunction.hpp:41
bool containsAtom(const Atom &atom) const
Tells whether the specified atom is part of this molecule.
ConstBondIterator getBondsEnd() const
Returns a constant iterator pointing to the end of the bonds.
BasicMolecule & operator+=(const BasicMolecule &mol)
Extends the current set of atoms and bonds by a copy of the atoms and bonds in the molecule mol.
ConstBondIterator getBondsBegin() const
Returns a constant iterator pointing to the beginning of the bonds.
Definition of the preprocessor macro CDPL_CHEM_API.
void reserveMemoryForBonds(std::size_t num_bonds)
Reserves memory for num_bonds bonds.
const BasicAtom & getAtom(std::size_t idx) const
Returns a const reference to the atom at index idx.
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Bond.
Definition: Bond.hpp:50
AtomIterator getAtomsEnd()
Returns a mutable iterator pointing to the end of the atoms.
void append(const MolecularGraph &molgraph)
Extends the current set of atoms and bonds by a copy of the atoms and bonds in the molecular graph mo...
std::shared_ptr< BasicMolecule > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated BasicMolecule instances.
Definition: BasicMolecule.hpp:67
BasicMolecule.
Definition: BasicMolecule.hpp:54
BasicMolecule(const MolecularGraph &molgraph)
Constructs a BasicMolecule instance with copies of the atoms and bonds of the Chem::MolecularGraph in...
void append(const BasicMolecule &mol)
Extends the current set of atoms and bonds by a copy of the atoms and bonds in the molecule mol.
AtomIterator getAtomsBegin()
Returns a mutable iterator pointing to the beginning of the atoms.
A STL compatible random access iterator for container elements accessible by index.
Definition: IndexedElementIterator.hpp:125
Atom.
Definition: Atom.hpp:52
void clear()
Removes all atoms and bonds and clears all properties of the molecule.
BondIterator getBondsEnd()
Returns a mutable iterator pointing to the end of the bonds.
void copy(const Molecule &mol)
Replaces the current set of atoms, bonds and properties by a copy of the atoms, bonds and properties ...
BasicBond.
Definition: BasicBond.hpp:49
MolecularGraph::SharedPointer clone() const
Creates a copy of the molecular graph.
BasicBond & addBond(std::size_t atom1_idx, std::size_t atom2_idx)
Creates a new or returns an already existing bond between the atoms specified by atom1_idx and atom2_...
bool containsBond(const Bond &bond) const
Tells whether the specified bond is part of this molecule.
std::size_t getBondIndex(const Bond &bond) const
Returns the index of the specified bond.
MolecularGraph.
Definition: MolecularGraph.hpp:52
Molecule.
Definition: Molecule.hpp:49
BasicMolecule & operator=(const BasicMolecule &mol)
Replaces the current set of atoms, bonds and properties by a copy of the atoms, bonds and properties ...
ConstAtomIterator getAtomsEnd() const
Returns a constant iterator pointing to the end of the atoms.
void remove(const MolecularGraph &molgraph)
Removes atoms and bonds referenced by the molecular graph molgraph that are part of this Molecule ins...
Definition of the class CDPL::Chem::BasicAtom.
~BasicMolecule()
Destructor.
const BasicBond & getBond(std::size_t idx) const
Returns a const reference to the bond at index idx.
void removeAtom(std::size_t idx)
Removes the atom at the specified index.
BasicMolecule(const Molecule &mol)
Constructs a copy of the Chem::Molecule instance mol.
std::function< bool(const Chem::Bond &, const Chem::Bond &)> BondCompareFunction
A generic wrapper class used to store a user-defined bond compare function.
Definition: BondCompareFunction.hpp:41
ConstAtomIterator getAtomsBegin() const
Returns a constant iterator pointing to the beginning of the atoms.
boost::indirect_iterator< BondList::const_iterator, const BasicBond > ConstBondIterator
Definition: BasicMolecule.hpp:72
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
boost::indirect_iterator< BondList::iterator, BasicBond > BondIterator
Definition: BasicMolecule.hpp:71
boost::indirect_iterator< AtomList::iterator, BasicAtom > AtomIterator
Definition: BasicMolecule.hpp:69
void copy(const MolecularGraph &molgraph)
Replaces the current set of atoms, bonds and properties by a copy of the atoms, bonds and properties ...
void orderBonds(const BondCompareFunction &func)
Orders the stored bonds according to criteria implemented by the provided bond comparison function.
void reserveMemoryForAtoms(std::size_t num_atoms)
Reserves memory for num_atoms atoms.
BondIterator getBondsBegin()
Returns a mutable iterator pointing to the beginning of the bonds.
The namespace of the Chemical Data Processing Library.
void append(const Molecule &mol)
Extends the current set of atoms and bonds by a copy of the atoms and bonds in the molecule mol.
BasicMolecule()
Constructs an empty BasicMolecule instance.
BasicBond & getBond(std::size_t idx)
Returns a non-const reference to the bond at index idx.
boost::indirect_iterator< AtomList::const_iterator, const BasicAtom > ConstAtomIterator
Definition: BasicMolecule.hpp:70
BasicMolecule(const BasicMolecule &mol)
Constructs a copy of the BasicMolecule instance mol.
void orderAtoms(const AtomCompareFunction &func)
Orders the stored atoms according to criteria implemented by the provided atom comparison function.
BondIterator removeBond(const BondIterator &it)
Removes the bond specified by the iterator it.
AtomIterator removeAtom(const AtomIterator &it)
Removes the atom specified by the iterator it.
BasicAtom & getAtom(std::size_t idx)
Returns a non-const reference to the atom at index idx.
void removeBond(std::size_t idx)
Removes the bond at the specified index.
std::size_t getNumAtoms() const
Returns the number of explicit atoms.
std::size_t getNumBonds() const
Returns the number of explicit bonds.
Definition of the class CDPL::Chem::BasicBond.
void copy(const BasicMolecule &mol)
Replaces the current set of atoms, bonds and properties by a copy of the atoms, bonds and properties ...