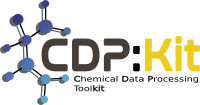 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_BASICATOM_HPP
30 #define CDPL_CHEM_BASICATOM_HPP
35 #include <boost/iterator/transform_iterator.hpp>
58 typedef std::pair<BasicAtom*, BasicBond*> AtomBondPair;
59 typedef std::vector<AtomBondPair> NeighborList;
61 template <
typename AtomType>
65 typedef AtomType& result_type;
67 AtomType& operator()(
const AtomBondPair& ab_pair)
const
69 return *ab_pair.first;
73 template <
typename BondType>
77 typedef BondType& result_type;
79 BondType& operator()(
const AtomBondPair& ab_pair)
const
81 return *ab_pair.second;
86 typedef boost::transform_iterator<AtomAccessor<BasicAtom>, NeighborList::iterator>
AtomIterator;
87 typedef boost::transform_iterator<AtomAccessor<const BasicAtom>, NeighborList::const_iterator>
ConstAtomIterator;
88 typedef boost::transform_iterator<BondAccessor<BasicBond>, NeighborList::iterator>
BondIterator;
89 typedef boost::transform_iterator<BondAccessor<const BasicBond>, NeighborList::const_iterator>
ConstBondIterator;
184 using Atom::operator=;
193 void setIndex(std::size_t idx);
195 void clearAdjacencyLists();
199 static void disconnectAtoms(
BasicBond& bond);
203 NeighborList neighbors;
208 #endif // CDPL_CHEM_BASICATOM_HPP
boost::transform_iterator< BondAccessor< const BasicBond >, NeighborList::const_iterator > ConstBondIterator
Definition: BasicAtom.hpp:89
BasicAtom.
Definition: BasicAtom.hpp:54
std::function< bool(const Chem::Atom &, const Chem::Atom &)> AtomCompareFunction
A generic wrapper class used to store a user-defined atom compare function.
Definition: AtomCompareFunction.hpp:41
const Molecule & getMolecule() const
Returns a const reference to the parent molecule.
Definition of the preprocessor macro CDPL_CHEM_API.
Bond & getBondToAtom(const Atom &atom)
Returns a non-const reference to the Chem::Bond object that connects this atom to the argument atom.
ConstBondIterator getBondsEnd() const
Returns a constant iterator pointing to the end of the incident bonds.
BondIterator getBondsEnd()
Returns a mutable iterator pointing to the end of the incident bonds.
Bond * findBondToAtom(const Atom &atom)
Returns a pointer to the non-const Chem::Bond object that connects this atom to the argument atom.
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Bond.
Definition: Bond.hpp:50
std::size_t getIndex() const
Returns the index of the atom in its parent molecule.
const Bond & getBond(std::size_t idx) const
Returns a const reference to the incident bond at index idx.
BondIterator getBondsBegin()
Returns a mutable iterator pointing to the beginning of the incident bonds.
BasicMolecule.
Definition: BasicMolecule.hpp:54
std::size_t getBondIndex(const Bond &bond) const
Returns the index of the specified incident bond.
A STL compatible random access iterator for container elements accessible by index.
Definition: IndexedElementIterator.hpp:125
Atom.
Definition: Atom.hpp:52
boost::transform_iterator< BondAccessor< BasicBond >, NeighborList::iterator > BondIterator
Definition: BasicAtom.hpp:88
ConstAtomIterator getAtomsEnd() const
Returns a constant iterator pointing to the end of the connected atoms.
BasicBond.
Definition: BasicBond.hpp:49
boost::transform_iterator< AtomAccessor< const BasicAtom >, NeighborList::const_iterator > ConstAtomIterator
Definition: BasicAtom.hpp:87
CDPL_CHEM_API void connectAtoms(Molecule &mol, double dist_tol=0.3, std::size_t atom_idx_offs=0)
const Bond & getBondToAtom(const Atom &atom) const
Returns a const reference to the Chem::Bond object that connects this atom to the argument atom.
bool containsAtom(const Atom &atom) const
Tells whether this atom and the argument atom are connected by a bond.
Molecule.
Definition: Molecule.hpp:49
void orderBonds(const BondCompareFunction &func)
Orders the stored bonds according to criteria implemented by the provided bond comparison function.
Atom & getAtom(std::size_t idx)
Returns a non-const reference to the connected atom at index idx.
const Bond * findBondToAtom(const Atom &atom) const
Returns a pointer to the const Chem::Bond object that connects this atom to the argument atom.
std::size_t getNumBonds() const
Returns the number of incident bonds.
std::function< bool(const Chem::Bond &, const Chem::Bond &)> BondCompareFunction
A generic wrapper class used to store a user-defined bond compare function.
Definition: BondCompareFunction.hpp:41
Definition of the class CDPL::Chem::Atom.
std::size_t getAtomIndex(const Atom &atom) const
Returns the index of the specified connected atom.
AtomIterator getAtomsEnd()
Returns a mutable iterator pointing to the end of the connected atoms.
Molecule & getMolecule()
Returns a non-const reference to the parent molecule.
ConstBondIterator getBondsBegin() const
Returns a constant iterator pointing to the beginning of the incident bonds.
bool containsBond(const Bond &bond) const
Tells whether the specified bond is incident to this atom.
The namespace of the Chemical Data Processing Library.
AtomIterator getAtomsBegin()
ConstAtomIterator getAtomsBegin() const
boost::transform_iterator< AtomAccessor< BasicAtom >, NeighborList::iterator > AtomIterator
Definition: BasicAtom.hpp:86
std::size_t getNumAtoms() const
Returns the number of connected atoms.
Bond & getBond(std::size_t idx)
Returns a non-const reference to the incident bond at index idx.
BasicAtom & operator=(const BasicAtom &atom)
Assignment operator that replaces the current set of properties with the properties of atom;.
void orderAtoms(const AtomCompareFunction &func)
Orders the stored atoms according to criteria implemented by the provided atom comparison function.
const Atom & getAtom(std::size_t idx) const
Returns a const reference to the connected atom at index idx.