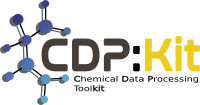 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_BASE_EXCEPTIONS_HPP
30 #define CDPL_BASE_EXCEPTIONS_HPP
66 const
char* what() const throw();
267 #endif // CDPL_BASE_EXCEPTIONS_HPP
virtual ~CalculationFailed()
Virtual destructor.
ValueError(const std::string &msg="")
Constructs a ValueError object with the error message set to msg.
virtual ~RangeError()
Virtual destructor.
virtual ~SizeError()
Virtual destructor.
The root of the CDPL exception hierarchy.
Definition: Base/Exceptions.hpp:48
Thrown to indicate that some requested operation has failed (e.g. due to unfulfilled preconditions or...
Definition: Base/Exceptions.hpp:211
Thrown to indicate that a value is out of range.
Definition: Base/Exceptions.hpp:114
virtual ~IOError()
Virtual destructor.
Thrown to indicate that an I/O operation has failed because of physical (e.g. broken pipe) or logical...
Definition: Base/Exceptions.hpp:250
#define CDPL_BASE_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Thrown to indicate that some requested data item could not be found.
Definition: Base/Exceptions.hpp:171
NullPointerException(const std::string &msg="")
Constructs a NullPointerException object with the error message set to msg.
virtual ~ItemNotFound()
Virtual destructor.
BadCast(const std::string &msg="")
Constructs a BadCast object with the error message set to msg.
IOError(const std::string &msg="")
Constructs an IOError object with the error message set to msg.
Definition of the preprocessor macro CDPL_BASE_API.
Thrown to indicate that an index is out of range.
Definition: Base/Exceptions.hpp:152
Thrown to indicate that a cast or conversion from a given source type to a requested target type is n...
Definition: Base/Exceptions.hpp:191
Thrown to indicate that the size of a (multidimensional) array is not correct.
Definition: Base/Exceptions.hpp:133
SizeError(const std::string &msg="")
Constructs a SizeError object with the error message set to msg.
Thrown to indicate errors caused by some invalid value.
Definition: Base/Exceptions.hpp:76
virtual ~ValueError()
Virtual destructor.
Thrown to indicate that some requested calculation has failed.
Definition: Base/Exceptions.hpp:230
ItemNotFound(const std::string &msg="")
Constructs an ItemNotFound object with the error message set to msg.
virtual ~NullPointerException()
Virtual destructor.
RangeError(const std::string &msg="")
Constructs a RangeError object with the error message set to msg.
The namespace of the Chemical Data Processing Library.
IndexError(const std::string &msg="")
Constructs an IndexError object with the error message set to msg.
virtual ~BadCast()
Virtual destructor.
Exception(const std::string &msg="")
Constructs an Exception object with the error message set to msg.
Thrown when an operation requires or expects a valid pointer but a null pointer was provided.
Definition: Base/Exceptions.hpp:95
OperationFailed(const std::string &msg="")
Constructs an OperationFailed object with the error message set to msg.
virtual ~OperationFailed()
Virtual destructor.
CalculationFailed(const std::string &msg="")
Constructs a CalaculationFailed object with the error message set to msg.
virtual ~Exception()
Virtual destructor.
virtual ~IndexError()
Virtual destructor.