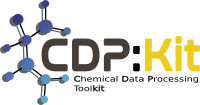 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_AUTOMORPHISMGROUPSEARCH_HPP
30 #define CDPL_CHEM_AUTOMORPHISMGROUPSEARCH_HPP
57 static constexpr
unsigned int DEF_ATOM_PROPERTY_FLAGS =
66 static constexpr
unsigned int DEF_BOND_PROPERTY_FLAGS =
88 unsigned int bond_flags = DEF_BOND_PROPERTY_FLAGS);
257 getAtomMatchExpression(
const Atom& atom)
const;
260 getBondMatchExpression(
const Bond& bond)
const;
265 class AtomMatchExpression :
public MatchExpression<Atom, MolecularGraph>
272 bool requiresAtomBondMapping()
const;
284 mutable unsigned int type;
285 mutable unsigned int hybState;
286 mutable std::size_t isotope;
287 mutable std::size_t hCount;
289 mutable bool aromatic;
290 mutable std::size_t expBondCount;
293 class BondMatchExpression :
public MatchExpression<Bond, MolecularGraph>
297 BondMatchExpression(AutomorphismGroupSearch* parent):
300 bool requiresAtomBondMapping()
const;
302 bool operator()(
const Bond& query_bond,
const MolecularGraph& query_molgraph,
303 const Bond& target_bond,
const MolecularGraph& target_molgraph,
306 bool operator()(
const Bond& query_bond,
const MolecularGraph& query_molgraph,
307 const Bond& target_bond,
const MolecularGraph& target_molgraph,
308 const AtomBondMapping& mapping,
const Base::Any& aux_data)
const;
311 AutomorphismGroupSearch* parent;
312 mutable std::size_t order;
314 mutable bool aromatic;
317 class MolGraphMatchExpression :
public MatchExpression<MolecularGraph>
321 MolGraphMatchExpression(
const AutomorphismGroupSearch* parent):
324 bool requiresAtomBondMapping()
const;
326 bool operator()(
const MolecularGraph& query_molgraph,
327 const MolecularGraph& target_molgraph,
330 bool operator()(
const MolecularGraph& query_molgraph,
331 const MolecularGraph& target_molgraph,
332 const AtomBondMapping& mapping,
const Base::Any& aux_data)
const;
335 const AutomorphismGroupSearch* parent;
342 SubstructureSearch substructSearch;
343 bool incIdentityMapping;
344 unsigned int atomPropFlags;
345 unsigned int bondPropFlags;
346 AtomMatchExprPtr atomMatchExpr;
347 BondMatchExprPtr bondMatchExpr;
348 MolGraphMatchExprPtr molGraphMatchExpr;
349 MappingCallbackFunction mappingCallbackFunc;
350 const Atom* lastQueryAtom;
351 const Bond* lastQueryBond;
356 #endif // CDPL_CHEM_AUTOMORPHISMGROUPSEARCH_HPP
ConstMappingIterator begin() const
Returns a constant iterator pointing to the beginning of the stored atom/bond mapping objects.
const unsigned int FORMAL_CHARGE
Specifies the formal charge of an atom.
Definition: Chem/AtomPropertyFlag.hpp:73
void includeIdentityMapping(bool include)
std::size_t getMaxNumMappings() const
Returns the specified limit on the number of stored atom/bond mappings.
const unsigned int AROMATICITY
Specifies the membership of a bond in aromatic rings.
Definition: BondPropertyFlag.hpp:73
Definition of the preprocessor macro CDPL_CHEM_API.
void addAtomMappingConstraint(std::size_t atom1_idx, std::size_t atom2_idx)
Adds a constraint on the allowed atom mappings.
const unsigned int Any
Specifies any atom.
Definition: SybylAtomType.hpp:198
const MappingCallbackFunction & getFoundMappingCallback() const
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
ConstMappingIterator end() const
Returns a constant iterator pointing to the end of the stored atom/bond mapping objects.
Bond.
Definition: Bond.hpp:50
const unsigned int CONFIGURATION
Specifies the steric configuration of a double bond.
Definition: BondPropertyFlag.hpp:78
void setMaxNumMappings(std::size_t max_num_mappings)
Allows to specify a limit on the number of stored atom/bond mappings.
MappingIterator end()
Returns a mutable iterator pointing to the end of the stored atom/bond mapping objects.
AutomorphismGroupSearch(unsigned int atom_flags=DEF_ATOM_PROPERTY_FLAGS, unsigned int bond_flags=DEF_BOND_PROPERTY_FLAGS)
Constructs and initializes a AutomorphismGroupSearch instance.
std::size_t getNumMappings() const
Returns the number of atom/bond mappings that were recorded in the last call to findMappings().
ConstMappingIterator getMappingsBegin() const
Returns a constant iterator pointing to the beginning of the stored atom/bond mapping objects.
Atom.
Definition: Atom.hpp:52
std::function< bool(const MolecularGraph &, const AtomBondMapping &)> MappingCallbackFunction
Definition: AutomorphismGroupSearch.hpp:82
void clearAtomMappingConstraints()
Clears all previously defined atom mapping constraints.
const unsigned int EXPLICIT_BOND_COUNT
Specifies the explicit bond count of an atom.
Definition: Chem/AtomPropertyFlag.hpp:118
MolecularGraph.
Definition: MolecularGraph.hpp:52
const AtomBondMapping & getMapping(std::size_t idx) const
Returns a const reference to the stored atom/bond mapping object at index idx.
Definition of constants in namespace CDPL::Chem::AtomPropertyFlag.
const unsigned int CONFIGURATION
Specifies the configuration of a stereogenic atom.
Definition: Chem/AtomPropertyFlag.hpp:98
A safe, type checked container for arbitrary data of variable type.
Definition: Any.hpp:59
MappingIterator begin()
Returns a mutable iterator pointing to the beginning of the stored atom/bond mapping objects.
void setAtomPropertyFlags(unsigned int flags)
std::shared_ptr< MatchExpression > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated MatchExpression instances.
Definition: MatchExpression.hpp:81
const unsigned int ORDER
Specifies the order of a bond.
Definition: BondPropertyFlag.hpp:63
bool findMappings(const MolecularGraph &molgraph)
Searches for the possible atom/bond mappings in the automorphism group of the given molecular graph.
ConstMappingIterator getMappingsEnd() const
Returns a constant iterator pointing to the end of the stored atom/bond mapping objects.
boost::indirect_iterator< ABMappingList::const_iterator, const AtomBondMapping > ConstMappingIterator
A constant random access iterator used to iterate over the stored atom/bond mapping objects.
Definition: SubstructureSearch.hpp:83
const unsigned int TOPOLOGY
Specifies the ring/chain topology of a bond.
Definition: BondPropertyFlag.hpp:68
const unsigned int TYPE
Specifies the generic type or element of an atom.
Definition: Chem/AtomPropertyFlag.hpp:63
const unsigned int ISOTOPE
Specifies the isotopic mass of an atom.
Definition: Chem/AtomPropertyFlag.hpp:68
void clearBondMappingConstraints()
Clears all previously defined bond mapping constraints.
A generic boolean expression interface for the implementation of query/target object equivalence test...
Definition: MatchExpression.hpp:75
unsigned int getBondPropertyFlags() const
Definition of the class CDPL::Chem::MatchExpression.
The namespace of the Chemical Data Processing Library.
unsigned int getAtomPropertyFlags() const
void setFoundMappingCallback(const MappingCallbackFunction &func)
SubstructureSearch::MappingIterator MappingIterator
A mutable random access iterator used to iterate over the stored atom/bond mapping objects.
Definition: AutomorphismGroupSearch.hpp:75
MappingIterator getMappingsBegin()
Returns a mutable iterator pointing to the beginning of the stored atom/bond mapping objects.
const unsigned int AROMATICITY
Specifies the membership of an atom in aromatic rings.
Definition: Chem/AtomPropertyFlag.hpp:93
A data structure for the common storage of related atom to atom and bond to bond mappings.
Definition: AtomBondMapping.hpp:55
Definition of the class CDPL::Chem::SubstructureSearch.
void addBondMappingConstraint(std::size_t bond1_idx, std::size_t bond2_idx)
Adds a constraint on the allowed bond mappings.
std::shared_ptr< AutomorphismGroupSearch > SharedPointer
Definition: AutomorphismGroupSearch.hpp:70
const unsigned int H_COUNT
Specifies the hydrogen count of an atom.
Definition: Chem/AtomPropertyFlag.hpp:78
boost::indirect_iterator< ABMappingList::iterator, AtomBondMapping > MappingIterator
A mutable random access iterator used to iterate over the stored atom/bond mapping objects.
Definition: SubstructureSearch.hpp:78
SubstructureSearch::ConstMappingIterator ConstMappingIterator
A constant random access iterator used to iterate over the stored atom/bond mapping objects.
Definition: AutomorphismGroupSearch.hpp:80
Definition of constants in namespace CDPL::Chem::BondPropertyFlag.
bool identityMappingIncluded() const
AutomorphismGroupSearch.
Definition: AutomorphismGroupSearch.hpp:52
const unsigned int HYBRIDIZATION_STATE
Specifies the hybridization state an atom.
Definition: Chem/AtomPropertyFlag.hpp:123
AtomBondMapping & getMapping(std::size_t idx)
Returns a non-const reference to the stored atom/bond mapping object at index idx.
MappingIterator getMappingsEnd()
Returns a mutable iterator pointing to the end of the stored atom/bond mapping objects.
void setBondPropertyFlags(unsigned int flags)