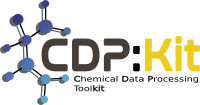 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_DESCR_AUTOCORRELATION3DVECTORCALCULATOR_HPP
30 #define CDPL_DESCR_AUTOCORRELATION3DVECTORCALCULATOR_HPP
149 template <
typename Iter,
typename Vec>
153 template <
typename Iter>
154 void init(Iter beg, Iter end);
157 double radiusIncrement;
158 std::size_t numSteps;
159 std::size_t numEntities;
171 template <
typename T>
173 startRadius(0.0), radiusIncrement(0.1), numSteps(99)
176 template <
typename T>
179 startRadius = start_radius;
182 template <
typename T>
185 radiusIncrement = radius_inc;
188 template <
typename T>
191 numSteps = num_steps;
194 template <
typename T>
200 template <
typename T>
203 return radiusIncrement;
206 template <
typename T>
212 template <
typename T>
218 template <
typename T>
224 template <
typename T>
225 template <
typename Iter,
typename Vec>
230 for (std::size_t i = 0; i <= numSteps; i++)
234 const double max_radius = startRadius + (numSteps + 1) * radiusIncrement;
236 for (std::size_t i = 0; i < numEntities; i++) {
237 for (std::size_t j = i; j < numEntities; j++) {
241 tmp.assign(entityPositions[i] - entityPositions[j]);
245 if (dist < startRadius || dist >= max_radius)
248 std::size_t idx = std::floor((dist - startRadius) / radiusIncrement);
250 vec[idx] = weightMatrix(i, j);
255 template <
typename T>
256 template <
typename Iter>
259 numEntities = std::distance(beg, end);
261 weightMatrix.resize(numEntities, numEntities,
false);
262 entityPositions.resize(numEntities);
264 for (std::size_t i = 0; beg != end; i++, ++beg) {
265 const EntityType& entity1 = *beg;
268 entityPositions[i] = coordsFunc(entity1);
270 entityPositions[i].clear(0.0);
274 for (Iter it = beg; it != end; ++it, j++) {
275 const EntityType& entity2 = *it;
278 weightMatrix(i, j) = 1.0;
280 weightMatrix(i, j) = weightFunc(entity1, entity2);
285 #endif // CDPL_DESCR_AUTOCORRELATION3DVECTORCALCULATOR_HPP
void setEntity3DCoordinatesFunction(const Entity3DCoordinatesFunction &func)
Allows to specify the entity 3D coordinates function.
Definition: AutoCorrelation3DVectorCalculator.hpp:219
Definition of the class CDPL::Math::VectorArray.
CVector< double, 3 > Vector3D
A bounded 3 element vector holding floating point values of type double.
Definition: Vector.hpp:1637
Atom.
Definition: Atom.hpp:52
AutoCorrelation3DVectorCalculator()
Constructs the AutoCorrelation3DVectorCalculator instance.
Definition: AutoCorrelation3DVectorCalculator.hpp:172
T EntityType
Definition: AutoCorrelation3DVectorCalculator.hpp:55
void setEntityPairWeightFunction(const EntityPairWeightFunction &func)
Allows to specify a custom entity pair weight function.
Definition: AutoCorrelation3DVectorCalculator.hpp:213
double getRadiusIncrement() const
Returns the radius step size between successive autocorrelation vector elements.
Definition: AutoCorrelation3DVectorCalculator.hpp:201
QuaternionVectorAdapter< E > vec(QuaternionExpression< E > &e)
Definition: QuaternionAdapter.hpp:237
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
std::function< const Math::Vector3D &(const EntityType &)> Entity3DCoordinatesFunction
Type of the generic functor class used to store a user-defined entity 3D coordinates function.
Definition: AutoCorrelation3DVectorCalculator.hpp:73
double getStartRadius() const
Returns the starting value of the radius.
Definition: AutoCorrelation3DVectorCalculator.hpp:195
std::function< double(const EntityType &, const EntityType &)> EntityPairWeightFunction
Type of the generic functor class used to store a user-defined entity pair weight function.
Definition: AutoCorrelation3DVectorCalculator.hpp:64
void setRadiusIncrement(double radius_inc)
Sets the radius step size between successive autocorrelation vector elements.
Definition: AutoCorrelation3DVectorCalculator.hpp:183
The namespace of the Chemical Data Processing Library.
std::size_t getNumSteps() const
Returns the number of performed radius incrementation steps.
Definition: AutoCorrelation3DVectorCalculator.hpp:207
Definition of matrix data types.
AutoCorrelation3DVectorCalculator.
Definition: AutoCorrelation3DVectorCalculator.hpp:52
void calculate(Iter beg, Iter end, Vec &vec)
Calculates the RDF code of an entity sequence.
Definition: AutoCorrelation3DVectorCalculator.hpp:226
void setStartRadius(double start_radius)
Sets the starting value of the radius.
Definition: AutoCorrelation3DVectorCalculator.hpp:177
VectorNorm2< E >::ResultType length(const VectorExpression< E > &e)
Definition: VectorExpression.hpp:553
void setNumSteps(std::size_t num_steps)
Sets the number of desired radius incrementation steps.
Definition: AutoCorrelation3DVectorCalculator.hpp:189