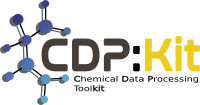 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_ATOM_HPP
30 #define CDPL_CHEM_ATOM_HPP
231 #endif // CDPL_CHEM_ATOM_HPP
virtual std::size_t getNumBonds() const =0
Returns the number of incident bonds.
Definition of the preprocessor macro CDPL_CHEM_API.
virtual std::size_t getIndex() const =0
Returns the index of the atom in its parent molecule.
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
virtual const Bond & getBond(std::size_t idx) const =0
Returns a const reference to the incident bond at index idx.
Bond.
Definition: Bond.hpp:50
virtual const Bond & getBondToAtom(const Atom &atom) const =0
Returns a const reference to the Chem::Bond object that connects this atom to the argument atom.
ConstBondIterator getBondsBegin() const
Returns a constant iterator pointing to the beginning of the stored const Chem::Bond objects.
virtual ~Atom()
Virtual destructor.
Definition: Atom.hpp:226
ConstBondIterator getBondsEnd() const
Returns a constant iterator pointing to the end of the stored const Chem::Bond objects.
A STL compatible random access iterator for container elements accessible by index.
Definition: IndexedElementIterator.hpp:125
Definition of the class CDPL::Chem::BondContainer.
Atom.
Definition: Atom.hpp:52
virtual const Atom & getAtom(std::size_t idx) const =0
Returns a const reference to the connected atom at index idx.
ConstAtomIterator getAtomsBegin() const
Returns a constant iterator pointing to the beginning of the stored const Chem::Atom objects.
virtual Atom & getAtom(std::size_t idx)=0
Returns a non-const reference to the connected atom at index idx.
Definition of the class CDPL::Chem::Entity3D.
Molecule.
Definition: Molecule.hpp:49
virtual std::size_t getBondIndex(const Bond &bond) const =0
Returns the index of the specified incident bond.
A common interface for data-structures that support a random access to stored Chem::Atom instances.
Definition: AtomContainer.hpp:55
BondContainer::BondIterator BondIterator
A mutable random access iterator used to iterate over the incident bonds.
Definition: Atom.hpp:68
Definition of the class CDPL::Chem::AtomContainer.
AtomContainer::ConstAtomIterator ConstAtomIterator
A constant random access iterator used to iterate over the connected atoms.
Definition: Atom.hpp:63
virtual Bond & getBond(std::size_t idx)=0
Returns a non-const reference to the incident bond at index idx.
virtual Molecule & getMolecule()=0
Returns a non-const reference to the parent molecule.
virtual Bond & getBondToAtom(const Atom &atom)=0
Returns a non-const reference to the Chem::Bond object that connects this atom to the argument atom.
The namespace of the Chemical Data Processing Library.
virtual const Molecule & getMolecule() const =0
Returns a const reference to the parent molecule.
A common interface for data-structures that support a random access to stored Chem::Bond instances.
Definition: BondContainer.hpp:54
virtual bool containsBond(const Bond &bond) const =0
Tells whether the specified bond is incident to this atom.
AtomContainer::AtomIterator AtomIterator
A mutable random access iterator used to iterate over the connected atoms.
Definition: Atom.hpp:58
virtual bool containsAtom(const Atom &atom) const =0
Tells whether this atom and the argument atom are connected by a bond.
virtual Bond * findBondToAtom(const Atom &atom)=0
Returns a pointer to the non-const Chem::Bond object that connects this atom to the argument atom.
Entity3D.
Definition: Entity3D.hpp:46
virtual const Bond * findBondToAtom(const Atom &atom) const =0
Returns a pointer to the const Chem::Bond object that connects this atom to the argument atom.
virtual std::size_t getAtomIndex(const Atom &atom) const =0
Returns the index of the specified connected atom.
BondContainer::ConstBondIterator ConstBondIterator
A constant random access iterator used to iterate over the incident bonds.
Definition: Atom.hpp:73
ConstAtomIterator getAtomsEnd() const
Returns a constant iterator pointing to the end of the stored const Chem::Atom objects.
virtual std::size_t getNumAtoms() const =0
Returns the number of connected atoms.