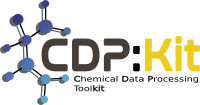 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_ATOMTYPE_HPP
30 #define CDPL_CHEM_ATOMTYPE_HPP
57 const unsigned int H = 1;
62 const unsigned int D = 1;
67 const unsigned int T = 1;
72 const unsigned int He = 2;
77 const unsigned int Li = 3;
82 const unsigned int Be = 4;
87 const unsigned int B = 5;
92 const unsigned int C = 6;
97 const unsigned int N = 7;
102 const unsigned int O = 8;
107 const unsigned int F = 9;
112 const unsigned int Ne = 10;
117 const unsigned int Na = 11;
122 const unsigned int Mg = 12;
127 const unsigned int Al = 13;
132 const unsigned int Si = 14;
137 const unsigned int P = 15;
142 const unsigned int S = 16;
147 const unsigned int Cl = 17;
152 const unsigned int Ar = 18;
157 const unsigned int K = 19;
162 const unsigned int Ca = 20;
167 const unsigned int Sc = 21;
172 const unsigned int Ti = 22;
177 const unsigned int V = 23;
182 const unsigned int Cr = 24;
187 const unsigned int Mn = 25;
192 const unsigned int Fe = 26;
197 const unsigned int Co = 27;
202 const unsigned int Ni = 28;
207 const unsigned int Cu = 29;
212 const unsigned int Zn = 30;
217 const unsigned int Ga = 31;
222 const unsigned int Ge = 32;
227 const unsigned int As = 33;
232 const unsigned int Se = 34;
237 const unsigned int Br = 35;
242 const unsigned int Kr = 36;
247 const unsigned int Rb = 37;
252 const unsigned int Sr = 38;
257 const unsigned int Y = 39;
262 const unsigned int Zr = 40;
267 const unsigned int Nb = 41;
272 const unsigned int Mo = 42;
277 const unsigned int Tc = 43;
282 const unsigned int Ru = 44;
287 const unsigned int Rh = 45;
292 const unsigned int Pd = 46;
297 const unsigned int Ag = 47;
302 const unsigned int Cd = 48;
307 const unsigned int In = 49;
312 const unsigned int Sn = 50;
317 const unsigned int Sb = 51;
322 const unsigned int Te = 52;
327 const unsigned int I = 53;
332 const unsigned int Xe = 54;
337 const unsigned int Cs = 55;
342 const unsigned int Ba = 56;
347 const unsigned int La = 57;
352 const unsigned int Ce = 58;
357 const unsigned int Pr = 59;
362 const unsigned int Nd = 60;
367 const unsigned int Pm = 61;
372 const unsigned int Sm = 62;
377 const unsigned int Eu = 63;
382 const unsigned int Gd = 64;
387 const unsigned int Tb = 65;
392 const unsigned int Dy = 66;
397 const unsigned int Ho = 67;
402 const unsigned int Er = 68;
407 const unsigned int Tm = 69;
412 const unsigned int Yb = 70;
417 const unsigned int Lu = 71;
422 const unsigned int Hf = 72;
427 const unsigned int Ta = 73;
432 const unsigned int W = 74;
437 const unsigned int Re = 75;
442 const unsigned int Os = 76;
447 const unsigned int Ir = 77;
452 const unsigned int Pt = 78;
457 const unsigned int Au = 79;
462 const unsigned int Hg = 80;
467 const unsigned int Tl = 81;
472 const unsigned int Pb = 82;
477 const unsigned int Bi = 83;
482 const unsigned int Po = 84;
487 const unsigned int At = 85;
492 const unsigned int Rn = 86;
497 const unsigned int Fr = 87;
502 const unsigned int Ra = 88;
507 const unsigned int Ac = 89;
512 const unsigned int Th = 90;
517 const unsigned int Pa = 91;
522 const unsigned int U = 92;
527 const unsigned int Np = 93;
532 const unsigned int Pu = 94;
537 const unsigned int Am = 95;
542 const unsigned int Cm = 96;
547 const unsigned int Bk = 97;
552 const unsigned int Cf = 98;
557 const unsigned int Es = 99;
562 const unsigned int Fm = 100;
567 const unsigned int Md = 101;
572 const unsigned int No = 102;
577 const unsigned int Lr = 103;
582 const unsigned int Rf = 104;
587 const unsigned int Db = 105;
592 const unsigned int Sg = 106;
597 const unsigned int Bh = 107;
602 const unsigned int Hs = 108;
607 const unsigned int Mt = 109;
672 #endif // CDPL_CHEM_ATOMTYPE_HPP
const unsigned int Fm
Specifies Fermium.
Definition: AtomType.hpp:562
const unsigned int H
Specifies Hydrogen.
Definition: AtomType.hpp:57
const unsigned int Nd
Specifies Neodymium.
Definition: AtomType.hpp:362
const unsigned int Ba
Specifies Barium.
Definition: AtomType.hpp:342
const unsigned int Q
A generic type that covers any element except hydrogen and carbon.
Definition: AtomType.hpp:627
const unsigned int At
Specifies Astatine.
Definition: AtomType.hpp:487
const unsigned int Pd
Specifies Palladium.
Definition: AtomType.hpp:292
const unsigned int Md
Specifies Mendelevium.
Definition: AtomType.hpp:567
const unsigned int Ar
Specifies Argon.
Definition: AtomType.hpp:152
const unsigned int Ne
Specifies Neon.
Definition: AtomType.hpp:112
const unsigned int Cd
Specifies Cadmium.
Definition: AtomType.hpp:302
const unsigned int Cu
Specifies Copper.
Definition: AtomType.hpp:207
const unsigned int Pm
Specifies Promethium.
Definition: AtomType.hpp:367
const unsigned int Pb
Specifies Lead.
Definition: AtomType.hpp:472
const unsigned int Sb
Specifies Antimony.
Definition: AtomType.hpp:317
const unsigned int Li
Specifies Lithium.
Definition: AtomType.hpp:77
const unsigned int Ga
Specifies Gallium.
Definition: AtomType.hpp:217
const unsigned int Rb
Specifies Rubidium.
Definition: AtomType.hpp:247
const unsigned int Am
Specifies Americium.
Definition: AtomType.hpp:537
const unsigned int B
Specifies Boron.
Definition: AtomType.hpp:87
const unsigned int Hs
Specifies Hassium.
Definition: AtomType.hpp:602
const unsigned int S
Specifies Sulfur.
Definition: AtomType.hpp:142
const unsigned int HET
A generic type for heteroatoms (N, O, S or P).
Definition: AtomType.hpp:662
const unsigned int Si
Specifies Silicon.
Definition: AtomType.hpp:132
const unsigned int Pu
Specifies Plutonium.
Definition: AtomType.hpp:532
const unsigned int Tb
Specifies Terbium.
Definition: AtomType.hpp:387
const unsigned int Mn
Specifies Manganese.
Definition: AtomType.hpp:187
const unsigned int Pa
Specifies Protactinium.
Definition: AtomType.hpp:517
const unsigned int Hf
Specifies Hafnium.
Definition: AtomType.hpp:422
const unsigned int Te
Specifies Tellurium.
Definition: AtomType.hpp:322
const unsigned int Np
Specifies Neptunium.
Definition: AtomType.hpp:527
const unsigned int Gd
Specifies Gadolinium.
Definition: AtomType.hpp:382
const unsigned int Db
Specifies Dubnium.
Definition: AtomType.hpp:587
const unsigned int Bi
Specifies Bismuth.
Definition: AtomType.hpp:477
const unsigned int Be
Specifies Beryllium.
Definition: AtomType.hpp:82
const unsigned int Xe
Specifies Xenon.
Definition: AtomType.hpp:332
const unsigned int Mt
Specifies Meitnerium.
Definition: AtomType.hpp:607
const unsigned int Ni
Specifies Nickel.
Definition: AtomType.hpp:202
const unsigned int X
A generic type that covers any element that is a halogen.
Definition: AtomType.hpp:647
const unsigned int N
Specifies Nitrogen.
Definition: AtomType.hpp:97
const unsigned int I
Specifies Iodine.
Definition: AtomType.hpp:327
const unsigned int Y
Specifies Yttrium.
Definition: AtomType.hpp:257
const unsigned int Rh
Specifies Rhodium.
Definition: AtomType.hpp:287
const unsigned int Ce
Specifies Cerium.
Definition: AtomType.hpp:352
const unsigned int Bh
Specifies Bohrium.
Definition: AtomType.hpp:597
const unsigned int As
Specifies Arsenic.
Definition: AtomType.hpp:227
const unsigned int Es
Specifies Einsteinium.
Definition: AtomType.hpp:557
const unsigned int Bk
Specifies Berkelium.
Definition: AtomType.hpp:547
const unsigned int W
Specifies Tungsten.
Definition: AtomType.hpp:432
const unsigned int MH
A generic type that covers hydrogen and any element that is a metal.
Definition: AtomType.hpp:642
const unsigned int Sm
Specifies Samarium.
Definition: AtomType.hpp:372
const unsigned int U
Specifies Uranium.
Definition: AtomType.hpp:522
const unsigned int ANY
A generic type that covers any element (equivalent to AtomType::AH).
Definition: AtomType.hpp:657
const unsigned int Ti
Specifies Titanium.
Definition: AtomType.hpp:172
const unsigned int Kr
Specifies Krypton.
Definition: AtomType.hpp:242
const unsigned int Fr
Specifies Francium.
Definition: AtomType.hpp:497
const unsigned int Co
Specifies Cobalt.
Definition: AtomType.hpp:197
const unsigned int Eu
Specifies Europium.
Definition: AtomType.hpp:377
const unsigned int Re
Specifies Rhenium.
Definition: AtomType.hpp:437
const unsigned int UNKNOWN
Specifies an atom with an unknown or undefined atom type.
Definition: AtomType.hpp:52
const unsigned int Po
Specifies Polonium.
Definition: AtomType.hpp:482
const unsigned int M
A generic type that covers any element that is a metal.
Definition: AtomType.hpp:637
const unsigned int Lu
Specifies Lutetium.
Definition: AtomType.hpp:417
const unsigned int Tm
Specifies Thulium.
Definition: AtomType.hpp:407
const unsigned int QH
A generic type that covers any element except carbon.
Definition: AtomType.hpp:632
const unsigned int Nb
Specifies Niobium.
Definition: AtomType.hpp:267
const unsigned int XH
A generic type that covers hydrogen and any element that is a halogen.
Definition: AtomType.hpp:652
const unsigned int Mo
Specifies Molybdenum.
Definition: AtomType.hpp:272
const unsigned int AH
A generic type that covers any element.
Definition: AtomType.hpp:622
const unsigned int Ru
Specifies Ruthenium.
Definition: AtomType.hpp:282
const unsigned int Tl
Specifies Thallium.
Definition: AtomType.hpp:467
const unsigned int Se
Specifies Selenium.
Definition: AtomType.hpp:232
const unsigned int Ca
Specifies Calcium.
Definition: AtomType.hpp:162
const unsigned int Ac
Specifies Actinium.
Definition: AtomType.hpp:507
const unsigned int Sn
Specifies Tin.
Definition: AtomType.hpp:312
const unsigned int Zr
Specifies Zirconium.
Definition: AtomType.hpp:262
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
const unsigned int Yb
Specifies Ytterbium.
Definition: AtomType.hpp:412
const unsigned int Na
Specifies Sodium.
Definition: AtomType.hpp:117
const unsigned int Ra
Specifies Radium.
Definition: AtomType.hpp:502
const unsigned int Cf
Specifies Californium.
Definition: AtomType.hpp:552
const unsigned int Al
Specifies Aluminium.
Definition: AtomType.hpp:127
const unsigned int Ir
Specifies Iridium.
Definition: AtomType.hpp:447
const unsigned int Fe
Specifies Iron.
Definition: AtomType.hpp:192
const unsigned int Br
Specifies Bromine.
Definition: AtomType.hpp:237
const unsigned int MAX_TYPE
Marks the end of all supported atom types.
Definition: AtomType.hpp:667
const unsigned int Er
Specifies Erbium.
Definition: AtomType.hpp:402
The namespace of the Chemical Data Processing Library.
const unsigned int K
Specifies Potassium.
Definition: AtomType.hpp:157
const unsigned int Rf
Specifies Rutherfordium.
Definition: AtomType.hpp:582
const unsigned int Sr
Specifies Strontium.
Definition: AtomType.hpp:252
const unsigned int Au
Specifies Gold.
Definition: AtomType.hpp:457
const unsigned int Sg
Specifies Seaborgium.
Definition: AtomType.hpp:592
const unsigned int Cr
Specifies Chromium.
Definition: AtomType.hpp:182
const unsigned int Tc
Specifies Technetium.
Definition: AtomType.hpp:277
const unsigned int Th
Specifies Thorium.
Definition: AtomType.hpp:512
const unsigned int Os
Specifies Osmium.
Definition: AtomType.hpp:442
const unsigned int He
Specifies Helium.
Definition: AtomType.hpp:72
const unsigned int Hg
Specifies Mercury.
Definition: AtomType.hpp:462
const unsigned int Ho
Specifies Holmium.
Definition: AtomType.hpp:397
const unsigned int MAX_ATOMIC_NO
Marks the end of the supported chemical elements.
Definition: AtomType.hpp:612
const unsigned int Pt
Specifies Platinum.
Definition: AtomType.hpp:452
const unsigned int D
Specifies Hydrogen (Deuterium).
Definition: AtomType.hpp:62
const unsigned int Dy
Specifies Dysprosium.
Definition: AtomType.hpp:392
const unsigned int In
Specifies Indium.
Definition: AtomType.hpp:307
const unsigned int Ta
Specifies Tantalum.
Definition: AtomType.hpp:427
const unsigned int Pr
Specifies Praseodymium.
Definition: AtomType.hpp:357
const unsigned int Sc
Specifies Scandium.
Definition: AtomType.hpp:167
const unsigned int O
Specifies Oxygen.
Definition: AtomType.hpp:102
const unsigned int Ge
Specifies Germanium.
Definition: AtomType.hpp:222
const unsigned int Cm
Specifies Curium.
Definition: AtomType.hpp:542
const unsigned int Zn
Specifies Zinc.
Definition: AtomType.hpp:212
const unsigned int Cs
Specifies Caesium.
Definition: AtomType.hpp:337
const unsigned int Lr
Specifies Lawrencium.
Definition: AtomType.hpp:577
const unsigned int F
Specifies Fluorine.
Definition: AtomType.hpp:107
const unsigned int A
A generic type that covers any element except hydrogen.
Definition: AtomType.hpp:617
const unsigned int Cl
Specifies Chlorine.
Definition: AtomType.hpp:147
const unsigned int C
Specifies Carbon.
Definition: AtomType.hpp:92
const unsigned int No
Specifies Nobelium.
Definition: AtomType.hpp:572
const unsigned int Mg
Specifies Magnesium.
Definition: AtomType.hpp:122
const unsigned int Ag
Specifies Silver.
Definition: AtomType.hpp:297
const unsigned int La
Specifies Lanthanum.
Definition: AtomType.hpp:347
const unsigned int Rn
Specifies Radon.
Definition: AtomType.hpp:492
const unsigned int P
Specifies Phosphorus.
Definition: AtomType.hpp:137