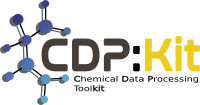 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_ATOMMATCHCONSTRAINT_HPP
30 #define CDPL_CHEM_ATOMMATCHCONSTRAINT_HPP
42 namespace AtomMatchConstraint
54 const unsigned int TYPE = 1;
154 #endif // CDPL_CHEM_ATOMMATCHCONSTRAINT_HPP
const unsigned int RING_TOPOLOGY
Specifies a constraint on the ring-membership of the target atom.
Definition: AtomMatchConstraint.hpp:124
const unsigned int CONSTRAINT_LIST
Specifies a constraint which requires the target atom to fulfill additional contraints specified by a...
Definition: AtomMatchConstraint.hpp:49
const unsigned int EXPLICIT_BOND_COUNT
Specifies a constraint on the explicit bond count of the target atom.
Definition: AtomMatchConstraint.hpp:99
const unsigned int IMPLICIT_H_COUNT
Specifies a constraint on the implicit hydrogen count of the target atom.
Definition: AtomMatchConstraint.hpp:84
const unsigned int EXPLICIT_VALENCE
Specifies a constraint on the explicit valence of the target atom.
Definition: AtomMatchConstraint.hpp:114
const unsigned int SSSR_RING_COUNT
Specifies a constraint on the number of rings (from the SSSR) the target atom is a member of.
Definition: AtomMatchConstraint.hpp:139
const unsigned int HYBRIDIZATION_STATE
Specifies a constraint on the hybridization state of the target atom.
Definition: AtomMatchConstraint.hpp:149
const unsigned int EXPLICIT_H_COUNT
Specifies a constraint on the explicit hydrogen count of the target atom.
Definition: AtomMatchConstraint.hpp:89
const unsigned int RING_BOND_COUNT
Specifies a constraint on the ring bond count of the target atom.
Definition: AtomMatchConstraint.hpp:74
const unsigned int ENVIRONMENT
Specifies a constraint on the structural environment of the target atom.
Definition: AtomMatchConstraint.hpp:59
const unsigned int AROMATICITY
Specifies a constraint on the aromaticity of the target atom.
Definition: AtomMatchConstraint.hpp:119
const unsigned int HEAVY_BOND_COUNT
Specifies a constraint on the heavy bond count of the target atom.
Definition: AtomMatchConstraint.hpp:104
const unsigned int SSSR_RING_SIZE
Specifies a constraint on the size of the rings (from the SSSR) the target atom is a member of.
Definition: AtomMatchConstraint.hpp:144
const unsigned int UNSATURATION
Specifies a constraint on the (non-)membership of the target atom in a double or triple bond.
Definition: AtomMatchConstraint.hpp:134
The namespace of the Chemical Data Processing Library.
const unsigned int H_COUNT
Specifies a constraint on the total hydrogen count of the target atom.
Definition: AtomMatchConstraint.hpp:79
const unsigned int ISOTOPE
Specifies a constraint on the isotopic mass of the target atom.
Definition: AtomMatchConstraint.hpp:64
const unsigned int BOND_COUNT
Specifies a constraint on the total bond count of the target atom.
Definition: AtomMatchConstraint.hpp:94
const unsigned int CONFIGURATION
Specifies a constraint on the steric configuration of the target atom.
Definition: AtomMatchConstraint.hpp:129
const unsigned int TYPE
Specifies a constraint on the type of the target atom.
Definition: AtomMatchConstraint.hpp:54
const unsigned int VALENCE
Specifies a constraint on the valence of the target atom.
Definition: AtomMatchConstraint.hpp:109
const unsigned int CHARGE
Specifies a constraint on the fromal charge of the target atom.
Definition: AtomMatchConstraint.hpp:69