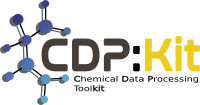 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_UTIL_ARRAY_HPP
30 #define CDPL_UTIL_ARRAY_HPP
41 #ifndef CDPL_UTIL_ARRAY_CHECK_INDEX
42 # ifdef CDPL_UTIL_ARRAY_CHECKS_DISABLE
43 # define CDPL_UTIL_ARRAY_CHECK_INDEX(idx, allow_end)
44 # else // !CDPL_UTIL_ARRAY_CHECKS_DISABLE
45 # define CDPL_UTIL_ARRAY_CHECK_INDEX(idx, allow_end) checkIndex(idx, allow_end)
46 # endif // CDPL_UTIL_ARRAY_CHECKS_DISABLE
47 #endif // !CDPL_UTIL_ARRAY_CHECK_INDEX
49 #ifndef CDPL_UTIL_ARRAY_CHECK_ITER
50 # ifdef CDPL_UTIL_ARRAY_CHECKS_DISABLE
51 # define CDPL_UTIL_ARRAY_CHECK_ITER(it, allow_end)
52 # else // !CDPL_UTIL_ARRAY_CHECKS_DISABLE
53 # define CDPL_UTIL_ARRAY_CHECK_ITER(it, allow_end) checkIterator(it, allow_end)
54 # endif // CDPL_UTIL_ARRAY_CHECKS_DISABLE
55 #endif // !CDPL_UTIL_ARRAY_CHECK_INDEX
90 template <
typename ValueType>
156 Array(std::size_t num_elem,
const ValueType& value = ValueType()):
157 data(num_elem, value) {}
164 template <
typename InputIter>
165 Array(
const InputIter& first,
const InputIter& last):
215 void resize(std::size_t num_elem,
const ValueType& value = ValueType());
260 void assign(std::size_t num_elem,
const ValueType& value = ValueType());
272 template <
typename InputIter>
273 void assign(
const InputIter& first,
const InputIter& last);
304 void insertElements(std::size_t idx, std::size_t num_elem,
const ValueType& value = ValueType());
322 template <
typename InputIter>
323 void insertElements(std::size_t idx,
const InputIter& first,
const InputIter& last);
333 template <
typename InputIter>
497 void setElement(std::size_t idx,
const ValueType& value = ValueType());
551 void throwIndexError()
const;
552 void throwRangeError()
const;
553 void throwOperationFailed()
const;
577 typedef std::pair<std::size_t, std::size_t>
STPair;
607 template <
typename ValueType>
618 template <
typename ValueType>
629 template <
typename ValueType>
640 template <
typename ValueType>
651 template <
typename ValueType>
662 template <
typename ValueType>
671 template <
typename ValueType>
677 template <
typename ValueType>
683 template <
typename ValueType>
689 template <
typename ValueType>
695 template <
typename ValueType>
701 template <
typename ValueType>
707 template <
typename ValueType>
713 template <
typename ValueType>
716 data.resize(n, value);
719 template <
typename ValueType>
722 return data.capacity();
725 template <
typename ValueType>
728 data.reserve(min_size);
731 template <
typename ValueType>
737 template <
typename ValueType>
740 data.swap(array.data);
743 template <
typename ValueType>
746 data.assign(n, value);
749 template <
typename ValueType>
750 template <
typename InputIter>
753 data.assign(first, last);
756 template <
typename ValueType>
759 data.push_back(value);
762 template <
typename ValueType>
767 data.insert(data.begin() + idx, value);
770 template <
typename ValueType>
772 const ValueType& value)
776 return data.insert(it, value);
779 template <
typename ValueType>
784 data.insert(data.begin() + idx, n, value);
787 template <
typename ValueType>
792 data.insert(it, n, value);
795 template <
typename ValueType>
796 template <
typename InputIter>
801 data.insert(data.begin() + idx, first, last);
804 template <
typename ValueType>
805 template <
typename InputIter>
810 data.insert(it, first, last);
813 template <
typename ValueType>
822 template <
typename ValueType>
827 data.erase(data.begin() + idx);
830 template <
typename ValueType>
835 return data.erase(it);
838 template <
typename ValueType>
846 throw Base::RangeError(std::string(getClassName()) +
": invalid iterator range: first > last");
848 return data.erase(first, last);
851 template <
typename ValueType>
859 template <
typename ValueType>
867 template <
typename ValueType>
875 template <
typename ValueType>
883 template <
typename ValueType>
889 template <
typename ValueType>
895 template <
typename ValueType>
901 template <
typename ValueType>
907 template <
typename ValueType>
913 template <
typename ValueType>
919 template <
typename ValueType>
925 template <
typename ValueType>
931 template <
typename ValueType>
934 return data.rbegin();
937 template <
typename ValueType>
940 return data.rbegin();
943 template <
typename ValueType>
949 template <
typename ValueType>
955 template <
typename ValueType>
963 template <
typename ValueType>
971 template <
typename ValueType>
979 template <
typename ValueType>
987 template <
typename ValueType>
995 template <
typename ValueType>
999 throwOperationFailed();
1002 template <
typename ValueType>
1005 if ((allow_end && idx > data.size()) || (!allow_end && idx >= data.size()))
1009 template <
typename ValueType>
1012 if (it < data.begin() || (allow_end && it > data.end()) || (!allow_end && it >= data.end()))
1016 template <
typename ValueType>
1019 if (it < data.begin() || (allow_end && it > data.end()) || (!allow_end && it >= data.end()))
1023 template <
typename ValueType>
1026 throw Base::IndexError(std::string(getClassName()) +
": element index out of bounds");
1029 template <
typename ValueType>
1032 throw Base::RangeError(std::string(getClassName()) +
": invalid iterator");
1035 template <
typename ValueType>
1038 throw Base::OperationFailed(std::string(getClassName()) +
": operation requires non-empty array");
1041 template <
typename ValueType>
1049 template <
typename ValueType>
1055 template <
typename ValueType>
1058 return (array1.getData() != array2.getData());
1061 template <
typename ValueType>
1064 return (array1.getData() <= array2.getData());
1067 template <
typename ValueType>
1070 return (array1.getData() >= array2.getData());
1073 template <
typename ValueType>
1076 return (array1.getData() < array2.getData());
1079 template <
typename ValueType>
1082 return (array1.getData() > array2.getData());
1087 #endif // CDPL_UTIL_ARRAY_HPP
Array< long > LArray
An array of unsigned integers of type long.
Definition: Array.hpp:572
ReverseElementIterator getElementsReverseEnd()
Returns a mutable iterator pointing to the end of the reversed array.
Definition: Array.hpp:950
Array< std::string > SArray
An array of std::string objects.
Definition: Array.hpp:592
void setElement(std::size_t idx, const ValueType &value=ValueType())
Assigns a new value to the element specified by the index idx.
Definition: Array.hpp:972
std::vector< ValueType > StorageType
Definition: Array.hpp:95
bool operator!=(const Array< ValueType > &array1, const Array< ValueType > &array2)
Inequality comparison operator.
ElementIterator removeElements(const ElementIterator &first, const ElementIterator &last)
Removes the elements pointed to by the iterators in the range [first, last).
Definition: Array.hpp:839
void popLastElement()
Removes the last element of the array.
Definition: Array.hpp:814
StorageType::reverse_iterator ReverseElementIterator
A mutable random access iterator used to iterate over the elements of the array in reverse order.
Definition: Array.hpp:143
std::size_t SizeType
The type of objects stored by the array.
Definition: Array.hpp:110
void insertElements(const ElementIterator &it, std::size_t num_elem, const ValueType &value=ValueType())
Inserts num_elem copies of value before the location specified by the iterator it.
Definition: Array.hpp:788
ConstElementIterator getElementsEnd() const
Returns a constant iterator pointing to the end of the array.
Definition: Array.hpp:896
ElementIterator end()
Returns a mutable iterator pointing to the end of the array.
Definition: Array.hpp:926
ConstElementIterator end() const
Returns a constant iterator pointing to the end of the array.
Definition: Array.hpp:920
Thrown to indicate that some requested operation has failed (e.g. due to unfulfilled preconditions or...
Definition: Base/Exceptions.hpp:211
Thrown to indicate that a value is out of range.
Definition: Base/Exceptions.hpp:114
ReverseElementIterator getElementsReverseBegin()
Returns a mutable iterator pointing to the beginning of the reversed array.
Definition: Array.hpp:938
A dynamic array class providing amortized constant time access to arbitrary elements.
Definition: Array.hpp:92
void reserve(std::size_t num_elem)
Preallocates memory for (at least) num_elem elements.
Definition: Array.hpp:726
ConstReverseElementIterator getElementsReverseEnd() const
Returns a constant iterator pointing to the end of the reversed array.
Definition: Array.hpp:944
void resize(std::size_t num_elem, const ValueType &value=ValueType())
Inserts or erases elements at the end so that the size becomes num_elem.
Definition: Array.hpp:714
void checkIndex(std::size_t idx, bool allow_end) const
Definition: Array.hpp:1003
ValueType & getLastElement()
Returns a non-const reference to the last element of the array.
Definition: Array.hpp:876
Array< BitSet > BitSetArray
An array of Util::BitSet objects.
Definition: Array.hpp:597
StorageType::const_reverse_iterator ConstReverseElementIterator
A constant random access iterator used to iterate over the elements of the array in reverse order.
Definition: Array.hpp:131
ConstElementIterator getElementsBegin() const
Returns a constant iterator pointing to the beginning of the array.
Definition: Array.hpp:884
Definition of the type CDPL::Util::BitSet.
void insertElements(std::size_t idx, std::size_t num_elem, const ValueType &value=ValueType())
Inserts num_elem copies of value before the location specified by the index idx.
Definition: Array.hpp:780
void checkIfNonEmpty() const
Definition: Array.hpp:996
void clear()
Erases all elements.
Definition: Array.hpp:732
void checkIterator(const ConstElementIterator &it, bool allow_end) const
Definition: Array.hpp:1017
Thrown to indicate that an index is out of range.
Definition: Base/Exceptions.hpp:152
Array(const InputIter &first, const InputIter &last)
Creates and initializes the array with copies of the elements in the range [first,...
Definition: Array.hpp:165
ValueType & getElement(std::size_t idx)
Returns a non-const reference to the element at index idx.
Definition: Array.hpp:964
ElementIterator getElementsBegin()
Returns a mutable iterator pointing to the beginning of the array.
Definition: Array.hpp:890
Array BaseType
Specifies for derived classes the type of the Array base class.
Definition: Array.hpp:119
void checkIterator(const ElementIterator &it, bool allow_end)
Definition: Array.hpp:1010
StorageType & getData()
Definition: Array.hpp:672
bool operator<(const Array< ValueType > &array1, const Array< ValueType > &array2)
Less than comparison operator.
bool operator>=(const Array< ValueType > &array1, const Array< ValueType > &array2)
Greater or equal comparison operator.
void addElement(const ValueType &value=ValueType())
Inserts a new element at the end of the array.
Definition: Array.hpp:757
virtual const char * getClassName() const
Returns the name of the (derived) array class.
Definition: Array.hpp:1042
std::size_t getSize() const
Returns the number of elements stored in the array.
Definition: Array.hpp:696
const ValueType & getElement(std::size_t idx) const
Returns a const reference to the element at index idx.
Definition: Array.hpp:956
StorageType::const_iterator ConstElementIterator
A constant random access iterator used to iterate over the elements of the array.
Definition: Array.hpp:125
bool isEmpty() const
Tells whether the array is empty (getSize() == 0).
Definition: Array.hpp:708
void swap(Array &array)
Swaps the contents with array.
Definition: Array.hpp:738
#define CDPL_UTIL_ARRAY_CHECK_ITER(it, allow_end)
Definition: Array.hpp:53
const ValueType & getFirstElement() const
Returns a const reference to the first element of the array.
Definition: Array.hpp:852
Array< STPair > STPairArray
An array of pairs of unsigned integers of type std::size_t.
Definition: Array.hpp:582
ElementIterator removeElement(const ElementIterator &it)
Removes the element at the position specified by the iterator it.
Definition: Array.hpp:831
Definition of exception classes.
bool operator<=(const Array< ValueType > &array1, const Array< ValueType > &array2)
Less or equal comparison operator.
const StorageType & getData() const
Definition: Array.hpp:678
ElementIterator getElementsEnd()
Returns a mutable iterator pointing to the end of the array.
Definition: Array.hpp:902
The namespace of the Chemical Data Processing Library.
ValueType & operator[](std::size_t idx)
Returns a non-const reference to the element at index idx.
Definition: Array.hpp:988
Array()
Creates an empty array.
Definition: Array.hpp:148
Array< std::size_t > STArray
An array of unsigned integers of type std::size_t.
Definition: Array.hpp:567
void insertElements(const ElementIterator &it, const InputIter &first, const InputIter &last)
Inserts the range of elements [first, last) before the location specified by the iterator it.
Definition: Array.hpp:806
ValueType & getFirstElement()
Returns a non-const reference to the first element of the array.
Definition: Array.hpp:868
void insertElements(std::size_t idx, const InputIter &first, const InputIter &last)
Inserts the range of elements [first, last) before the location specified by the index idx.
Definition: Array.hpp:797
std::size_t getCapacity() const
Returns the number of elements for which memory has been allocated.
Definition: Array.hpp:720
Array(std::size_t num_elem, const ValueType &value=ValueType())
Creates and initializes the array with num_elem copies of value.
Definition: Array.hpp:156
void assign(std::size_t num_elem, const ValueType &value=ValueType())
This function fills the array with num_elem copies of the given value.
Definition: Array.hpp:744
ValueType ElementType
The type of objects stored by the array.
Definition: Array.hpp:105
ElementIterator begin()
Returns a mutable iterator pointing to the beginning of the array.
Definition: Array.hpp:914
virtual ~Array()
Virtual destructor.
Definition: Array.hpp:172
ElementIterator insertElement(const ElementIterator &it, const ValueType &value=ValueType())
Inserts a new element before the location specified by the iterator it.
Definition: Array.hpp:771
const ValueType & getLastElement() const
Returns a const reference to the last element of the array.
Definition: Array.hpp:860
Array< double > DArray
An array of double precision floating-point numbers.
Definition: Array.hpp:587
BaseType & getBase()
Returns a non-const reference to itself.
Definition: Array.hpp:684
const BaseType & getBase() const
Returns a const reference to itself.
Definition: Array.hpp:690
Array< unsigned int > UIArray
An array of unsigned integers.
Definition: Array.hpp:562
bool operator>(const Array< ValueType > &array1, const Array< ValueType > &array2)
Greater than comparison operator.
void insertElement(std::size_t idx, const ValueType &value=ValueType())
Inserts a new element before the location specified by the index idx.
Definition: Array.hpp:763
#define CDPL_UTIL_ARRAY_CHECK_INDEX(idx, allow_end)
Definition: Array.hpp:45
bool operator==(const Array< ValueType > &array1, const Array< ValueType > &array2)
Equality comparison operator.
std::pair< std::size_t, std::size_t > STPair
A pair of unsigned integers of type std::size_t.
Definition: Array.hpp:577
StorageType::iterator ElementIterator
A mutable random access iterator used to iterate over the elements of the array.
Definition: Array.hpp:137
void removeElement(std::size_t idx)
Removes the element at the position specified by the index idx.
Definition: Array.hpp:823
std::shared_ptr< Array > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated Array instances.
Definition: Array.hpp:100
const ValueType & operator[](std::size_t idx) const
Returns a const reference to the element at index idx.
Definition: Array.hpp:980
ConstElementIterator begin() const
Returns a constant iterator pointing to the beginning of the array.
Definition: Array.hpp:908
void assign(const InputIter &first, const InputIter &last)
This function fills a vector with copies of the elements in the range [first, last).
Definition: Array.hpp:751
ConstReverseElementIterator getElementsReverseBegin() const
Returns a constant iterator pointing to the beginning of the reversed array.
Definition: Array.hpp:932
std::size_t size() const
Returns the number of elements stored in the array.
Definition: Array.hpp:702