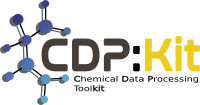 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
27 #ifndef CDPL_MATH_AFFINETRANSFORM_HPP
28 #define CDPL_MATH_AFFINETRANSFORM_HPP
49 class MatrixReference;
50 template <
typename T,
typename A>
52 template <
typename T,
typename A>
79 template <
typename T1,
typename T2,
typename T3,
typename T4>
89 std::copy(
m.data,
m.data + 4, data);
95 data[0] = q().getC1();
96 data[1] = q().getC2();
97 data[2] = q().getC3();
98 data[3] = q().getC4();
101 template <
typename T1,
typename T2,
typename T3,
typename T4>
102 void set(
const T1& w,
const T2& ux,
const T3& uy,
const T4& uz)
104 data[0] = std::cos(w / 2.0);
105 data[1] = std::sin(w / 2.0) * ux;
106 data[2] = std::sin(w / 2.0) * uy;
107 data[3] = std::sin(w / 2.0) * uz;
114 if (i >= 3 || i >= size || j >= 3 || j >= size)
124 return (data[0] * data[0] + data[1] * data[1] - data[2] * data[2] - data[3] * data[3]);
128 return 2 * (data[1] * data[2] - data[0] * data[3]);
132 return 2 * (data[1] * data[3] + data[0] * data[2]);
143 return 2 * (data[1] * data[2] + data[0] * data[3]);
147 return (data[0] * data[0] - data[1] * data[1] + data[2] * data[2] - data[3] * data[3]);
151 return 2 * (data[2] * data[3] - data[0] * data[1]);
162 return 2 * (data[1] * data[3] - data[0] * data[2]);
166 return 2 * (data[2] * data[3] + data[0] * data[1]);
170 return (data[0] * data[0] - data[1] * data[1] - data[2] * data[2] + data[3] * data[3]);
198 return std::numeric_limits<SizeType>::max();
203 return std::numeric_limits<SizeType>::max();
209 std::copy(
m.data,
m.data + 4, data);
219 std::swap_ranges(data, data + 4,
m.data);
220 std::swap(size,
m.size);
241 template <
typename T>
268 std::copy(
m.data,
m.data + 3, data);
286 if (i < size && i < 3)
309 return std::numeric_limits<SizeType>::max();
314 return std::numeric_limits<SizeType>::max();
320 std::copy(
m.data,
m.data + 3, data);
330 std::swap_ranges(data, data + 3,
m.data);
331 std::swap(size,
m.size);
352 template <
typename T>
379 std::copy(
m.data,
m.data + 3, data);
397 if (j == (size - 1) && i < size && i < 3)
420 return std::numeric_limits<SizeType>::max();
425 return std::numeric_limits<SizeType>::max();
431 std::copy(
m.data,
m.data + 3, data);
441 std::swap_ranges(data, data + 3,
m.data);
442 std::swap(size,
m.size);
480 #endif // CDPL_MATH_AFFINETRANSFORM_HPP
SizeType getMaxSize1() const
Definition: AffineTransform.hpp:196
Definition: Vector.hpp:258
ScalingMatrix & operator=(const ScalingMatrix &m)
Definition: AffineTransform.hpp:317
const T ConstReference
Definition: AffineTransform.hpp:361
Definition: AffineTransform.hpp:354
const T ConstReference
Definition: AffineTransform.hpp:250
ScalingMatrix< float > FScalingMatrix
Definition: AffineTransform.hpp:463
MatrixReference< SelfType > ClosureType
Definition: AffineTransform.hpp:67
const T ConstReference
Definition: AffineTransform.hpp:64
RotationMatrix< double > DRotationMatrix
Definition: AffineTransform.hpp:469
const MatrixReference< const SelfType > ConstClosureType
Definition: AffineTransform.hpp:68
MatrixReference< SelfType > ClosureType
Definition: AffineTransform.hpp:364
T ValueType
Definition: AffineTransform.hpp:248
friend void swap(TranslationMatrix &m1, TranslationMatrix &m2)
Definition: AffineTransform.hpp:446
ScalingMatrix(const ScalingMatrix &m)
Definition: AffineTransform.hpp:265
std::ptrdiff_t DifferenceType
Definition: AffineTransform.hpp:252
ScalingMatrix< unsigned long > ULScalingMatrix
Definition: AffineTransform.hpp:466
RotationMatrix(SizeType n, const T1 &w, const T2 &ux, const T3 &uy, const T4 &uz)
Definition: AffineTransform.hpp:80
T ValueType
Definition: AffineTransform.hpp:359
void set(const T1 &w, const T2 &ux, const T3 &uy, const T4 &uz)
Definition: AffineTransform.hpp:102
TranslationMatrix< float > FTranslationMatrix
Definition: AffineTransform.hpp:473
bool isEmpty() const
Definition: AffineTransform.hpp:403
const MatrixReference< const SelfType > ConstClosureType
Definition: AffineTransform.hpp:365
SizeType getSize2() const
Definition: AffineTransform.hpp:191
ConstReference operator()(SizeType i, SizeType j) const
Definition: AffineTransform.hpp:110
Vector< T, std::vector< T > > VectorTemporaryType
Definition: AffineTransform.hpp:256
friend void swap(ScalingMatrix &m1, ScalingMatrix &m2)
Definition: AffineTransform.hpp:335
Definition: Matrix.hpp:60
void set(const ValueType &sx=ValueType(1), const ValueType &sy=ValueType(1), const ValueType &sz=ValueType(1))
Definition: AffineTransform.hpp:271
Definition: AffineTransform.hpp:57
std::size_t SizeType
Definition: AffineTransform.hpp:251
void resize(SizeType n)
Definition: AffineTransform.hpp:451
RotationMatrix< long > LRotationMatrix
Definition: AffineTransform.hpp:470
const T Reference
Definition: AffineTransform.hpp:63
SizeType getMaxSize1() const
Definition: AffineTransform.hpp:307
#define CDPL_MATH_CHECK(expr, msg, e)
Definition: Check.hpp:36
const T Reference
Definition: AffineTransform.hpp:249
Definition: AffineTransform.hpp:243
void swap(ScalingMatrix &m)
Definition: AffineTransform.hpp:327
Matrix< T, std::vector< T > > MatrixTemporaryType
Definition: AffineTransform.hpp:69
Definition: Expression.hpp:98
void set(const QuaternionExpression< E > &q)
Definition: AffineTransform.hpp:93
T ValueType
Definition: AffineTransform.hpp:62
SizeType getMaxSize2() const
Definition: AffineTransform.hpp:312
Thrown to indicate that an index is out of range.
Definition: Base/Exceptions.hpp:152
const MatrixReference< const SelfType > ConstClosureType
Definition: AffineTransform.hpp:254
TranslationMatrix< long > LTranslationMatrix
Definition: AffineTransform.hpp:475
TranslationMatrix & operator=(const TranslationMatrix &m)
Definition: AffineTransform.hpp:428
ScalingMatrix< long > LScalingMatrix
Definition: AffineTransform.hpp:465
SizeType getSize2() const
Definition: AffineTransform.hpp:413
bool isEmpty() const
Definition: AffineTransform.hpp:181
void swap(RotationMatrix &m)
Definition: AffineTransform.hpp:216
SizeType getMaxSize2() const
Definition: AffineTransform.hpp:423
RotationMatrix< unsigned long > ULRotationMatrix
Definition: AffineTransform.hpp:471
const unsigned int m
Specifies that the stereocenter has m configuration.
Definition: CIPDescriptor.hpp:116
Matrix< T, std::vector< T > > MatrixTemporaryType
Definition: AffineTransform.hpp:255
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
ScalingMatrix< double > DScalingMatrix
Definition: AffineTransform.hpp:464
Definition of exception classes.
SizeType getSize1() const
Definition: AffineTransform.hpp:408
RotationMatrix & operator=(const RotationMatrix &m)
Definition: AffineTransform.hpp:206
const T Reference
Definition: AffineTransform.hpp:360
void resize(SizeType n)
Definition: AffineTransform.hpp:229
std::size_t SizeType
Definition: AffineTransform.hpp:362
Vector< T, std::vector< T > > VectorTemporaryType
Definition: AffineTransform.hpp:70
Definition: Matrix.hpp:280
void resize(SizeType n)
Definition: AffineTransform.hpp:340
TranslationMatrix(const TranslationMatrix &m)
Definition: AffineTransform.hpp:376
The namespace of the Chemical Data Processing Library.
TranslationMatrix< double > DTranslationMatrix
Definition: AffineTransform.hpp:474
friend void swap(RotationMatrix &m1, RotationMatrix &m2)
Definition: AffineTransform.hpp:224
Vector< T, std::vector< T > > VectorTemporaryType
Definition: AffineTransform.hpp:367
std::ptrdiff_t DifferenceType
Definition: AffineTransform.hpp:363
SizeType getMaxSize1() const
Definition: AffineTransform.hpp:418
ConstReference operator()(SizeType i, SizeType j) const
Definition: AffineTransform.hpp:279
TranslationMatrix< unsigned long > ULTranslationMatrix
Definition: AffineTransform.hpp:476
void swap(TranslationMatrix &m)
Definition: AffineTransform.hpp:438
SizeType getSize2() const
Definition: AffineTransform.hpp:302
RotationMatrix(const RotationMatrix &m)
Definition: AffineTransform.hpp:86
RotationMatrix(SizeType n, const QuaternionExpression< E > &q)
Definition: AffineTransform.hpp:73
ScalingMatrix(SizeType n, const ValueType &sx=ValueType(1), const ValueType &sy=ValueType(1), const ValueType &sz=ValueType(1))
Definition: AffineTransform.hpp:258
RotationMatrix< float > FRotationMatrix
Definition: AffineTransform.hpp:468
void set(const ValueType &tx=ValueType(), const ValueType &ty=ValueType(), const ValueType &tz=ValueType())
Definition: AffineTransform.hpp:382
Definition of various preprocessor macros for error checking.
std::size_t SizeType
Definition: AffineTransform.hpp:65
SizeType getSize1() const
Definition: AffineTransform.hpp:297
Matrix< T, std::vector< T > > MatrixTemporaryType
Definition: AffineTransform.hpp:366
SizeType getMaxSize2() const
Definition: AffineTransform.hpp:201
TranslationMatrix(SizeType n, const ValueType &tx=ValueType(), const ValueType &ty=ValueType(), const ValueType &tz=ValueType())
Definition: AffineTransform.hpp:369
Definition of basic expression types.
MatrixReference< SelfType > ClosureType
Definition: AffineTransform.hpp:253
ConstReference operator()(SizeType i, SizeType j) const
Definition: AffineTransform.hpp:390
bool isEmpty() const
Definition: AffineTransform.hpp:292
SizeType getSize1() const
Definition: AffineTransform.hpp:186
Definition: Expression.hpp:164
std::ptrdiff_t DifferenceType
Definition: AffineTransform.hpp:66