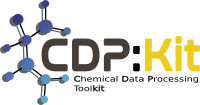 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_ANDMATCHEXPRESSIONLIST_HPP
30 #define CDPL_CHEM_ANDMATCHEXPRESSIONLIST_HPP
49 template <
typename ObjType1,
typename ObjType2 =
void>
70 bool operator()(
const ObjType1& query_obj1,
const ObjType2& query_obj2,
const ObjType1& target_obj1,
const ObjType2& target_obj2,
86 bool operator()(
const ObjType1& query_obj1,
const ObjType2& query_obj2,
const ObjType1& target_obj1,
const ObjType2& target_obj2,
90 const char* getClassName()
const
92 return "ANDMatchExpressionList";
100 template <
typename ObjType>
119 bool operator()(
const ObjType& query_obj,
const ObjType& target_obj,
const Base::Any& aux_data)
const;
136 const char* getClassName()
const
138 return "ANDMatchExpressionList";
147 template <
typename ObjType1,
typename ObjType2>
149 const ObjType1& target_obj1,
const ObjType2& target_obj2,
155 if (!(*it)(query_obj1, query_obj2, target_obj1, target_obj2, data))
161 template <
typename ObjType1,
typename ObjType2>
163 const ObjType1& target_obj1,
const ObjType2& target_obj2,
169 if (!(*it)(query_obj1, query_obj2, target_obj1, target_obj2, mapping, data))
176 template <
typename ObjType>
183 if (!(*it)(query_obj, target_obj, data))
189 template <
typename ObjType>
196 if (!(*it)(query_obj, target_obj, mapping, data))
202 #endif // CDPL_CHEM_ANDMATCHEXPRESSIONLIST_HPP
bool operator()(const ObjType1 &query_obj1, const ObjType2 &query_obj2, const ObjType1 &target_obj1, const ObjType2 &target_obj2, const Base::Any &aux_data) const
Performs an evaluation of the conjunctive expression list for the given query and target objects.
Definition: ANDMatchExpressionList.hpp:148
ConstElementIterator getElementsBegin() const
Returns a constant iterator over the pointed-to objects that points to the beginning of the array.
Definition: IndirectArray.hpp:448
std::shared_ptr< ANDMatchExpressionList > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated ANDMatchExpressionList instances.
Definition: ANDMatchExpressionList.hpp:57
A safe, type checked container for arbitrary data of variable type.
Definition: Any.hpp:59
ConstElementIterator getElementsEnd() const
Returns a constant iterator over the pointed-to objects that points to the end of the array.
Definition: IndirectArray.hpp:462
StorageType::const_iterator ConstElementIterator
A constant random access iterator used to iterate over the elements of the array.
Definition: Array.hpp:125
std::shared_ptr< ANDMatchExpressionList > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated ANDMatchExpressionList instances.
Definition: ANDMatchExpressionList.hpp:108
A container for the storage and evaluation of logical match expression lists.
Definition: MatchExpressionList.hpp:61
The namespace of the Chemical Data Processing Library.
ANDMatchExpressionList.
Definition: ANDMatchExpressionList.hpp:51
Definition of the class CDPL::Chem::MatchExpressionList.
A data structure for the common storage of related atom to atom and bond to bond mappings.
Definition: AtomBondMapping.hpp:55