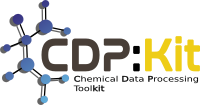 |
Chemical Data Processing Library C++ API - Version 1.1.0
|
Go to the documentation of this file.
29 #ifndef CDPL_FORCEFIELD_MMFF94VANDERWAALSINTERACTION_HPP
30 #define CDPL_FORCEFIELD_MMFF94VANDERWAALSINTERACTION_HPP
57 double atom_pol1,
double eff_el_num1,
double fact_a1,
double fact_g1,
60 double expo,
double fact_b,
double beta,
double fact_darad,
double fact_daeps):
65 bool have_don =
false;
66 bool have_don_acc =
false;
68 switch (don_acc_type1) {
72 have_don_acc = (don_acc_type2 == ACCEPTOR);
76 have_don_acc = (don_acc_type2 == DONOR);
77 have_don = have_don_acc;
81 have_don = (don_acc_type2 == DONOR);
85 double rII = fact_a1 * std::pow(atom_pol1, expo);
86 double rJJ = fact_a2 * std::pow(atom_pol2, expo);
87 double gIJ = (rII - rJJ) / (rII + rJJ);
89 rIJ = 0.5 * (rII + rJJ);
92 rIJ += rIJ * fact_b * (1.0 - std::exp(-beta * gIJ * gIJ));
94 eIJ = 181.16 * fact_g1 * fact_g2 * atom_pol1 * atom_pol2 / (std::pow(atom_pol1 / eff_el_num1, 0.5) + std::pow(atom_pol2 / eff_el_num2, 0.5)) * std::pow(rIJ, -6.0);
101 rIJPow7 = std::pow(rIJ, 7.0);
130 std::size_t atom1Idx;
131 std::size_t atom2Idx;
139 #endif // CDPL_FORCEFIELD_MMFF94VANDERWAALSINTERACTION_HPP
Definition of the preprocessor macro CDPL_FORCEFIELD_API.
@ DONOR
Definition: MMFF94VanDerWaalsInteraction.hpp:52
std::size_t getAtom2Index() const
Definition: MMFF94VanDerWaalsInteraction.hpp:109
MMFF94VanDerWaalsInteraction(std::size_t atom1_idx, std::size_t atom2_idx, double atom_pol1, double eff_el_num1, double fact_a1, double fact_g1, HDonorAcceptorType don_acc_type1, double atom_pol2, double eff_el_num2, double fact_a2, double fact_g2, HDonorAcceptorType don_acc_type2, double expo, double fact_b, double beta, double fact_darad, double fact_daeps)
Definition: MMFF94VanDerWaalsInteraction.hpp:56
double getEIJ() const
Definition: MMFF94VanDerWaalsInteraction.hpp:114
double getRIJ() const
Definition: MMFF94VanDerWaalsInteraction.hpp:119
std::size_t getAtom1Index() const
Definition: MMFF94VanDerWaalsInteraction.hpp:104
Definition: MMFF94VanDerWaalsInteraction.hpp:45
HDonorAcceptorType
Definition: MMFF94VanDerWaalsInteraction.hpp:49
The namespace of the Chemical Data Processing Library.
@ NONE
Definition: MMFF94VanDerWaalsInteraction.hpp:51
#define CDPL_FORCEFIELD_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
double getRIJPow7() const
Definition: MMFF94VanDerWaalsInteraction.hpp:124