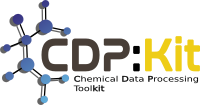 |
Chemical Data Processing Library C++ API - Version 1.0.0
|
Go to the documentation of this file.
29 #ifndef CDPL_UTIL_OBJECTSTACK_HPP
30 #define CDPL_UTIL_OBJECTSTACK_HPP
83 maxSize(stack.maxSize), freeIndex(0), ctorFunc(stack.ctorFunc), dtorFunc(stack.dtorFunc),
84 initFunc(stack.initFunc), cleanFunc(stack.cleanFunc) {}
87 maxSize(max_pool_size), freeIndex(0), ctorFunc(DefaultConstructor()), dtorFunc(DefaultDestructor()) {}
89 template <
typename C,
typename D>
90 ObjectStack(
const C& ctor_func,
const D& dtor_func, std::size_t max_pool_size = 0):
91 maxSize(max_pool_size), freeIndex(0), ctorFunc(ctor_func), dtorFunc(dtor_func)
103 if (freeIndex == allocObjects.size()) {
107 throw std::bad_alloc();
109 allocObjects.push_back(obj_ptr);
126 cleanFunc(*allocObjects[freeIndex]);
129 if (maxSize > 0 && freeIndex <= maxSize && allocObjects.size() > maxSize)
130 allocObjects.resize(maxSize);
136 std::for_each(allocObjects.begin(), allocObjects.begin() + freeIndex,
138 std::placeholders::_1)));
141 if (maxSize > 0 && allocObjects.size() > maxSize)
142 allocObjects.resize(maxSize);
154 if (maxSize > 0 && allocObjects.size() > maxSize)
155 allocObjects.resize(std::max(freeIndex, maxSize));
161 allocObjects.resize(freeIndex);
166 allocObjects.clear();
184 maxSize = stack.maxSize;
185 ctorFunc = stack.ctorFunc;
186 dtorFunc = stack.dtorFunc;
187 initFunc = stack.initFunc;
189 if (maxSize > 0 && allocObjects.size() > maxSize)
190 allocObjects.resize(std::max(freeIndex, maxSize));
196 typedef std::vector<SharedObjectPointer> AllocObjectList;
199 std::size_t freeIndex;
200 AllocObjectList allocObjects;
209 #endif // CDPL_UTIL_OBJECTSTACK_HPP
ObjectStack(const C &ctor_func, const D &dtor_func, std::size_t max_pool_size=0)
Definition: ObjectStack.hpp:90
void setCleanupFunction(const ObjectFunction &func)
Definition: ObjectStack.hpp:174
void put()
Definition: ObjectStack.hpp:120
T * operator()() const
Definition: ObjectStack.hpp:67
void freeMemory(bool unused_only=true)
Definition: ObjectStack.hpp:158
ObjectStack(const ObjectStack &stack)
Definition: ObjectStack.hpp:82
~ObjectStack()
Definition: ObjectStack.hpp:94
const SharedObjectPointer & get()
Definition: ObjectStack.hpp:101
An unary functor for the dereferenciation of pointers without null pointer checking.
Definition: Dereferencer.hpp:52
std::function< void(ObjectType *)> DestructorFunction
Definition: ObjectStack.hpp:61
void setMaxPoolSize(std::size_t max_size)
Definition: ObjectStack.hpp:150
void putAll()
Definition: ObjectStack.hpp:133
ObjectType * getRaw()
Definition: ObjectStack.hpp:96
void operator()(T *obj) const
Definition: ObjectStack.hpp:76
std::shared_ptr< ObjectType > SharedObjectPointer
Definition: ObjectStack.hpp:58
Definition: ObjectStack.hpp:74
ObjectStack & operator=(const ObjectStack &stack)
Definition: ObjectStack.hpp:179
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
The namespace of the Chemical Data Processing Library.
T ObjectType
Definition: ObjectStack.hpp:56
Definition: ObjectStack.hpp:65
std::function< void(ObjectType &)> ObjectFunction
Definition: ObjectStack.hpp:62
Definition of the classes CDPL::Util::Dereferencer and CDPL::Util::NullCheckDereferencer.
const unsigned int D
Specifies Hydrogen (Deuterium).
Definition: AtomType.hpp:62
ObjectStack.
Definition: ObjectStack.hpp:53
std::size_t getMaxPoolSize() const
Definition: ObjectStack.hpp:145
std::function< ObjectType *()> ConstructorFunction
Definition: ObjectStack.hpp:60
ObjectStack(std::size_t max_pool_size=0)
Definition: ObjectStack.hpp:86
const unsigned int C
Specifies Carbon.
Definition: AtomType.hpp:92
void setInitFunction(const ObjectFunction &func)
Definition: ObjectStack.hpp:169