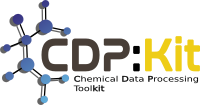 |
Chemical Data Processing Library C++ API - Version 1.0.0
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_MAXCOMMONBONDSUBSTRUCTURESEARCH_HPP
30 #define CDPL_CHEM_MAXCOMMONBONDSUBSTRUCTURESEARCH_HPP
36 #include <boost/iterator/indirect_iterator.hpp>
62 typedef std::vector<AtomBondMapping*> ABMappingList;
68 typedef boost::indirect_iterator<ABMappingList::iterator, AtomBondMapping>
MappingIterator;
73 typedef boost::indirect_iterator<ABMappingList::const_iterator, const AtomBondMapping>
ConstMappingIterator;
268 void initMatchExpressions();
270 bool findEquivAtoms();
272 bool atomsCompatible(
const Bond&,
const Bond&)
const;
274 const Atom* getCommonAtom(
const Bond&,
const Bond&)
const;
276 bool buildAssocGraph();
278 bool findAssocGraphCliques(std::size_t);
279 bool isLegal(
const AGNode*);
281 void undoAtomMapping(std::size_t);
285 bool hasPostMappingMatchExprs()
const;
288 bool foundMappingUnique(
bool);
290 void clearMappings();
293 void freeAtomBondMapping();
295 void freeAssocGraph();
296 void freeAtomBondMappings();
300 AGNode* allocAGNode(
const Bond*,
const Bond*);
301 AGEdge* allocAGEdge(
const Atom*,
const Atom*);
303 typedef std::vector<const AGEdge*> AGraphEdgeList;
309 void setQueryBond(
const Bond*);
310 const Bond* getQueryBond()
const;
312 void setAssocBond(
const Bond*);
313 const Bond* getAssocBond()
const;
315 void addEdge(
const AGEdge*);
317 bool isConnected(
const AGNode*)
const;
318 const AGEdge* findEdge(
const AGNode*)
const;
322 void setIndex(std::size_t idx);
326 const Bond* queryBond;
327 const Bond* assocBond;
329 AGraphEdgeList atomEdges;
336 void setQueryAtom(
const Atom*);
337 const Atom* getQueryAtom()
const;
339 void setAssocAtom(
const Atom*);
340 const Atom* getAssocAtom()
const;
342 void setNode1(
const AGNode*);
343 void setNode2(
const AGNode*);
345 const AGNode* getNode1()
const;
346 const AGNode* getNode2()
const;
348 const AGNode* getOther(
const AGNode*)
const;
351 const Atom* queryAtom;
352 const Atom* assocAtom;
361 void initQueryAtomMask(std::size_t);
362 void initTargetAtomMask(std::size_t);
364 void initQueryBondMask(std::size_t);
365 void initTargetBondMask(std::size_t);
367 void setQueryAtomBit(std::size_t);
368 void setTargetAtomBit(std::size_t);
370 void resetQueryAtomBit(std::size_t);
371 void resetTargetAtomBit(std::size_t);
373 bool testQueryAtomBit(std::size_t)
const;
374 bool testTargetAtomBit(std::size_t)
const;
376 void setQueryBondBit(std::size_t);
377 void setTargetBondBit(std::size_t);
379 void resetQueryAtomBits();
380 void resetTargetAtomBits();
382 void resetBondBits();
384 bool operator<(
const ABMappingMask&)
const;
385 bool operator>(
const ABMappingMask&)
const;
396 typedef std::vector<Util::BitSet> BitMatrix;
397 typedef std::vector<AGNode*> AGraphNodeList;
398 typedef std::vector<AGraphNodeList> AGraphNodeMatrix;
399 typedef std::set<ABMappingMask> UniqueMappingList;
400 typedef std::vector<const Atom*> AtomList;
401 typedef std::vector<const Bond*> BondList;
402 typedef std::vector<MatchExpression<Atom, MolecularGraph>::SharedPointer> AtomMatchExprTable;
403 typedef std::vector<MatchExpression<Bond, MolecularGraph>::SharedPointer> BondMatchExprTable;
404 typedef Util::ObjectStack<AGNode> NodeCache;
405 typedef Util::ObjectStack<AGEdge> EdgeCache;
406 typedef Util::ObjectStack<AtomBondMapping> MappingCache;
408 const MolecularGraph* query;
409 const MolecularGraph* target;
410 BitMatrix atomEquivMatrix;
411 AGraphNodeMatrix nodeMatrix;
412 ABMappingList foundMappings;
413 UniqueMappingList uniqueMappings;
414 AGraphEdgeList cliqueEdges;
415 AGraphNodeList cliqueNodes;
416 ABMappingMask mappingMask;
417 AtomMatchExprTable atomMatchExprTable;
418 BondMatchExprTable bondMatchExprTable;
419 MolGraphMatchExprPtr molGraphMatchExpr;
420 AtomList postMappingMatchAtoms;
421 BondList postMappingMatchBonds;
424 MappingCache mappingCache;
429 std::size_t numQueryAtoms;
430 std::size_t numQueryBonds;
431 std::size_t numTargetAtoms;
432 std::size_t numTargetBonds;
433 std::size_t maxBondSubstructureSize;
434 std::size_t currNumNullNodes;
435 std::size_t minNumNullNodes;
436 std::size_t maxNumMappings;
437 std::size_t minSubstructureSize;
438 std::size_t currNodeIdx;
443 #endif // CDPL_CHEM_MAXCOMMONBONDSUBSTRUCTURESEARCH_HPP
std::size_t getMinSubstructureSize() const
Returns the minimum accepted common substructure size.
void uniqueMappingsOnly(bool unique)
Allows to specify whether or not to store only unique atom/bond mappings.
Definition of the class CDPL::Util::ObjectStack.
MaxCommonBondSubstructureSearch.
Definition: MaxCommonBondSubstructureSearch.hpp:60
MaxCommonBondSubstructureSearch(const MolecularGraph &query)
Constructs and initializes a MaxCommonBondSubstructureSearch instance for the specified query structu...
Definition of the preprocessor macro CDPL_CHEM_API.
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Bond.
Definition: Bond.hpp:50
MaxCommonBondSubstructureSearch()
Constructs and initializes a MaxCommonBondSubstructureSearch instance.
MappingIterator end()
Returns a mutable iterator pointing to the end of the stored atom/bond mapping objects.
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
bool mappingExists(const MolecularGraph &target)
Searches for a common substructure between the query and the specified target molecular graph.
Atom.
Definition: Atom.hpp:52
std::size_t getNumMappings() const
Returns the number of atom/bond mappings that were recorded in the last search for common substructur...
boost::indirect_iterator< ABMappingList::iterator, AtomBondMapping > MappingIterator
A mutable random access iterator used to iterate over the stored atom/bond mapping objects.
Definition: MaxCommonBondSubstructureSearch.hpp:68
const AtomBondMapping & getMapping(std::size_t idx) const
Returns a const reference to the stored atom/bond mapping object at index idx.
MolecularGraph.
Definition: MolecularGraph.hpp:52
Definition of the type CDPL::Util::BitSet.
boost::indirect_iterator< ABMappingList::const_iterator, const AtomBondMapping > ConstMappingIterator
A constant random access iterator used to iterate over the stored atom/bond mapping objects.
Definition: MaxCommonBondSubstructureSearch.hpp:73
MappingIterator getMappingsBegin()
Returns a mutable iterator pointing to the beginning of the stored atom/bond mapping objects.
bool operator<(const Array< ValueType > &array1, const Array< ValueType > &array2)
Less than comparison operator.
ConstMappingIterator end() const
Returns a constant iterator pointing to the end of the stored atom/bond mapping objects.
bool findMappings(const MolecularGraph &target)
Searches for all atom/bond mappings of query subgraphs to substructures of the specified target molec...
std::shared_ptr< MatchExpression > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated MatchExpression instances.
Definition: MatchExpression.hpp:81
Definition of the class CDPL::Chem::AtomBondMapping.
void setMaxNumMappings(std::size_t max_num_mappings)
Allows to specify a limit on the number of stored atom/bond mappings.
MappingIterator begin()
Returns a mutable iterator pointing to the beginning of the stored atom/bond mapping objects.
void setMinSubstructureSize(std::size_t min_size)
Allows to specify the minimum accepted common substructure size.
ConstMappingIterator begin() const
Returns a constant iterator pointing to the beginning of the stored atom/bond mapping objects.
Definition of the class CDPL::Chem::MatchExpression.
The namespace of the Chemical Data Processing Library.
MappingIterator getMappingsEnd()
Returns a mutable iterator pointing to the end of the stored atom/bond mapping objects.
bool uniqueMappingsOnly() const
Tells whether duplicate atom/bond mappings are discarded.
~MaxCommonBondSubstructureSearch()
Destructor.
ConstMappingIterator getMappingsEnd() const
Returns a constant iterator pointing to the end of the stored atom/bond mapping objects.
A data structure for the common storage of related atom to atom and bond to bond mappings.
Definition: AtomBondMapping.hpp:55
bool operator>(const Array< ValueType > &array1, const Array< ValueType > &array2)
Greater than comparison operator.
std::size_t getMaxNumMappings() const
Returns the specified limit on the number of stored atom/bond mappings.
void setQuery(const MolecularGraph &query)
Allows to specify a new query structure.
AtomBondMapping & getMapping(std::size_t idx)
Returns a non-const reference to the stored atom/bond mapping object at index idx.
ConstMappingIterator getMappingsBegin() const
Returns a constant iterator pointing to the beginning of the stored atom/bond mapping objects.