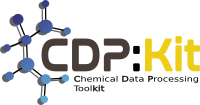 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_TOPOLOGICALENTITYALIGNMENT_HPP
30 #define CDPL_CHEM_TOPOLOGICALENTITYALIGNMENT_HPP
36 #include <boost/iterator/indirect_iterator.hpp>
70 typedef boost::indirect_iterator<typename EntitySet::const_iterator, const EntityType>
ConstEntityIterator;
174 typedef std::vector<Util::STPair> CompatGraphNodeArray;
179 CompatGraphNodeArray compatGraphNodes;
192 template <
typename T>
195 entityMatchFunc = func;
199 template <
typename T>
203 return entityMatchFunc;
206 template <
typename T>
209 entityPairMatchFunc = func;
213 template <
typename T>
217 return entityPairMatchFunc;
220 template <
typename T>
223 return (first_set ? firstEntities : secondEntities).size();
226 template <
typename T>
229 (first_set ? firstEntities : secondEntities).push_back(&entity);
233 template <
typename T>
236 (first_set ? firstEntities : secondEntities).clear();
240 template <
typename T>
244 return (first_set ? firstEntities : secondEntities).begin();
247 template <
typename T>
251 return (first_set ? firstEntities : secondEntities).end();
254 template <
typename T>
258 const EntitySet& entity_set = (first_set ? firstEntities : secondEntities);
260 if (idx >= entity_set.size())
261 throw Base::IndexError(
"TopologicalEntityAlignment: entity index out of bounds");
263 return *entity_set[idx];
266 template <
typename T>
269 compatGraphNodes.clear();
271 if (entityMatchFunc) {
274 for (
typename EntitySet::const_iterator it1 = firstEntities.begin(), end1 = firstEntities.end(); it1 != end1; ++it1, i++) {
275 const EntityType* ent1 = *it1;
278 for (
typename EntitySet::const_iterator it2 = secondEntities.begin(), end2 = secondEntities.end(); it2 != end2; ++it2, j++)
279 if (entityMatchFunc(*ent1, **it2))
284 for (std::size_t i = 0, num_ents1 = firstEntities.size(); i < num_ents1; i++)
285 for (std::size_t j = 0, num_ents2 = secondEntities.size(); j < num_ents2; j++)
289 std::size_t num_nodes = compatGraphNodes.size();
291 adjMatrix.resize(num_nodes);
293 for (std::size_t i = 0; i < num_nodes; i++) {
294 adjMatrix[i].resize(num_nodes);
295 adjMatrix[i].
reset();
298 for (std::size_t i = 0; i < num_nodes; i++) {
301 for (std::size_t j = i + 1; j < num_nodes; j++) {
304 if (p1.first == p2.first)
307 if (p1.second == p2.second)
310 if (!entityPairMatchFunc || entityPairMatchFunc(*firstEntities[p1.first], *firstEntities[p2.first],
311 *secondEntities[p1.second], *secondEntities[p2.second])) {
318 bkAlgorithm.init(adjMatrix);
323 template <
typename T>
329 if (!bkAlgorithm.nextClique(clique))
334 for (std::size_t i = clique.find_first(); i != Util::BitSet::npos; i = clique.find_next(i))
340 template <
typename T>
346 #endif // CDPL_CHEM_TOPOLOGICALENTITYALIGNMENT_HPP
std::function< bool(const EntityType &, const EntityType &, const EntityType &, const EntityType &)> EntityPairMatchFunction
A generic wrapper class used to store a user-defined entity-pair match constraint function.
Definition: TopologicalEntityAlignment.hpp:80
Implementation of the Bron-Kerbosch algorithm.
TopologicalEntityAlignment()
Constructs the TopologicalEntityAlignment instance.
Definition: TopologicalEntityAlignment.hpp:85
void reset()
Definition: TopologicalEntityAlignment.hpp:341
T EntityType
The actual entity type.
Definition: TopologicalEntityAlignment.hpp:60
const EntityMatchFunction & getEntityMatchFunction() const
Returns the function that was registered for restricting allowed entity mappings.
Definition: TopologicalEntityAlignment.hpp:201
TopologicalEntityAlignment.
Definition: TopologicalEntityAlignment.hpp:54
const EntityPairMatchFunction & getEntityPairMatchFunction() const
Returns the function that was registered for checking the compatibility of entity-pairs.
Definition: TopologicalEntityAlignment.hpp:215
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
std::vector< const EntityType * > EntitySet
The container storing the entities to align.
Definition: TopologicalEntityAlignment.hpp:65
A dynamic array class providing amortized constant time access to arbitrary elements.
Definition: Array.hpp:92
std::function< bool(const EntityType &, const EntityType &)> EntityMatchFunction
A generic wrapper class used to store a user-defined entity match constraint function.
Definition: TopologicalEntityAlignment.hpp:75
ConstEntityIterator getEntitiesBegin(bool first_set) const
Returns a constant iterator pointing to the beginning of the entities stored in the specified set.
Definition: TopologicalEntityAlignment.hpp:242
Implementation of the Bron-Kerbosch clique-detection algorithm [BKA].
Definition: BronKerboschAlgorithm.hpp:49
void setEntityPairMatchFunction(const EntityPairMatchFunction &func)
Specifies a function for checking the compatibility of entity-pairs in the search for alignment solut...
Definition: TopologicalEntityAlignment.hpp:207
void clear()
Erases all elements.
Definition: Array.hpp:732
Thrown to indicate that an index is out of range.
Definition: Base/Exceptions.hpp:152
Definition of the class CDPL::Util::Array.
const EntityType & getEntity(std::size_t idx, bool first_set) const
Returns a non-const reference to the stored entity at index idx in the specified set.
Definition: TopologicalEntityAlignment.hpp:256
void addElement(const ValueType &value=ValueType())
Inserts a new element at the end of the array.
Definition: Array.hpp:757
bool nextAlignment(Util::STPairArray &mapping)
Searches for the next alignment solution and stores the corresponding mapping of the entities in the ...
Definition: TopologicalEntityAlignment.hpp:324
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
Definition of exception classes.
void addEntity(const EntityType &entity, bool first_set)
Adds an entity to the specified alignment entity set.
Definition: TopologicalEntityAlignment.hpp:227
The namespace of the Chemical Data Processing Library.
std::size_t getNumEntities(bool first_set) const
Returns the number of entities in the specified alignment entity set.
Definition: TopologicalEntityAlignment.hpp:221
ConstEntityIterator getEntitiesEnd(bool first_set) const
Returns a constant iterator pointing to the end of the entities stored in the specified set.
Definition: TopologicalEntityAlignment.hpp:249
boost::indirect_iterator< typename EntitySet::const_iterator, const EntityType > ConstEntityIterator
A constant iterator over the stored entities.
Definition: TopologicalEntityAlignment.hpp:70
void setEntityMatchFunction(const EntityMatchFunction &func)
Specifies a function for restricting allowed entity mappings in the search for alignment solutions.
Definition: TopologicalEntityAlignment.hpp:193
void clearEntities(bool first_set)
Removes all entities in the specified alignment entity set.
Definition: TopologicalEntityAlignment.hpp:234
std::pair< std::size_t, std::size_t > STPair
A pair of unsigned integers of type std::size_t.
Definition: Array.hpp:577
virtual ~TopologicalEntityAlignment()
Virtual destructor.
Definition: TopologicalEntityAlignment.hpp:91