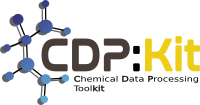 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_SURFACEATOMEXTRACTOR_HPP
30 #define CDPL_CHEM_SURFACEATOMEXTRACTOR_HPP
59 static constexpr
double DEF_PROBE_RADIUS = 1.2;
60 static constexpr
double DEF_GRID_OVERSIZE = 5.0;
61 static constexpr
double DEF_GRID_STEP_SIZE = 0.75;
62 static constexpr
double DEF_MIN_SURFACE_ACC = 0.01;
63 static constexpr std::size_t DEF_NUM_TEST_POINTS = 250;
169 typedef std::vector<double> AtomRadiusTable;
170 typedef std::vector<std::size_t> AtomIndexList;
171 typedef std::shared_ptr<AtomIndexList> AtomIndexListPtr;
172 typedef std::vector<AtomIndexListPtr> GridAtomLookupTable;
177 void transformCoordinates();
179 void initGridAtomLookupTable();
180 void extractSurfaceAtoms(
Fragment& frag);
182 void initTestPoints();
188 std::size_t numTestPoints;
192 AtomRadiusTable atomRadii;
193 AtomIndexList atomIndices;
195 Vector3DArray atomCoords;
196 Vector3DArray testPoints;
199 std::size_t gridXSize;
200 std::size_t gridYSize;
201 std::size_t gridZSize;
202 GridAtomLookupTable gridAtomLookup;
207 #endif // CDPL_CHEM_SURFACEATOMEXTRACTOR_HPP
Definition of the preprocessor macro CDPL_CHEM_API.
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
CVector< double, 3 > Vector3D
A bounded 3 element vector holding floating point values of type double.
Definition: Vector.hpp:1637
Fragment.
Definition: Fragment.hpp:52
MolecularGraph.
Definition: MolecularGraph.hpp:52
Type definition of a generic wrapper class for storing user-defined Chem::Atom 3D-coordinates functio...
A common interface for data-structures that support a random access to stored Chem::Atom instances.
Definition: AtomContainer.hpp:55
VectorArray< Vector3D > Vector3DArray
An array of Math::Vector3D objects.
Definition: VectorArray.hpp:84
std::function< const Math::Vector3D &(const Chem::Atom &)> Atom3DCoordinatesFunction
A generic wrapper class used to store a user-defined Chem::Atom 3D-coordinates function.
Definition: Atom3DCoordinatesFunction.hpp:43
The namespace of the Chemical Data Processing Library.
Definition of matrix data types.
CDPL_CHEM_API void calcBoundingBox(const AtomContainer &cntnr, Math::Vector3D &min, Math::Vector3D &max, const Atom3DCoordinatesFunction &coords_func, bool reset=true)
Definition of vector data types.