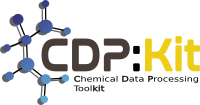 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_SUBSTRUCTURESEARCH_HPP
30 #define CDPL_CHEM_SUBSTRUCTURESEARCH_HPP
36 #include <unordered_map>
40 #include <boost/iterator/indirect_iterator.hpp>
66 typedef std::vector<AtomBondMapping*> ABMappingList;
78 typedef boost::indirect_iterator<ABMappingList::iterator, AtomBondMapping>
MappingIterator;
83 typedef boost::indirect_iterator<ABMappingList::const_iterator, const AtomBondMapping>
ConstMappingIterator;
309 void initMatchExpressions();
311 bool findEquivAtoms();
312 bool findEquivBonds();
316 std::size_t nextQueryAtom()
const;
317 bool nextTargetAtom(std::size_t, std::size_t&, std::size_t&)
const;
319 bool atomMappingAllowed(std::size_t, std::size_t)
const;
320 bool checkAtomMappingConstraints(std::size_t, std::size_t)
const;
321 bool checkBondMappingConstraints(std::size_t, std::size_t)
const;
323 bool mapBonds(std::size_t, std::size_t);
324 bool mapAtoms(std::size_t);
325 bool mapAtoms(std::size_t, std::size_t);
329 bool hasPostMappingMatchExprs()
const;
332 bool foundMappingUnique();
334 void freeAtomBondMappings();
335 void freeAtomBondMapping();
343 void initAtomMask(std::size_t);
344 void initBondMask(std::size_t);
346 void setAtomBit(std::size_t);
347 void resetAtomBit(std::size_t);
349 bool testAtomBit(std::size_t)
const;
351 void setBondBit(std::size_t);
352 void resetBondMask();
354 bool operator<(
const ABMappingMask&)
const;
355 bool operator>(
const ABMappingMask&)
const;
362 typedef std::vector<Util::BitSet> BitMatrix;
363 typedef std::vector<const Atom*> AtomMappingTable;
364 typedef std::vector<const Bond*> BondMappingTable;
365 typedef std::deque<std::size_t> AtomQueue;
366 typedef std::set<ABMappingMask> UniqueMappingList;
367 typedef std::vector<const Atom*> AtomList;
368 typedef std::vector<const Bond*> BondList;
369 typedef std::vector<AtomMatchExprPtr> AtomMatchExprTable;
370 typedef std::vector<BondMatchExprPtr> BondMatchExprTable;
371 typedef std::unordered_multimap<std::size_t, std::size_t> MappingConstraintMap;
376 AtomMatchExpressionFunction atomMatchExprFunc;
377 BondMatchExpressionFunction bondMatchExprFunc;
378 MolecularGraphMatchExpressionFunction molGraphMatchExprFunc;
379 BitMatrix atomEquivMatrix;
380 BitMatrix bondEquivMatrix;
381 MappingConstraintMap atomMappingConstrs;
382 MappingConstraintMap bondMappingConstrs;
383 AtomQueue termQueryAtoms;
384 AtomMappingTable queryAtomMapping;
385 BondMappingTable queryBondMapping;
387 ABMappingMask targetMappingMask;
388 ABMappingList foundMappings;
389 UniqueMappingList uniqueMappings;
390 AtomMatchExprTable atomMatchExprTable;
391 BondMatchExprTable bondMatchExprTable;
392 MolGraphMatchExprPtr molGraphMatchExpr;
393 AtomList postMappingMatchAtoms;
394 BondList postMappingMatchBonds;
395 MappingCache mappingCache;
401 std::size_t numQueryAtoms;
402 std::size_t numQueryBonds;
403 std::size_t numTargetAtoms;
404 std::size_t numTargetBonds;
405 std::size_t numMappedAtoms;
406 std::size_t maxNumMappings;
411 #endif // CDPL_CHEM_SUBSTRUCTURESEARCH_HPP
Definition of the class CDPL::Util::ObjectStack.
void setQuery(const MolecularGraph &query)
Allows to specify a new query structure.
ConstMappingIterator end() const
Returns a constant iterator pointing to the end of the stored atom/bond mapping objects.
SubstructureSearch(const MolecularGraph &query)
Constructs and initializes a SubstructureSearch instance for the specified query structure.
Definition of the preprocessor macro CDPL_CHEM_API.
ConstMappingIterator getMappingsBegin() const
Returns a constant iterator pointing to the beginning of the stored atom/bond mapping objects.
void setBondMatchExpressionFunction(const BondMatchExpressionFunction &func)
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Bond.
Definition: Bond.hpp:50
const AtomBondMapping & getMapping(std::size_t idx) const
Returns a const reference to the stored atom/bond mapping object at index idx.
SubstructureSearch.
Definition: SubstructureSearch.hpp:64
SubstructureSearch()
Constructs and initializes a SubstructureSearch instance.
std::size_t getMaxNumMappings() const
Returns the specified limit on the number of stored atom/bond mappings.
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
Atom.
Definition: Atom.hpp:52
void clearAtomMappingConstraints()
Clears all previously defined query to target atom mapping constraints.
MappingIterator end()
Returns a mutable iterator pointing to the end of the stored atom/bond mapping objects.
MappingIterator begin()
Returns a mutable iterator pointing to the beginning of the stored atom/bond mapping objects.
MolecularGraph.
Definition: MolecularGraph.hpp:52
Definition of the type CDPL::Util::BitSet.
void addAtomMappingConstraint(std::size_t query_atom_idx, std::size_t target_atom_idx)
Adds a constraint on the allowed mappings between query and target structure atoms.
std::function< const MolGraphMatchExprPtr &(const MolecularGraph &)> MolecularGraphMatchExpressionFunction
Definition: SubstructureSearch.hpp:87
ConstMappingIterator begin() const
Returns a constant iterator pointing to the beginning of the stored atom/bond mapping objects.
void setMolecularGraphMatchExpressionFunction(const MolecularGraphMatchExpressionFunction &func)
bool operator<(const Array< ValueType > &array1, const Array< ValueType > &array2)
Less than comparison operator.
void uniqueMappingsOnly(bool unique)
Allows to specify whether or not to store only unique atom/bond mappings.
~SubstructureSearch()
Destructor.
bool findMappings(const MolecularGraph &target)
Searches for all possible atom/bond mappings of the query structure to substructures of the specified...
bool mappingExists(const MolecularGraph &target)
Tells whether the query structure matches a substructure of the specified target molecular graph.
Definition of the class CDPL::Chem::AtomBondMapping.
boost::indirect_iterator< ABMappingList::const_iterator, const AtomBondMapping > ConstMappingIterator
A constant random access iterator used to iterate over the stored atom/bond mapping objects.
Definition: SubstructureSearch.hpp:83
bool uniqueMappingsOnly() const
Tells whether duplicate atom/bond mappings are discarded.
A generic boolean expression interface for the implementation of query/target object equivalence test...
Definition: MatchExpression.hpp:75
Definition of the class CDPL::Chem::MatchExpression.
void addBondMappingConstraint(std::size_t query_bond_idx, std::size_t target_bond_idx)
Adds a constraint on the allowed mappings between query and target structure bonds.
The namespace of the Chemical Data Processing Library.
MappingIterator getMappingsBegin()
Returns a mutable iterator pointing to the beginning of the stored atom/bond mapping objects.
MappingIterator getMappingsEnd()
Returns a mutable iterator pointing to the end of the stored atom/bond mapping objects.
void clearBondMappingConstraints()
Clears all previously defined query to target bond mapping constraints.
AtomBondMapping & getMapping(std::size_t idx)
Returns a non-const reference to the stored atom/bond mapping object at index idx.
ConstMappingIterator getMappingsEnd() const
Returns a constant iterator pointing to the end of the stored atom/bond mapping objects.
void setMaxNumMappings(std::size_t max_num_mappings)
Allows to specify a limit on the number of stored atom/bond mappings.
A data structure for the common storage of related atom to atom and bond to bond mappings.
Definition: AtomBondMapping.hpp:55
boost::indirect_iterator< ABMappingList::iterator, AtomBondMapping > MappingIterator
A mutable random access iterator used to iterate over the stored atom/bond mapping objects.
Definition: SubstructureSearch.hpp:78
std::shared_ptr< SubstructureSearch > SharedPointer
Definition: SubstructureSearch.hpp:73
bool operator>(const Array< ValueType > &array1, const Array< ValueType > &array2)
Greater than comparison operator.
std::function< const AtomMatchExprPtr &(const Atom &)> AtomMatchExpressionFunction
Definition: SubstructureSearch.hpp:85
std::function< const BondMatchExprPtr &(const Bond &)> BondMatchExpressionFunction
Definition: SubstructureSearch.hpp:86
void setAtomMatchExpressionFunction(const AtomMatchExpressionFunction &func)
std::size_t getNumMappings() const
Returns the number of atom/bond mappings that were recorded in the last call to findMappings().