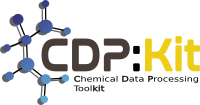 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_VIS_RECTANGLE2D_HPP
30 #define CDPL_VIS_RECTANGLE2D_HPP
77 Rectangle2D(
double min_x,
double min_y,
double max_x,
double max_y);
210 void setBounds(
double min_x,
double min_y,
double max_x,
double max_y);
313 #endif // CDPL_VIS_RECTANGLE2D_HPP
void reset()
Resets the rectangle to an undefinded state.
void addPoint(const Math::Vector2D &pt)
Adds the point pt to the current rectangle.
void scale(double factor)
Scales the minimum and maximum point of the rectangle by the specified factor.
virtual ~Rectangle2D()
Virtual destructor.
Definition: Rectangle2D.hpp:82
const Math::Vector2D & getMin() const
Returns the minimum point of the rectangle.
void setBounds(const Math::Vector2D &min, const Math::Vector2D &max)
Sets the minimum point of the rectangle to min and the maximum point to max.
bool intersectsRectangle(const Rectangle2D &rect) const
Tests if the interior of this rectangle intersects the rectangle rect.
bool containsPoint(double x, double y) const
Tests if the point (x, y) is within the boundary of the rectangle.
void setMin(const Math::Vector2D &pt)
Sets the minimum point of the rectangle to pt.
double getArea() const
Calculates the area of the rectangle.
Rectangle2D(const Math::Vector2D &min, const Math::Vector2D &max)
Constructs a rectangle with the minimum point set to min and the maximum point set to max.
bool containsPoint(const Math::Vector2D &pt) const
Tests if the point pt is within the boundary of the rectangle.
bool operator!=(const Rectangle2D &rect) const
Inequality comparison operator.
void addRectangle(const Rectangle2D &rect)
Adds the rectangle rect to the current rectangle.
const Math::Vector2D & getMax() const
Returns the maximum point of the rectangle.
#define CDPL_VIS_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
bool isDefined() const
Tells if the rectangle is well defined.
QuaternionVectorAdapter< E > vec(QuaternionExpression< E > &e)
Definition: QuaternionAdapter.hpp:237
double getWidth() const
Returns the width of the rectangle.
void getCenter(Math::Vector2D &ctr) const
Calculates the center of the rectangle and stores the result in ctr.
void translate(const Math::Vector2D &vec)
Translates the minimum and maximum point of the rectangle by vec.
The namespace of the Chemical Data Processing Library.
void setMax(double x, double y)
Sets the maximum point of the rectangle to (x, y).
Specifies an axis aligned rectangular area in 2D space.
Definition: Rectangle2D.hpp:51
Rectangle2D()
Constructs an undefined rectangle.
CVector< double, 2 > Vector2D
A bounded 2 element vector holding floating point values of type double.
Definition: Vector.hpp:1632
void addPoint(double x, double y)
Adds the point (x, y) to the current rectangle.
double getHeight() const
Returns the height of the rectangle.
void setMin(double x, double y)
Sets the minimum point of the rectangle to (x, y).
void addMargin(double width, double height)
Extends the rectangle by adding a margin with the specified width and height.
Math::Vector2D getCenter() const
Calculates the center of the rectangle.
bool operator==(const Rectangle2D &rect) const
Equality comparison operator.
Definition of the preprocessor macro CDPL_VIS_API.
Rectangle2D(double min_x, double min_y, double max_x, double max_y)
Constructs a rectangle with the minimum point set to (min_x, min_y) and the maximum point set to (max...
bool containsRectangle(const Rectangle2D &rect) const
Tests if the specified rectangle rect is within the boundary of this rectangle.
void setBounds(double min_x, double min_y, double max_x, double max_y)
Sets the minimum point of the rectangle to (min_x, min_y) and the maximum point to (max_x,...
void setMax(const Math::Vector2D &pt)
Sets the maximum point of the rectangle to pt.
Definition of vector data types.