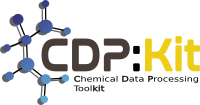 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_REACTOR_HPP
30 #define CDPL_CHEM_REACTOR_HPP
212 void editAtoms()
const;
213 void editBonds()
const;
215 void editStereoProperties()
const;
217 void editProdAtomStereoDescriptor(
const Atom*,
Atom*)
const;
218 void editProdBondStereoDescriptor(
const Bond*,
Bond*)
const;
220 template <
typename T>
228 Atom* getMappedTgtProdAtom(
const Atom*)
const;
229 Bond* getMappedTgtProdBond(
const Bond*)
const;
231 typedef std::pair<std::size_t, std::size_t> IDPair;
233 struct IDPairLessCmpFunc
236 bool operator()(
const IDPair&,
const IDPair&)
const;
239 typedef std::pair<const Atom*, const Atom*> AtomPair;
240 typedef std::pair<const Bond*, const Bond*> BondPair;
241 typedef std::map<std::size_t, const Atom*> IDAtomMap;
242 typedef std::map<IDPair, const Bond*, IDPairLessCmpFunc> IDPairBondMap;
243 typedef std::map<const Atom*, Atom*> AtomMap;
244 typedef std::map<const Bond*, Bond*> BondMap;
245 typedef std::vector<AtomPair> AtomPairList;
246 typedef std::vector<BondPair> BondPairList;
251 IDAtomMap reacAtomsToDelete;
252 IDAtomMap prodAtomsToCreate;
253 IDPairBondMap reacBondsToDelete;
254 IDPairBondMap prodBondsToCreate;
255 AtomPairList ptnAtomMapping;
256 BondPairList ptnBondMapping;
257 AtomMap tgtAtomMapping;
258 BondMap tgtBondMapping;
264 #endif // CDPL_CHEM_REACTOR_HPP
AtomBondMapping ReactionSite
Stores information about perceived reaction-sites.
Definition: Reactor.hpp:59
ReactionSubstructureSearch::MappingIterator ReactionSiteIterator
A mutable random access iterator used to iterate over the perceived reaction-sites.
Definition: Reactor.hpp:64
Definition of the preprocessor macro CDPL_CHEM_API.
boost::indirect_iterator< ABMappingList::iterator, AtomBondMapping > MappingIterator
A mutable random access iterator used to iterate over the stored atom/bond mapping objects.
Definition: ReactionSubstructureSearch.hpp:70
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Bond.
Definition: Bond.hpp:50
ConstReactionSiteIterator getReactionSitesEnd() const
Returns a constant iterator pointing to the end of the stored reaction-site data objects.
std::size_t getNumReactionSites() const
Returns the number of recorded reactions-sites in the last call to findReactionSites().
ReactionSiteIterator begin()
Returns a mutable iterator pointing to the beginning of the stored reaction-site data objects.
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
Definition of the class CDPL::Chem::ReactionSubstructureSearch.
Atom.
Definition: Atom.hpp:52
boost::indirect_iterator< ABMappingList::const_iterator, const AtomBondMapping > ConstMappingIterator
A constant random access iterator used to iterate over the stored atom/bond mapping objects.
Definition: ReactionSubstructureSearch.hpp:75
ReactionSiteIterator getReactionSitesBegin()
Returns a mutable iterator pointing to the beginning of the stored reaction-site data objects.
Reactor()
Constructs and initializes a Reactor instance.
An unique lookup key for control-parameter and property values.
Definition: LookupKey.hpp:54
ConstReactionSiteIterator begin() const
Returns a constant iterator pointing to the beginning of the stored reaction-site data objects.
void setReactionPattern(const Reaction &rxn_pattern)
Allows to specify a new reaction pattern for the transformation of reactants to products.
ReactionSite & getReactionSite(std::size_t idx)
Returns a non-const reference to the stored reaction-site data object at index idx.
ReactionSubstructureSearch.
Definition: ReactionSubstructureSearch.hpp:62
Definition of the type CDPL::Util::BitSet.
Molecule.
Definition: Molecule.hpp:49
A safe, type checked container for arbitrary data of variable type.
Definition: Any.hpp:59
Reactor(const Reaction &rxn_pattern)
Constructs and initializes a Reactor instance for the specified reaction pattern.
const ReactionSite & getReactionSite(std::size_t idx) const
Returns a const reference to the stored reaction-site data object at index idx.
ConstReactionSiteIterator end() const
Returns a constant iterator pointing to the end of the stored reaction-site data objects.
Definition of the class CDPL::Chem::Reaction.
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
ReactionSiteIterator getReactionSitesEnd()
Returns a mutable iterator pointing to the end of the stored reaction-site data objects.
Reactor.
Definition: Reactor.hpp:53
The namespace of the Chemical Data Processing Library.
Reaction.
Definition: Reaction.hpp:52
bool findReactionSites(Reaction &rxn_target)
Perceives all possible reaction-sites on the reactants of the given reaction target where the specifi...
ConstReactionSiteIterator getReactionSitesBegin() const
Returns a constant iterator pointing to the beginning of the stored reaction-site data objects.
A data structure for the common storage of related atom to atom and bond to bond mappings.
Definition: AtomBondMapping.hpp:55
void performReaction(const ReactionSite &rxn_site)
Performs a transformation of the target reactants to corresponding products at the specified reaction...
ReactionSubstructureSearch::ConstMappingIterator ConstReactionSiteIterator
A constant random access iterator used to iterate over the perceived reaction-sites.
Definition: Reactor.hpp:69
ReactionSiteIterator end()
Returns a mutable iterator pointing to the end of the stored reaction-site data objects.