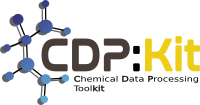 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_VIS_REACTIONVIEW2D_HPP
30 #define CDPL_VIS_REACTIONVIEW2D_HPP
62 class ReactionView2DParameters;
646 void renderGraphicsPrimitives(
Renderer2D&)
const;
650 void initComponentViews();
651 void initComponentBoundsTable();
652 void layoutReaction();
653 void calcOutputTransforms();
654 void initComponentViewViewports();
655 void createPlusSignPrimitives();
656 void createArrowPrimitives();
658 void createStdReactionArrow(
bool);
660 void layoutComponents(
unsigned int, std::size_t, std::size_t);
661 void createPackedComponentLayout(
unsigned int, std::size_t, std::size_t);
662 void createLinearComponentLayout(
unsigned int, std::size_t, std::size_t);
663 void createHLinearComponentLayout(
unsigned int, std::size_t, std::size_t);
664 void createVLinearComponentLayout(
unsigned int, std::size_t, std::size_t);
669 void shiftComponentPositions(std::size_t, std::size_t,
const Math::Vector2D&);
670 void shiftPlusSignPositions(std::size_t, std::size_t,
const Math::Vector2D&);
672 void calcArrowDimensions();
673 void calcPlusSignDimensions();
675 void freeComponentViews();
676 void freeGraphicsPrimitives();
678 void initArrowStyle();
679 void initArrowLength();
687 void initComponentLayout();
688 void initComponentLayoutDirection();
689 void initComponentMargin();
690 void initComponentVisibility();
691 void initAgentAlignment();
692 void initAgentLayout();
693 void initAgentLayoutDirection();
699 void initPlusSignVisibility();
705 typedef std::vector<StructureView2D*> ComponentViewList;
706 typedef std::vector<const GraphicsPrimitive2D*> GraphicsPrimitiveList;
707 typedef std::map<const Chem::MolecularGraph*, Rectangle2D> ComponentBoundsMap;
712 typedef std::unique_ptr<ReactionView2DParameters> ReactionView2DParametersPtr;
715 ReactionView2DParametersPtr parameters;
717 ComponentViewList componentViews;
718 GraphicsPrimitiveList drawList;
721 ComponentBoundsMap componentBounds;
722 Vector2DArray componentPositions;
723 Vector2DArray plusSignPositions;
725 double unscaledArrowLength;
726 double unscaledArrowHeadLength;
727 double unscaledArrowHeadWidth;
728 double unscaledArrowShaftWidth;
729 double unscaledArrowLineWidth;
730 double maxUnscaledArrowWidth;
731 double unscaledPlusSignSize;
732 double unscaledPlusSignLineWidth;
733 double outputScalingFactor;
735 unsigned int arrowStyle;
737 unsigned int componentLayout;
738 unsigned int componentLayoutDirection;
740 unsigned int agentAlignment;
741 unsigned int agentLayout;
742 unsigned int agentLayoutDirection;
746 bool plusSignsVisible;
747 bool reactionChanged;
748 bool fontMetricsChanged;
756 #endif // CDPL_VIS_REACTIONVIEW2D_HPP
Specifies the value and type of a size attribute and defines how the value may change during processi...
Definition: SizeSpecification.hpp:45
An interface that provides methods for low level 2D drawing operations.
Definition: Renderer2D.hpp:86
Definition of the class CDPL::Util::ObjectStack.
void setFontMetrics(FontMetrics *font_metrics)
Specifies a font metrics object that will be used to measure the dimension of text labels.
void getModelBounds(Rectangle2D &bounds)
Calculates the bounding rectangle of the visualized model.
CDPL_VIS_API const SizeSpecification & getArrowLineWidth(const Chem::Reaction &rxn)
Definition of the class CDPL::Vis::SizeSpecification.
void render(Renderer2D &renderer)
Renders the visual representation of the model using the specified Vis::Renderer2D instance.
The abstract base of classes implementing the 2D visualization of data objects.
Definition: View2D.hpp:59
Implements the 2D visualization of chemical reactions.
Definition: ReactionView2D.hpp:590
CDPL_VIS_API const SizeSpecification & getArrowShaftWidth(const Chem::Reaction &rxn)
Definition of the class CDPL::Vis::LineSegmentListPrimitive2D.
void setReaction(const Chem::Reaction *rxn)
Specifies the chemical reaction to visualize.
CDPL_VIS_API const SizeSpecification & getArrowHeadWidth(const Chem::Reaction &rxn)
~ReactionView2D()
Destructor.
CDPL_VIS_API unsigned int getComponentLayout(const Chem::Reaction &rxn)
A graphics primitive representing a list of disjoint line segments.
Definition: LineSegmentListPrimitive2D.hpp:51
Definition of the class CDPL::Vis::PolygonPrimitive2D.
CDPL_VIS_API const Color & getPlusSignColor(const Chem::Reaction &rxn)
FontMetrics * getFontMetrics() const
Returns a pointer to the used font metrics object.
Implements the 2D visualization of chemical structures.
Definition: StructureView2D.hpp:493
CDPL_VIS_API const SizeSpecification & getPlusSignSize(const Chem::Reaction &rxn)
Molecule.
Definition: Molecule.hpp:49
CDPL_VIS_API unsigned int getComponentLayoutDirection(const Chem::Reaction &rxn)
#define CDPL_VIS_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Definition of the class CDPL::Vis::View2D.
CDPL_VIS_API const Color & getArrowColor(const Chem::Reaction &rxn)
A graphics primitive representing a polygon.
Definition: PolygonPrimitive2D.hpp:52
The namespace of the Chemical Data Processing Library.
Reaction.
Definition: Reaction.hpp:52
Specifies an axis aligned rectangular area in 2D space.
Definition: Rectangle2D.hpp:51
CVector< double, 2 > Vector2D
A bounded 2 element vector holding floating point values of type double.
Definition: Vector.hpp:1632
Definition of the class CDPL::Vis::Rectangle2D.
CDPL_VIS_API const SizeSpecification & getArrowHeadLength(const Chem::Reaction &rxn)
Definition of the class CDPL::Vis::StructureView2D.
An interface class with methods that provide information about the metrics of a font.
Definition: FontMetrics.hpp:71
VectorArray< Vector2D > Vector2DArray
An array of Math::Vector2D objects.
Definition: VectorArray.hpp:79
Definition of the class CDPL::Vis::Color.
Definition of the preprocessor macro CDPL_VIS_API.
CDPL_VIS_API const SizeSpecification & getPlusSignLineWidth(const Chem::Reaction &rxn)
ReactionView2D(const Chem::Reaction *rxn=0)
Constructs and initializes a ReactionView2D instance for the visualization of the chemical reaction s...
std::shared_ptr< ReactionView2D > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated ReactionView2D instances.
Definition: ReactionView2D.hpp:596
Specifies a color in terms of its red, green and blue components and an alpha-channel for transparenc...
Definition: Color.hpp:52
Definition of vector data types.
const Chem::Reaction * getReaction() const
Returns a pointer to the visualized chemical reaction.