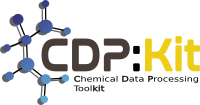 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_FORCEFIELD_MMFF94TORSIONPARAMETERTABLE_HPP
30 #define CDPL_FORCEFIELD_MMFF94TORSIONPARAMETERTABLE_HPP
35 #include <unordered_map>
39 #include <boost/iterator/transform_iterator.hpp>
57 typedef std::unordered_map<std::uint64_t, Entry> DataStorage;
68 Entry(
unsigned int tor_type_idx,
unsigned int term_atom1_type,
unsigned int ctr_atom1_type,
unsigned int ctr_atom2_type,
69 unsigned int term_atom2_type,
double tor_param1,
double tor_param2,
double tor_param3);
90 unsigned int torTypeIdx;
91 unsigned int termAtom1Type;
92 unsigned int ctrAtom1Type;
93 unsigned int ctrAtom2Type;
94 unsigned int termAtom2Type;
101 typedef boost::transform_iterator<std::function<
const Entry&(
const DataStorage::value_type&)>,
102 DataStorage::const_iterator>
105 typedef boost::transform_iterator<std::function<
Entry&(DataStorage::value_type&)>,
106 DataStorage::iterator>
111 void addEntry(
unsigned int tor_type_idx,
unsigned int term_atom1_type,
unsigned int ctr_atom1_type,
unsigned int ctr_atom2_type,
112 unsigned int term_atom2_type,
double tor_param1,
double tor_param2,
double tor_param3);
114 const Entry&
getEntry(
unsigned int tor_type_idx,
unsigned int term_atom1_type,
unsigned int ctr_atom1_type,
unsigned int ctr_atom2_type,
115 unsigned int term_atom2_type)
const;
121 bool removeEntry(
unsigned int tor_type_idx,
unsigned int term_atom1_type,
unsigned int ctr_atom1_type,
unsigned int ctr_atom2_type,
122 unsigned int term_atom2_type);
159 #endif // CDPL_FORCEFIELD_MMFF94TORSIONPARAMETERTABLE_HPP
std::size_t getNumEntries() const
Definition of the preprocessor macro CDPL_FORCEFIELD_API.
unsigned int getTorsionTypeIndex() const
static const SharedPointer & get(unsigned int param_set)
unsigned int getTerminalAtom1Type() const
ConstEntryIterator getEntriesEnd() const
void addEntry(unsigned int tor_type_idx, unsigned int term_atom1_type, unsigned int ctr_atom1_type, unsigned int ctr_atom2_type, unsigned int term_atom2_type, double tor_param1, double tor_param2, double tor_param3)
unsigned int getTerminalAtom2Type() const
Definition: MMFF94TorsionParameterTable.hpp:51
ConstEntryIterator getEntriesBegin() const
ConstEntryIterator end() const
Entry(unsigned int tor_type_idx, unsigned int term_atom1_type, unsigned int ctr_atom1_type, unsigned int ctr_atom2_type, unsigned int term_atom2_type, double tor_param1, double tor_param2, double tor_param3)
MMFF94TorsionParameterTable()
bool removeEntry(unsigned int tor_type_idx, unsigned int term_atom1_type, unsigned int ctr_atom1_type, unsigned int ctr_atom2_type, unsigned int term_atom2_type)
ConstEntryIterator begin() const
unsigned int getCenterAtom1Type() const
unsigned int getCenterAtom2Type() const
Definition: MMFF94TorsionParameterTable.hpp:63
const Entry & getEntry(unsigned int tor_type_idx, unsigned int term_atom1_type, unsigned int ctr_atom1_type, unsigned int ctr_atom2_type, unsigned int term_atom2_type) const
void loadDefaults(unsigned int param_set)
static void set(const SharedPointer &table, unsigned int param_set)
The namespace of the Chemical Data Processing Library.
double getTorsionParameter2() const
EntryIterator getEntriesBegin()
#define CDPL_FORCEFIELD_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
EntryIterator getEntriesEnd()
void load(std::istream &is)
std::shared_ptr< MMFF94TorsionParameterTable > SharedPointer
Definition: MMFF94TorsionParameterTable.hpp:60
double getTorsionParameter1() const
boost::transform_iterator< std::function< const Entry &(const DataStorage::value_type &)>, DataStorage::const_iterator > ConstEntryIterator
Definition: MMFF94TorsionParameterTable.hpp:103
EntryIterator removeEntry(const EntryIterator &it)
boost::transform_iterator< std::function< Entry &(DataStorage::value_type &)>, DataStorage::iterator > EntryIterator
Definition: MMFF94TorsionParameterTable.hpp:107
double getTorsionParameter3() const