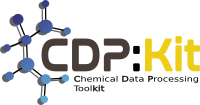 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_FORCEFIELD_MMFF94PARTIALBONDCHARGEINCREMENTTABLE_HPP
30 #define CDPL_FORCEFIELD_MMFF94PARTIALBONDCHARGEINCREMENTTABLE_HPP
34 #include <unordered_map>
38 #include <boost/iterator/transform_iterator.hpp>
56 typedef std::unordered_map<unsigned int, Entry> DataStorage;
59 typedef std::shared_ptr<MMFF94PartialBondChargeIncrementTable>
SharedPointer;
67 Entry(
unsigned int atom_type,
double part_bond_chg_inc,
double form_chg_adj_factor);
78 unsigned int atomType;
79 double partChargeIncr;
80 double formChargeAdjFactor;
84 typedef boost::transform_iterator<std::function<
const Entry&(
const DataStorage::value_type&)>,
85 DataStorage::const_iterator>
88 typedef boost::transform_iterator<std::function<
Entry&(DataStorage::value_type&)>,
89 DataStorage::iterator>
94 void addEntry(
unsigned int atom_type,
double part_bond_chg_inc,
double form_chg_adj_factor);
137 #endif // CDPL_FORCEFIELD_MMFF94PARTIALBONDCHARGEINCREMENTTABLE_HPP
Definition of the preprocessor macro CDPL_FORCEFIELD_API.
static void set(const SharedPointer &table)
ConstEntryIterator getEntriesBegin() const
const Entry & getEntry(unsigned int atom_type) const
double getPartialChargeIncrement() const
ConstEntryIterator end() const
EntryIterator getEntriesBegin()
void addEntry(unsigned int atom_type, double part_bond_chg_inc, double form_chg_adj_factor)
Definition: MMFF94PartialBondChargeIncrementTable.hpp:62
Entry(unsigned int atom_type, double part_bond_chg_inc, double form_chg_adj_factor)
EntryIterator removeEntry(const EntryIterator &it)
bool removeEntry(unsigned int atom_type)
boost::transform_iterator< std::function< const Entry &(const DataStorage::value_type &)>, DataStorage::const_iterator > ConstEntryIterator
Definition: MMFF94PartialBondChargeIncrementTable.hpp:86
unsigned int getAtomType() const
ConstEntryIterator begin() const
std::shared_ptr< MMFF94PartialBondChargeIncrementTable > SharedPointer
Definition: MMFF94PartialBondChargeIncrementTable.hpp:59
double getFormalChargeAdjustmentFactor() const
The namespace of the Chemical Data Processing Library.
std::size_t getNumEntries() const
ConstEntryIterator getEntriesEnd() const
#define CDPL_FORCEFIELD_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
EntryIterator getEntriesEnd()
Definition: MMFF94PartialBondChargeIncrementTable.hpp:50
static const SharedPointer & get()
void load(std::istream &is)
boost::transform_iterator< std::function< Entry &(DataStorage::value_type &)>, DataStorage::iterator > EntryIterator
Definition: MMFF94PartialBondChargeIncrementTable.hpp:90
MMFF94PartialBondChargeIncrementTable()