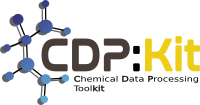 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_FORCEFIELD_MMFF94BONDSTRETCHINGPARAMETERTABLE_HPP
30 #define CDPL_FORCEFIELD_MMFF94BONDSTRETCHINGPARAMETERTABLE_HPP
35 #include <unordered_map>
39 #include <boost/iterator/transform_iterator.hpp>
57 typedef std::unordered_map<std::uint32_t, Entry> DataStorage;
60 typedef std::shared_ptr<MMFF94BondStretchingParameterTable>
SharedPointer;
68 Entry(
unsigned int bond_type_idx,
unsigned int atom1_type,
unsigned int atom2_type,
69 double force_const,
double ref_length);
84 unsigned int bondTypeIdx;
85 unsigned int atom1Type;
86 unsigned int atom2Type;
92 typedef boost::transform_iterator<std::function<
const Entry&(
const DataStorage::value_type&)>,
93 DataStorage::const_iterator>
96 typedef boost::transform_iterator<std::function<
Entry&(DataStorage::value_type&)>,
97 DataStorage::iterator>
102 void addEntry(
unsigned int bond_type_idx,
unsigned int atom1_type,
unsigned int atom2_type,
103 double force_const,
double ref_length);
105 const Entry&
getEntry(
unsigned int bond_type_idx,
unsigned int atom1_type,
unsigned int atom2_type)
const;
111 bool removeEntry(
unsigned int bond_type_idx,
unsigned int atom1_type,
unsigned int atom2_type);
146 #endif // CDPL_FORCEFIELD_MMFF94BONDSTRETCHINGPARAMETERTABLE_HPP
boost::transform_iterator< std::function< Entry &(DataStorage::value_type &)>, DataStorage::iterator > EntryIterator
Definition: MMFF94BondStretchingParameterTable.hpp:98
Definition of the preprocessor macro CDPL_FORCEFIELD_API.
static const SharedPointer & get()
static void set(const SharedPointer &table)
ConstEntryIterator end() const
EntryIterator getEntriesBegin()
Definition: MMFF94BondStretchingParameterTable.hpp:63
ConstEntryIterator getEntriesBegin() const
EntryIterator removeEntry(const EntryIterator &it)
std::shared_ptr< MMFF94BondStretchingParameterTable > SharedPointer
Definition: MMFF94BondStretchingParameterTable.hpp:60
double getReferenceLength() const
Definition: MMFF94BondStretchingParameterTable.hpp:51
std::size_t getNumEntries() const
unsigned int getAtom2Type() const
ConstEntryIterator begin() const
bool removeEntry(unsigned int bond_type_idx, unsigned int atom1_type, unsigned int atom2_type)
The namespace of the Chemical Data Processing Library.
boost::transform_iterator< std::function< const Entry &(const DataStorage::value_type &)>, DataStorage::const_iterator > ConstEntryIterator
Definition: MMFF94BondStretchingParameterTable.hpp:94
void addEntry(unsigned int bond_type_idx, unsigned int atom1_type, unsigned int atom2_type, double force_const, double ref_length)
ConstEntryIterator getEntriesEnd() const
void load(std::istream &is)
EntryIterator getEntriesEnd()
#define CDPL_FORCEFIELD_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
unsigned int getBondTypeIndex() const
MMFF94BondStretchingParameterTable()
const Entry & getEntry(unsigned int bond_type_idx, unsigned int atom1_type, unsigned int atom2_type) const
unsigned int getAtom1Type() const
Entry(unsigned int bond_type_idx, unsigned int atom1_type, unsigned int atom2_type, double force_const, double ref_length)
double getForceConstant() const