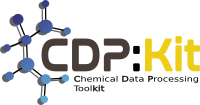 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_FRAGMENTGENERATOR_HPP
30 #define CDPL_CHEM_FRAGMENTGENERATOR_HPP
111 unsigned int rule_id,
unsigned int atom1_label,
unsigned int atom2_label);
126 std::size_t frag1Idx;
127 std::size_t frag2Idx;
130 unsigned int atom1Label;
131 unsigned int atom2Label;
135 typedef std::vector<FragmentationRule> FragmentationRuleList;
136 typedef std::vector<ExcludePattern> ExcludePatternList;
137 typedef std::vector<FragmentLink> FragmentLinkList;
232 std::size_t start_idx)
const;
239 unsigned int atom1Label;
240 unsigned int atom2Label;
243 typedef std::vector<SplitBondData> SplitBondDataArray;
245 FragmentationRuleList fragRules;
246 ExcludePatternList exclPatterns;
247 FragmentLinkList fragLinks;
249 FragmentFilterFunction fragFilterFunc;
253 SplitBondDataArray splitBondData;
258 #endif // CDPL_CHEM_FRAGMENTGENERATOR_HPP
std::shared_ptr< MolecularGraph > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated MolecularGraph instances.
Definition: MolecularGraph.hpp:58
std::size_t getNumFragmentationRules() const
void removeFragmentationRule(std::size_t idx)
Definition of the preprocessor macro CDPL_CHEM_API.
void setMatchPattern(const MolecularGraph::SharedPointer &ptn)
void addFragmentationRule(const MolecularGraph::SharedPointer &match_ptn, unsigned int rule_id)
void addExcludePattern(const ExcludePattern &excl_ptn)
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Definition: FragmentGenerator.hpp:62
ConstFragmentationRuleIterator getFragmentationRulesEnd() const
ConstFragmentationRuleIterator getFragmentationRulesBegin() const
Bond.
Definition: Bond.hpp:50
FragmentationRuleList::const_iterator ConstFragmentationRuleIterator
Definition: FragmentGenerator.hpp:140
std::size_t getNumFragmentLinks() const
void clearFragmentationRules()
void addExcludePattern(const MolecularGraph::SharedPointer &match_ptn, unsigned int rule_id)
void setGeneric(bool generic)
const MolecularGraph::SharedPointer & getMatchPattern() const
SubstructureSearch.
Definition: SubstructureSearch.hpp:64
unsigned int getAtom1Label() const
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
void removeExcludePattern(std::size_t idx)
Atom.
Definition: Atom.hpp:52
Fragment.
Definition: Fragment.hpp:52
const ExcludePattern & getExcludePattern(std::size_t idx) const
std::size_t getNumExcludePatterns() const
FragmentGenerator(const FragmentGenerator &gen)
FragmentGenerator & operator=(const FragmentGenerator &gen)
MolecularGraph.
Definition: MolecularGraph.hpp:52
Definition of the type CDPL::Util::BitSet.
Definition: FragmentGenerator.hpp:81
ExcludePattern(const MolecularGraph::SharedPointer &match_ptn, unsigned int rule_id)
void setMatchPattern(const MolecularGraph::SharedPointer &ptn)
Definition: FragmentGenerator.hpp:107
std::shared_ptr< FragmentGenerator > SharedPointer
Definition: FragmentGenerator.hpp:57
void addExcludePattern(const MolecularGraph::SharedPointer &match_ptn)
std::size_t getFragment2Index() const
ConstFragmentLinkIterator getFragmentLinksEnd() const
FragmentationRuleIterator getFragmentationRulesBegin()
ConstExcludePatternIterator getExcludePatternsBegin() const
unsigned int getRuleID() const
Definition of the class CDPL::Chem::MolecularGraph.
unsigned int getAtom2Label() const
FragmentGenerator()
Constructs the FragmentGenerator instance.
bool splitBondsIncluded() const
void setFragmentFilterFunction(const FragmentFilterFunction &func)
FragmentGenerator.
Definition: FragmentGenerator.hpp:54
const FragmentLink & getFragmentLink(std::size_t idx) const
unsigned int getRuleID() const
A data type for the storage of Chem::Fragment objects.
Definition: FragmentList.hpp:49
ConstFragmentLinkIterator getFragmentLinksBegin() const
void includeSplitBonds(bool include)
CDPL_CHEM_API void splitIntoFragments(const MolecularGraph &molgraph, FragmentList &frag_list, const Util::BitSet &split_bond_mask, bool append=false)
ExcludePatternList::const_iterator ConstExcludePatternIterator
Definition: FragmentGenerator.hpp:143
FragmentationRuleIterator getFragmentationRulesEnd()
The namespace of the Chemical Data Processing Library.
ExcludePatternIterator getExcludePatternsEnd()
const MolecularGraph::SharedPointer & getMatchPattern() const
const Bond & getBond() const
ExcludePattern(const MolecularGraph::SharedPointer &match_ptn)
std::function< bool(const MolecularGraph &)> FragmentFilterFunction
Definition: FragmentGenerator.hpp:59
void setRuleID(unsigned int id)
ExcludePattern & getExcludePattern(std::size_t idx)
FragmentationRule & getFragmentationRule(std::size_t idx)
void addFragmentationRule(const FragmentationRule &rule)
ConstExcludePatternIterator getExcludePatternsEnd() const
const FragmentFilterFunction & getFragmentFilterFunction() const
unsigned int getID() const
Definition of the class CDPL::Chem::SubstructureSearch.
FragmentationRuleList::iterator FragmentationRuleIterator
Definition: FragmentGenerator.hpp:141
virtual ~FragmentGenerator()
Definition: FragmentGenerator.hpp:155
ExcludePatternIterator getExcludePatternsBegin()
FragmentLinkList::const_iterator ConstFragmentLinkIterator
Definition: FragmentGenerator.hpp:146
Definition of the class CDPL::Chem::FragmentList.
FragmentLink(std::size_t frag1_idx, std::size_t frag2_idx, const Bond &bond, unsigned int rule_id, unsigned int atom1_label, unsigned int atom2_label)
void clearExcludePatterns()
FragmentationRule(const MolecularGraph::SharedPointer &match_ptn, unsigned int id)
std::size_t getFragment1Index() const
void setID(unsigned int id)
void generate(const MolecularGraph &molgraph, FragmentList &frag_list, bool append=false)
const FragmentationRule & getFragmentationRule(std::size_t idx) const
ExcludePatternList::iterator ExcludePatternIterator
Definition: FragmentGenerator.hpp:144