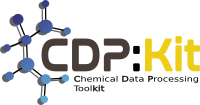 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_SHAPE_FASTGAUSSIANSHAPEALIGNMENT_HPP
30 #define CDPL_SHAPE_FASTGAUSSIANSHAPEALIGNMENT_HPP
35 #include <unordered_map>
39 #include <boost/functional/hash.hpp>
40 #include <boost/random/mersenne_twister.hpp>
62 typedef std::vector<AlignmentResult> ResultList;
65 static constexpr
double DEF_OPTIMIZATION_STOP_GRADIENT = 1.0;
66 static constexpr std::size_t DEF_MAX_OPTIMIZATION_ITERATIONS = 20;
68 static constexpr
double DEF_SYMMETRY_THRESHOLD = 0.15;
69 static constexpr std::size_t DEF_NUM_RANDOM_STARTS = 4;
70 static constexpr
double DEF_MAX_RANDOM_TRANSLATION = 2.0;
211 typedef std::vector<Element> ElementArray;
213 ElementArray elements;
214 std::size_t colElemOffs;
215 std::size_t setIndex;
217 unsigned int symClass;
220 double colSelfOverlap;
221 bool equalNonColDelta;
224 typedef std::pair<std::size_t, std::size_t> ResultID;
230 void alignAndProcessResults(std::size_t ref_idx, std::size_t al_idx);
231 void processResult(
AlignmentResult& res, std::size_t ref_idx, std::size_t al_idx);
233 void setupShapeData(
const GaussianShape& shape, ShapeData& data,
bool ref);
236 void prepareForAlignment();
238 bool generateStartTransforms(
const ShapeData& ref_data);
240 void generateTransformsForElementCenters(
const ShapeData& data,
unsigned int axes_swap_flags,
bool ref_shape);
241 void generateTransforms(
const Math::Vector3D& ctr_trans,
unsigned int axes_swap_flags);
243 template <
typename QE>
246 void transformAlignedShape();
251 double calcOverlap(
const ShapeData& ref_data,
const ShapeData& ovl_data,
bool color)
const;
254 bool getResultIndex(
const ResultID& res_id, std::size_t& res_idx);
256 typedef std::vector<ShapeData> ShapeDataArray;
257 typedef std::unordered_map<ResultID, std::size_t, boost::hash<ResultID> > ResultIndexMap;
258 typedef std::vector<QuaternionTransformation> StartTransformList;
259 typedef boost::random::mt11213b RandomEngine;
265 std::size_t maxNumOptIters;
267 unsigned int resultSelMode;
270 ShapeDataArray refShapeData;
271 ShapeData algdShapeData;
272 ResultIndexMap resIndexMap;
274 std::size_t currSetIndex;
275 std::size_t currShapeIndex;
278 bool nonColCtrStarts;
280 bool genForAlgdShape;
282 bool genForLargerShape;
284 double maxRandomTrans;
285 std::size_t numRandomStarts;
286 std::size_t numSubTransforms;
287 RandomEngine randomEngine;
288 StartTransformList startTransforms;
294 std::size_t currRefShapeIdx;
299 #endif // CDPL_SHAPE_FASTGAUSSIANSHAPEALIGNMENT_HPP
std::size_t getMaxNumOptimizationIterations() const
bool genShapeCenterStarts() const
Definition: GaussianShape.hpp:55
void genShapeCenterStarts(bool generate)
std::size_t getNumReferenceShapes() const
void genForLargerShapeCenters(bool generate)
~FastGaussianShapeAlignment()
ConstResultIterator begin() const
Definition: GaussianShapeSet.hpp:46
Definition of the class CDPL::Math::VectorArray.
FastGaussianShapeAlignment(const GaussianShapeSet &ref_shapes)
void greedyOptimization(bool greedy)
Definition: FastGaussianShapeAlignment.hpp:60
CVector< double, 3 > Vector3D
A bounded 3 element vector holding floating point values of type double.
Definition: Vector.hpp:1637
void performAlignment(bool perf_align)
void optimizeOverlap(bool optimize)
Definition of the class CDPL::Shape::AlignmentResult.
bool genRandomStarts() const
AlignmentResult & getResult(std::size_t idx)
CDPL_SHAPE_API void transform(GaussianShape &shape, const Math::Matrix4D &xform)
ConstResultIterator getResultsEnd() const
const unsigned int BEST_PER_REFERENCE_SET
Definition: AlignmentResultSelectionMode.hpp:45
void genForAlignedShapeCenters(bool generate)
double getSymmetryThreshold()
double getMaxRandomTranslation() const
std::function< bool(const AlignmentResult &, const AlignmentResult &)> ResultCompareFunction
Definition: FastGaussianShapeAlignment.hpp:78
ResultIterator getResultsEnd()
Definition of various quaternion expression types and operations.
Definition of the class CDPL::Shape::GaussianShapeSet.
Implementation of the BFGS optimization algorithm.
unsigned int getResultSelectionMode() const
const AlignmentResult & getResult(std::size_t idx) const
void setMaxRandomTranslation(double max_trans)
Definition: Expression.hpp:98
bool performAlignment() const
void setRandomSeed(unsigned int seed)
Definition of constants in namespace CDPL::Shape::AlignmentResultSelectionMode.
const ResultCompareFunction & getResultCompareFunction() const
double radius
Definition: FastGaussianShapeAlignment.hpp:205
Definition: FastGaussianShapeAlignment.hpp:201
std::shared_ptr< FastGaussianShapeAlignment > SharedPointer
Definition: FastGaussianShapeAlignment.hpp:72
bool greedyOptimization() const
double weightFactor
Definition: FastGaussianShapeAlignment.hpp:207
void setSymmetryThreshold(double thresh)
ConstResultIterator end() const
std::size_t getNumRandomStarts() const
void addReferenceShape(const GaussianShape &shape, bool new_set=true)
bool optimizeOverlap() const
FastGaussianShapeAlignment(const GaussianShape &ref_shape)
Definition: Vector.hpp:1053
A data type for the descripton of arbitrary shapes composed of spheres approximated by gaussian funct...
Definition: GaussianShape.hpp:51
void setOptimizationStopGradient(double grad_norm)
double delta
Definition: FastGaussianShapeAlignment.hpp:206
std::size_t color
Definition: FastGaussianShapeAlignment.hpp:203
bool genForReferenceShapeCenters() const
void setNumRandomStarts(std::size_t num_starts)
std::function< double(const AlignmentResult &)> ScoringFunction
Definition: FastGaussianShapeAlignment.hpp:77
void addReferenceShapes(const GaussianShapeSet &shapes, bool new_set=true)
Math::Vector3D center
Definition: FastGaussianShapeAlignment.hpp:204
void genNonColorCenterStarts(bool generate)
#define CDPL_SHAPE_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
void setScoringFunction(const ScoringFunction &func)
bool align(const GaussianShape &shape)
The namespace of the Chemical Data Processing Library.
bool genForLargerShapeCenters() const
Definition of the preprocessor macro CDPL_SHAPE_API.
bool genNonColorCenterStarts() const
ResultList::iterator ResultIterator
Definition: FastGaussianShapeAlignment.hpp:75
double getOptimizationStopGradient() const
void genRandomStarts(bool generate)
double volume
Definition: FastGaussianShapeAlignment.hpp:208
Definition: AlignmentResult.hpp:45
void genForReferenceShapeCenters(bool generate)
Definition of matrix data types.
void setMaxNumOptimizationIterations(std::size_t max_iter)
void setResultCompareFunction(const ResultCompareFunction &func)
void genColorCenterStarts(bool generate)
bool genColorCenterStarts() const
void clearReferenceShapes()
void setResultSelectionMode(unsigned int mode)
ResultList::const_iterator ConstResultIterator
Definition: FastGaussianShapeAlignment.hpp:74
bool align(const GaussianShapeSet &shapes)
FastGaussianShapeAlignment()
ConstResultIterator getResultsBegin() const
const ScoringFunction & getScoringFunction() const
std::size_t getNumResults() const
ResultIterator getResultsBegin()
bool genForAlignedShapeCenters() const