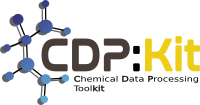 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_ENTITYCONTAINER_HPP
30 #define CDPL_CHEM_ENTITYCONTAINER_HPP
55 class ConstEntityAccessor;
175 ConstEntityAccessor(
const EntityAccessor& accessor):
176 container(accessor.container) {}
181 const Entity3D& operator()(std::size_t idx)
const
183 return container->getEntity(idx);
186 bool operator==(
const ConstEntityAccessor& accessor)
const
188 return (container == accessor.container);
191 ConstEntityAccessor& operator=(
const EntityAccessor& accessor)
193 container = accessor.container;
198 const Entity3DContainer* container;
204 friend class ConstEntityAccessor;
207 EntityAccessor(Entity3DContainer* cntnr):
210 Entity3D& operator()(std::size_t idx)
const
212 return container->getEntity(idx);
215 bool operator==(
const EntityAccessor& accessor)
const
217 return (container == accessor.container);
221 Entity3DContainer* container;
227 #endif // CDPL_CHEM_ENTITYCONTAINER_HPP
ConstEntityIterator getEntitiesBegin() const
Returns a constant iterator pointing to the beginning of the stored const Chem::Entity3D objects.
Util::IndexedElementIterator< Entity3D, EntityAccessor > EntityIterator
A mutable random access iterator used to iterate over the stored Chem::Entity3D objects.
Definition: Entity3DContainer.hpp:67
Definition of the preprocessor macro CDPL_CHEM_API.
Definition of the class CDPL::Util::IndexedElementIterator.
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Entity3DContainer & getEntities()
Returns a reference to itself.
Definition: Entity3DContainer.hpp:152
GridEquality< E1, E2 >::ResultType operator==(const GridExpression< E1 > &e1, const GridExpression< E2 > &e2)
Definition: GridExpression.hpp:339
virtual const Entity3D & getEntity(std::size_t idx) const =0
Returns a const reference to the Chem::Entity3D instance at index idx.
A STL compatible random access iterator for container elements accessible by index.
Definition: IndexedElementIterator.hpp:125
EntityIterator end()
Returns a mutable iterator pointing to the end of the stored Chem::Entity3D objects.
ConstEntityIterator begin() const
Returns a constant iterator pointing to the beginning of the stored const Chem::Entity3D objects.
EntityIterator begin()
Returns a mutable iterator pointing to the beginning of the stored Chem::Entity3D objects.
EntityIterator getEntitiesEnd()
Returns a mutable iterator pointing to the end of the stored Chem::Entity3D objects.
EntityIterator getEntitiesBegin()
Returns a mutable iterator pointing to the beginning of the stored Chem::Entity3D objects.
A common interface for data-structures that support a random access to stored Chem::Entity3D instance...
Definition: Entity3DContainer.hpp:53
Entity3DContainer & operator=(const Entity3DContainer &cntnr)
Assignment operator.
Util::IndexedElementIterator< const Entity3D, ConstEntityAccessor > ConstEntityIterator
A constant random access iterator used to iterate over the stored const Chem::Entity3D objects.
Definition: Entity3DContainer.hpp:56
const Entity3DContainer & getEntities() const
Returns a const reference to itself.
Definition: Entity3DContainer.hpp:143
ConstEntityIterator end() const
Returns a constant iterator pointing to the end of the stored const Chem::Entity3D objects.
The namespace of the Chemical Data Processing Library.
ConstEntityIterator getEntitiesEnd() const
Returns a constant iterator pointing to the end of the stored const Chem::Entity3D objects.
virtual ~Entity3DContainer()
Virtual destructor.
Definition: Entity3DContainer.hpp:161
virtual Entity3D & getEntity(std::size_t idx)=0
Returns a non-const reference to the entity at index idx.
virtual std::size_t getNumEntities() const =0
Returns the number of stored Chem::Entity3D objects.
Entity3D.
Definition: Entity3D.hpp:46