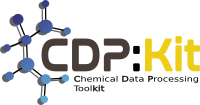 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CONFGEN_DGCONSTRAINTGENERATOR_HPP
30 #define CDPL_CONFGEN_DGCONSTRAINTGENERATOR_HPP
34 #include <unordered_map>
51 class MMFF94InteractionData;
74 typedef std::vector<StereoCenterData> StereoCenterDataArray;
126 std::size_t& num_vol_constr, std::size_t level);
133 void extractAtomStereoCenterData();
134 void extractBondStereoCenterData();
136 void setBondLength(std::size_t atom1_idx, std::size_t atom2_idx,
double length);
137 double getBondLength(std::size_t atom1_idx, std::size_t atom2_idx)
const;
139 void setBondAngle(std::size_t atom1_idx, std::size_t atom2_idx, std::size_t atom3_idx,
double angle);
140 double getBondAngle(std::size_t atom1_idx, std::size_t atom2_idx, std::size_t atom3_idx)
const;
143 std::size_t getSmallestRingSize(
const Chem::FragmentList& sssr, std::size_t atom1_idx, std::size_t atom2_idx)
const;
145 void markAtomPairProcessed(std::size_t atom1_idx, std::size_t atom2_idx);
146 bool atomPairProcessed(std::size_t atom1_idx, std::size_t atom2_idx)
const;
148 double calc13AtomDistance(
double bond1_len,
double bond2_len,
double angle)
const;
150 double calcCis14AtomDistance(
double bond1_len,
double bond2_len,
double bond3_len,
151 double angle_12,
double angle_23)
const;
152 double calcTrans14AtomDistance(
double bond1_len,
double bond2_len,
double bond3_len,
153 double angle_12,
double angle_23)
const;
158 typedef std::vector<std::size_t> AtomIndexList;
160 std::size_t getNeighborAtoms(
const Chem::Atom& atom, AtomIndexList& idx_list,
163 typedef std::pair<std::size_t, std::size_t> BondLengthKey;
164 typedef std::tuple<std::size_t, std::size_t, std::size_t> BondAngleKey;
169 std::size_t operator()(
const BondAngleKey& k)
const;
175 std::size_t operator()(
const BondLengthKey& k)
const;
178 typedef std::unordered_map<BondLengthKey, double, BondLengthKeyHash> BondLengthTable;
179 typedef std::unordered_map<BondAngleKey, double, BondAngleKeyHash> BondAngleTable;
185 BondLengthTable bondLengthTable;
186 BondAngleTable bondAngleTable;
187 StereoCenterDataArray atomStereoData;
188 StereoCenterDataArray bondStereoData;
189 std::size_t numAtoms;
190 AtomIndexList atomIndexList1;
191 AtomIndexList atomIndexList2;
192 DGConstraintGeneratorSettings settings;
197 #endif // CDPL_CONFGEN_DGCONSTRAINTGENERATOR_HPP
Definition: MMFF94InteractionData.hpp:51
Definition of the class CDPL::Math::VectorArray.
void setup(const Chem::MolecularGraph &molgraph, const ForceField::MMFF94InteractionData &ia_data)
Bond.
Definition: Bond.hpp:50
const StereoCenterData & getBondStereoCenterData(std::size_t idx) const
DGCoordinatesGenerator< 3, double > DG3DCoordinatesGenerator
Definition: DGCoordinatesGenerator.hpp:296
std::pair< std::size_t, Chem::StereoDescriptor > StereoCenterData
Definition: DGConstraintGenerator.hpp:71
ConstStereoCenterDataIterator getAtomStereoCenterDataBegin() const
const StereoCenterData & getAtomStereoCenterData(std::size_t idx) const
DGConstraintGeneratorSettings & getSettings()
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
std::size_t getNumBondStereoCenters() const
void add14DistanceConstraints(Util::DG3DCoordinatesGenerator &coords_gen)
Atom.
Definition: Atom.hpp:52
void addBondConfigurationConstraints(Util::DG3DCoordinatesGenerator &coords_gen)
StereoCenterDataArray::const_iterator ConstStereoCenterDataIterator
Definition: DGConstraintGenerator.hpp:77
MolecularGraph.
Definition: MolecularGraph.hpp:52
Definition of the type CDPL::Util::BitSet.
void setup(const Chem::MolecularGraph &molgraph)
A common interface for data-structures that support a random access to stored Chem::Atom instances.
Definition: AtomContainer.hpp:55
const Util::BitSet & getExcludedHydrogenMask() const
void addDefaultDistanceConstraints(Util::DG3DCoordinatesGenerator &coords_gen)
std::size_t getNumAtomStereoCenters() const
Definition of the preprocessor macro CDPL_CONFGEN_API.
#define CDPL_CONFGEN_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
A data type for the storage of Chem::Fragment objects.
Definition: FragmentList.hpp:49
void addAtomPlanarityConstraints(Util::DG3DCoordinatesGenerator &coords_gen)
Definition: DGConstraintGenerator.hpp:68
const DGConstraintGeneratorSettings & getSettings() const
The namespace of the Chemical Data Processing Library.
void addBondStereoCenter(const Chem::Bond &bond, const Chem::StereoDescriptor &descr)
ConstStereoCenterDataIterator getBondStereoCenterDataBegin() const
void addBondPlanarityConstraints(Util::DG3DCoordinatesGenerator &coords_gen)
ConstStereoCenterDataIterator getBondStereoCenterDataEnd() const
Definition of the class CDPL::ConfGen::DGConstraintGeneratorSettings.
A data structure for the storage and retrieval of stereochemical information about atoms and bonds.
Definition: StereoDescriptor.hpp:102
Definition of the type CDPL::Chem::StereoDescriptor.
Implementation of a distance geometry based coordinates generator.
void addAtomConfigurationConstraints(Util::DG3DCoordinatesGenerator &coords_gen)
void addBondAngleConstraints(Util::DG3DCoordinatesGenerator &coords_gen)
void addFixedSubstructureConstraints(const Chem::AtomContainer &atoms, const Math::Vector3DArray &coords, Util::DG3DCoordinatesGenerator &coords_gen)
Definition: DGConstraintGeneratorSettings.hpp:42
void addAtomStereoCenter(const Chem::Atom &atom, const Chem::StereoDescriptor &descr)
ConstStereoCenterDataIterator getAtomStereoCenterDataEnd() const
VectorNorm2< E >::ResultType length(const VectorExpression< E > &e)
Definition: VectorExpression.hpp:553
void addBondLengthConstraints(Util::DG3DCoordinatesGenerator &coords_gen)